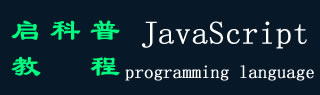
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - ES5
JavaScript ES5,也称为 ECMAScript 5,于 2009 年发布。这是 JavaScript 的第一个主要修订版。它引入了许多新功能,包括 getter 和 setter,以及 'use strict' 指令。ES5 对以前的 JavaScript 版本进行了显著改进。ES5 中引入的新功能使代码更强大、更简洁、更易于维护。
JavaScript ES5 中新增的功能
以下是添加到 ES5 版 JavaScript 中的新方法、功能等。
- 数组 every()
- 数组 filter()
- 数组 forEach()
- 数组 isArray()
- 数组 indexOf()
- 数组 lastIndexOf()
- 数组 map()
- 数组 reduce()
- 数组 reduceRight()
- 数组 some()
- 现在确定日期()
- 日期 toJSON()
- 到 ISOString() 的日期
- 函数 bind()
- JSON 解析()
- JSON 字符串化()
- 多行字符串
- 对象方法
- 对象 defineProperty()
- Property getter 和 setter
- 作为属性名称的保留字
- “使用严格”
- String[number] 访问
- 字符串 trim()
- 尾随逗号
在这里,我们详细解释了每个功能。
JavaScript 数组 every()
array.every() 方法检查数组的每个元素是否遵循特定条件。
例在下面的代码中,我们使用 array.every() 方法来检查数组的每个元素是否为偶数。
<html>
<body>
<div id = "output"> All elements of the array are even? </div>
<script>
const arr = [10, 20, 30, 40, 2, 6];
function even(element) {
return element % 2 == 0;
}
document.getElementById("output").innerHTML += arr.every(even);
</script>
</body>
</html>
输出
JavaScript 数组 filter()
array.filter() 过滤数组元素并获取新数组中过滤的元素。
例在下面的代码中,我们过滤了能被 10 整除的数组元素。
<html>
<body>
<div id = "output"> Elements divisible by 10 are </div>
<script>
const arr = [10, 20, 8, 30, 40, 2, 6];
function divide(element) {
return element % 10 == 0;
}
document.getElementById("output").innerHTML += arr.filter(divide);
</script>
</body>
</html>
输出
JavaScript 数组 forEach()
array.forEach() 遍历数组元素。它类似于 JavaScript 中的循环。
例下面的代码使用 array.forEach() 方法遍历数组并在新行中打印每个数组元素。
<html>
<body>
<div id="output"> </div>
<script>
const arr = ["TATA", "Honda", "Toyota", "Maruti"];
arr.forEach(traverse); // Using the array.forEach() method
function traverse(element) {
document.getElementById("output").innerHTML += element + "<br>";
}
</script>
</body>
</html>
输出
Honda
Toyota
Maruti
JavaScript 数组 isArray()
Array.isArray() 方法检查作为参数传递的对象是否为数组。
例下面的代码使用 Array.isArray() 方法检查 arr 是否为数组。
<html>
<body>
<div id = "output"> </div>
<script>
const arr = ["TATA", "Honda", "Toyota", "Maruti"];
let bool = Array.isArray(arr);
document.getElementById("output").innerHTML += "Is arr array? " + bool;
</script>
</body>
</html>
输出
JavaScript 数组 indexOf()
array.indexOf() 方法返回数组中特定元素的第一个索引。
例下面的代码在数组中找到 23 的第一个索引,即 0。
<html>
<body>
<div id = "output"> The first index of 23 in the array is </div>
<script>
const arr = [23, 45, 32, 12, 23, 56];
document.getElementById("output").innerHTML += arr.indexOf(23);
</script>
</body>
</html>
输出
JavaScript 数组 lastIndexOf()
array.lastIndexOf() 方法返回数组中特定元素的最后一个索引。
例下面的代码查找数组中 45 的最后一个索引,即 4。
<html>
<body>
<div id = "output"> The last index of 45 in the array is </div>
<script>
const arr = [23, 45, 32, 45, 45, 56];
document.getElementById("output").innerHTML += arr.lastIndexOf(45);
</script>
</body>
</html>
输出
JavaScript 数组 map()
array.map() 方法在将当前数组元素与新数组元素映射后返回一个新数组。
例下面的代码使用 map() 方法通过将数组元素加倍来创建新数组。
<html>
<body>
<div id = "output"> The new array is </div>
<script>
const arr = [1, 2, 3, 4, 5];
function doubleEle(element) {
return element * 2;
}
document.getElementById("output").innerHTML += arr.map(doubleEle);
</script>
</body>
</html>
输出
JavaScript 数组 reduce()
array.reduce() 方法将数组缩减为单个值。
例下面的代码使用 array.reduce() 方法将数组的所有元素相乘。
<html>
<body>
<div id = "output">The multiplication result of the array elements is </div>
<script>
const arr = [1, 2, 3, 4, 5];
function multiplier(multiplication, element) {
return multiplication * 2;
}
document.getElementById("output").innerHTML += arr.reduce(multiplier);
</script>
</body>
</html>
输出
JavaScript 数组 reduceRight()
array.reduceRight() 方法从右到左而不是从左到右缩小数组。
<html>
<body>
<div id = "output">The merged array elements in the reverse order is: </div>
<script>
const arr = ["How", "are", "you", "doing?"];
function merge(res, element) {
return res += element + " ";
}
document.getElementById("output").innerHTML += arr.reduceRight(merge);
</script>
</body>
</html>
输出
JavaScript 数组 some()
array.some() 方法用于检查数组的某些元素是否符合特定条件。
