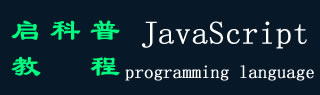
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript String fromCodePoint() 方法
JavaScript String fromCodePoint() 方法返回由指定的码位值序列创建的字符串。可以将一个或多个 Unicode 码位值传递给此方法。如果传递的值不是整数、小于 0(或负数)或大于 0x10FFFF,它将引发 RangeError 异常。此方法是静态的,可以使用类语法(如 String.fromCodePoint())调用,而不是在变量上调用它。
Unicode 码位是与 Unicode 标准中的特定字符相对应的数值。每个字符和符号都有自己唯一的 Unicode 码位值。
语法
以下是 JavaScript String fromCodePoint() 方法的语法 -
String.fromCodePoint(num1, num2, /*..., */ numN)
参数
此方法接受多个相同类型的参数,例如 'num1'、'num2'、'num3' 到 'numN',如下所述 -
- num1, num2....numN − 它是一个整数值,表示 [0, 0x10FFFF] 范围内的 Unicode 码位。
返回值
此方法返回由指定代码点序列创建的字符串。
示例 1
如果我们将单个 Unicode 码位值传递给此方法,它将返回一个只有一个字符的字符串。
在下面的示例中,我们使用 JavaScript String fromCodePoint() 方法来检索由指定的 Uni 码位值 65 创建的字符串。
<html>
<head>
<title>JavaScript 字符串 fromCodePoint() 方法</title>
</head>
<body>
<script>
const unicodepoint = 65;
document.write("统一编码点: ", unicodepoint);
document.write("<br>uni码点 ", unicodepoint, " 代表 ", String.fromCodePoint(unicodepoint));
</script>
</body>
</html>
输出
上面的程序返回一个字符串 “A”。
uni码点 65 代表 A
示例 2
如果传递了多个 Unicode 值,该方法将返回包含多个字符的字符串。
以下是 JavaScript String fromCodePoint() 方法的另一个示例。我们使用此方法检索包含由指定 uni 码序列 81、105、107、101、112、117、67、111、109 创建的多个字符的字符串。
<html>
<head>
<title>JavaScript 字符串 fromCodePoint() 方法</title>
</head>
<body>
<script>
const num1 = 81;
const num2 = 105;
const num3 = 107;
const num4 = 101;
const num5 = 112;
const num6 = 67;
const num7 = 111;
const num8 = 109;
document.write("统一码点值为: ", num1, ", ", num2, ", ", num3, ", ", num4, ", ", num5, ", ", num6, ", ", num7, ", ", num7, ", ", num8);
document.write("<br>已创建字符串: ", String.fromCodePoint(num1, num2, num3, num4, num5, num6, num7, num8));
</script>
</body>
</html>
输出
执行上述程序后,它将返回一个新字符串 “QikepuCom”。
已创建字符串: QikepCom
示例 3
如果 numN 不是整数、小于零或大于 0x10FFFF,它将引发 'RangeError' 异常。
<html>
<head>
<title>JavaScript 字符串 fromCodePoint() 方法</title>
</head>
<body>
<script>
const unicodepoint = -2;
document.write("统一编码点: ", unicodepoint);
try {
document.write("已创建字符串: ", String.fromCodePoint(unicodepoint));
} catch (error) {
document.write("<br>", error);
}
</script>
</body>
</html>
输出
执行上述程序后,它将引发 'RangeError' 异常。
RangeError: Invalid code point -2
示例 4
在下面给定的示例中,我们在 from 循环中使用 fromCodePoint() 方法检索一个字符串,其中包含由传递给该方法的数字一一创建的所有大写字母,循环从 65 开始迭代到 90。
<html>
<head>
<title>JavaScript 字符串 fromCodePoint() 方法</title>
</head>
<body>
<script>
const unicodepoint_start = 65;
document.write("Unicode起始于: ", unicodepoint_start);
document.write("<br>新建字符串: ");
for(let i = unicodepoint_start; i<=90; i++){
document.write(String.fromCodePoint(i));
}
</script>
</body>
</html>
输出
上面的程序返回一个包含所有大写字母的字符串 -
新建字符串: ABCDEFGHIJKLMNOPQRSTUVWXYZ