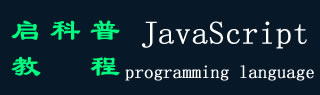
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - typeof 运算符
JavaScript 中的 typeof 运算符是用于获取特定变量的数据类型的一元运算符。它位于其单个操作数之前,该操作数可以是任何类型。它返回一个字符串值,该值指示其操作数的数据类型。JavaScript 包含原始数据类型和非原始数据类型。
JavaScript 数据类型中有七种原始或基本类型:数字、字符串、布尔值、undefined、null、symbol 和 bigint。还有一种称为 object 的复合数据类型。object 数据类型包含三种 sub 数据类型: Object、Array 和 Date。
语法
以下是 typeof 运算符的语法 −
typeof (operand);
我们可以按如下方式编写不带括号的操作数 -
typeof operand;
参数
- operand − 它可以是表示对象或基元的值、变量或表达式。在 JavaScript 中,基元是不是对象且没有方法或属性的数据。
返回值
它返回表示操作数数据类型的字符串值。
typeof 运算符返回的数据类型
下面是 typeof 运算符的返回值列表。
类型 | typeof 返回的字符串 |
---|---|
Number | "number" |
String | "string" |
Boolean | "boolean" |
Object | "object" |
Function | "function" |
Undefined | "undefined" |
Null | "object" |
Symbol | "symbol" |
Bigint | "bigint" |
JavaScript 中有七种原始数据类型: number、string、boolean、bigint、undefined、null 和 symbol。typeof 运算符可用于识别这些原始数据类型或基本数据类型。
typeof 运算符返回除 null 之外的所有基元值的相同数据类型。它为 null 值返回 “object”。
对于 object、date 和 array,它返回 “object” 作为数据类型。
对于函数和类,它返回 “function” 作为数据类型。
让我们使用 typeof 运算符逐个识别这些数据类型。
typeof 10; // 返回 'number'
typeof 'Hello World'; // 返回 'string'
typeof true; // 返回 'boolean'
typeof {name:"qikepu"}; // 返回 'object'
typeof function foo(){};// 返回 'function'
typeof undefined; // 返回 'undefined'
typeof null; // 返回 'object'
typeof Symbol(); // 返回 'symbol'
typeof 10n; // 返回 'bigint'
JavaScript typeof 用于检查数字类型的运算符
在 JavaScript 中,数字类型表示数值。JavaScript 对所有数字使用浮点表示。JavaScript typeof 运算符为所有类型的数字(如整数、浮点、零、Infinity、NaN 等)返回 'number'。
typeof 10; //返回 "number";
typeof -10; //返回 "number";
typeof 0; //返回 "number";
typeof 10.20; //返回 "number";
typeof Math.LN10; //返回 "number";
typeof Infinity; //返回 "number";
typeof NaN; //returns "返回 ";
typeof Number('1'); //返回 "number";
typeof Number('hello'); //返回 "number";
例
下面的示例演示如何使用 typeof 运算符检查数字数据类型。
<html>
<body>
<p> Using typeof operator to check number data type </p>
<div id="output"></div>
<script>
let num = 42;
document.getElementById("output").innerHTML = typeof num;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
number
Set the variable to different value and then try...
JavaScript typeof 运算符来检查字符串类型
字符串表示字符序列。typeof 运算符有助于识别字符串变量。JavaScript typeof 运算符为所有类型的字符串返回 “string”,例如空字符串、字符串、字符串、多行字符串等。
typeof "10"; //返回 "string";
typeof ""; //返回 "string";
typeof "Hello World"; //返回 "string";
typeof String(10); //返回 "string";
typeof typeof 2; //返回 "string";
例
在下面的示例中,我们使用 typeof 运算符来检查字符串数据类型。
<html>
<body>
<div id="output"></div>
<script>
let str = "Hello World";
document.getElementById("output").innerHTML = typeof str;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try...
JavaScript typeof 运算符来检查布尔类型
布尔值表示 true 或 false。tyepof 操作数返回布尔变量的布尔值。
typeof true; //返回 "boolean";
typeof false; //返回 "boolean";
typeof Boolean(10); //返回 "boolean";
例
在下面的示例中,我们使用 typeof 运算符来检查布尔数据类型。
<html>
<body>
<div id="output"></div>
<script>
let bool = true;
document.getElementById("output").innerHTML = typeof bool;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try...
JavaScript typeof 运算符来检查符号类型
符号是在 ES6 中引入的,它提供了一种创建唯一标识符的方法。将 typeof 运算符与符号一起使用将返回 “symbol”。
typeof Symbol(); //返回 "symbol";
typeof Symbol("unique values"); //返回 "symbol";
例
在下面的示例中,我们使用 typeof 运算符来检查 Symbol 数据类型。
<html>
<body>
<div id="output"></div>
<script>
let sym = Symbol("Hello");
document.getElementById("output").innerHTML = typeof sym;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try...
JavaScript typeof 运算符来检查 Undefined 和 Null
“undefined” 类型表示缺少值。“null” 类型表示没有任何对象值。当对 undefined 变量使用 typeof 运算符时,它将返回 'undefined'。令人惊讶的是,将 typeof 运算符与 null 一起使用也会返回 “object”,这是 JavaScript 中已知的怪癖。
typeof undefined; //返回 "undefined";
typeof null; //返回 "object";
请注意,typeof 运算符将对未声明的变量和已声明但未分配的变量返回 “undefined”。
例在下面的示例中,我们使用 typeof 运算符来检查 undefined 数据类型。
<html>
<body>
<div id="output"></div>
<script>
let x;
document.getElementById("output").innerHTML = typeof x;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try...
JavaScript typeof 运算符来检查对象类型
JavaScript typeof 运算符为所有类型的对象(如 JavaScript 对象、数组、日期、正则表达式等)返回 “object”。
const obj = {age: 23};
typeof obj; //返回 "object";
const arr = [1,2,3,4];
typeof arr; //返回 "object";
typeof new Date(); //返回 "object";
typeof new String("Hello World"); //返回 "object";
例
在下面的示例中,我们使用 typeof 运算符来检查对象数据类型。
<html>
<body>
<div id="output"></div>
<script>
const person = {name: "John", age: 34};
document.getElementById("output").innerHTML = typeof person;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try...
JavaScript typeof 运算符检查函数类型
函数是 JavaScript 中的一等公民。JavaScript typeof 运算符为所有类型的函数返回 “function”。有趣的是,它也为 classes 返回 “function”。
const myFunc = function(){返回 "Hello world"};
typeof myFunc; //返回 "function";
const func = new Function();
typeof func; //返回 "function";
class myClass {constructor() { }}
typeof myClass; // 返回 "function";
例
在下面的示例中,我们使用 typeof 运算符来检查函数数据类型。
<html>
<body>
<div id="output"></div>
<script>
const myFunc = function(){return "Hello world"};
document.getElementById("output").innerHTML = typeof myFunc;
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try...
JavaScript typeof 运算符检查 BigInt 类型
typeof 运算符为 BigInt 数字返回 “bigint”。BigInt 值是太大而无法由数字基元表示的数值。
typeof 100n; // 返回 "bigint"
实时开发中的 JavaScript typeof 运算符
例如,开发人员从 API 获取数据。如果只有一个字符串,则 API 返回字符串响应,对于多个字符串,API 返回字符串数组。在这个场景下,开发者需要检查响应的类型是 string 还是 array,如果是 array,则需要遍历 array 的每个 string。
例在下面的示例中,我们检查 'response' 变量的类型并相应地打印其值。
<html>
<body>
<script>
const response = ["Hello", "World!", "How", "are", "you?"];
if (typeof response == "string") {
document.write("The response is - ", response);
} else {
document.write("The response values are : ");
// Traversing the array
for (let val of response) {
document.write(val, " ");
}
}
</script>
</body>
</html>
输出
JavaScript 数组和 typeof 运算符
数组尽管在 JavaScript 中是一种对象类型,但与 typeof 运算符具有不同的行为。
let numbers = [1, 2, 3];
typeof numbers; // Output: 'object'
使用 typeof 运算符时,数组返回 “object”,因此对于精确的数组检测,通常最好使用 Array.isArray()。
例
<html>
<body>
<div id="output"></div>
<script>
let numbers = [1, 2, 3];
document.getElementById("output").innerHTML = Array.isArray(numbers);
</script>
<p>Set the variable to different value and then try...</p>
</body>
</html>
输出
Set the variable to different value and then try..