例下面的代码检查数组是否包含 1 个或多个长度大于 3 的字符串。
<html>
<body>
<div id = "output">Array contains one or more strings with lengths greater than 3? </div>
<script>
const arr = ["How", "are", "you", "doing?"];
function func_len(element) {
return element.length > 3;
}
document.getElementById("output").innerHTML += arr.some(func_len);
</script>
</body>
</html>
输出
JavaScript Date now()方法
Date.now() 方法用于获取自 1970 年 1 月 1 日以来的总毫秒数。
例下面的代码查找从 1970 年 1 月 1 日开始的总毫秒数。
<html>
<body>
<div id = "output">Total milliseconds since 1st January, 1970 is: </div>
<script>
document.getElementById("output").innerHTML += Date.now();
</script>
</body>
</html>
输出
JavaScript Date toJSON()
date.toJSON() 方法将日期转换为 JSON 日期格式。JSON 日期格式与 ISO 格式相同。ISO 格式为 YYYY-MM-DDTHH:mm:ss.sssZ。
例以下代码以 JSON 格式打印当前日期。
<html>
<body>
<div id = "output">The current date in JSON format is: </div>
<script>
const date = new Date();
document.getElementById("output").innerHTML += date.toJSON();
</script>
</body>
</html>
输出
JavaScript Date toISOString()
date.toISOString() 方法用于将日期转换为 ISO 标准格式。
例下面的代码以标准 ISO 字符串格式打印当前日期。
<html>
<body>
<div id = "output">The current date in ISO string format is: </div>
<script>
const date = new Date();
document.getElementById("output").innerHTML += date.toISOString();
</script>
</body>
</html>
输出
JavaScript 函数 bind()
bind() 方法用于从另一个对象借用该方法。
例在下面的代码中,bird 对象包含 properties 和 printInfo() 方法。animal 对象仅包含 name 和 category 属性。
我们使用 bind() 方法将 bird 对象的 printInfo() 方法借给了 animal 对象。在输出中,printInfo() 方法打印 animal 对象的信息。
<html>
<body>
<div id = "result"> </div>
<script>
const output = document.getElementById("result");
const bird = {
name: "Parrot",
category: "Bird",
printInfo() {
return "The name of the " + this.category + " is - " + this.name;
}
}
const animal = {
name: "Lion",
category: "Animal",
}
const animalInfo = bird.printInfo.bind(animal);
output.innerHTML += animalInfo();
</script>
</body>
</html>
输出
JavaScript JSON 解析()
JSON.parse() 方法用于将字符串转换为 JSON 对象。
例下面给出的代码将字符串转换为对象。此外,我们还在输出中打印了对象属性的值。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById("demo");
const objStr = '{"name":"Sam", "age":40, "city":"Delhi"}';
const obj = JSON.parse(objStr);
output.innerHTML += "The parsed JSON object values are - <br>";
output.innerHTML += "name : " + obj.name + "<br>";
output.innerHTML += "age : " + obj.age + "<br>";
output.innerHTML += "city: " + obj.city + "<br>";
</script>
</body>
</html>
输出
name : Sam
age : 40
city: Delhi
JavaScript JSON stringify()
JSON.stringify() 方法将对象转换为字符串。
例下面的代码使用 JSON.strringify() 方法将 obj 对象转换为字符串。
<html>
<body>
<div id = "output">The object in the string format is - </div>
<script>
const obj = {
message: "Hello World!",
messageType: "Welcome!",
}
document.getElementById("output").innerHTML += JSON.stringify(obj);
</script>
</body>
</html>
输出
JavaScript 多行字符串
您可以使用反斜杠 ('\') 来断开字符串的行并在多行中定义字符串。
例在下面的示例中,我们在多行中定义了 'str' 字符串,并使用反斜杠来换行。
<html>
<body>
<div id = "output"> </div>
<script>
let str = "Hi \
user";
document.getElementById("output").innerHTML += "The string is - " + str;
</script>
</body>
</html>
输出
JavaScript 对象方法
在 ES5 中,添加了与对象相关的方法来操作和保护对象。
操作对象的方法
Sr.No. | 方法 | 描述 |
---|---|---|
1 | create() | 要创建新对象,请使用现有对象作为原型。 |
2 | defineProperty() | 克隆对象并向其原型添加新属性。 |
3 | defineProperties() | 将属性定义到特定对象中并获取更新的对象。 |
4 | getOwnPropertyDescriptor() | 获取对象属性的属性描述符。 |
5 | getOwnPropertyNames() | 获取对象属性。 |
6 | getPrototypeOf() | 获取对象的原型。 |
7 | keys() | 以数组格式获取对象的所有键。 |
保护对象的方法
Sr.No. | 方法 | 描述 |
---|---|---|
1 | freeze() | 防止通过封装对象来添加或更新对象属性。 |
2 | seal() | 封装对象。 |
3 | isFrozen() | 检查对象是否已封装。 |
4 | isSealed() | 检查对象是否封装。 |
5 | isExtensible() | 检查对象是否可扩展。 |
6 | keys() | 以数组格式获取对象的所有键。 |
7 | preventExtensions() | 防止对象的原型更新。 |
JavaScript 对象 defineProperty()
您可以使用 Object.definedProperty() 方法定义对象的单个属性或更新属性值和元数据。
例下面的代码包含 car 对象的 brand、model 和 price 属性。我们使用 defineProperty() 方法来定义对象中的 'gears' 属性。
<html>
<body>
<div id = "output">The obj object is - </div>
<script>
const car = {
brand: "Tata",
model: "Nexon",
price: 1000000,
}
Object.defineProperty(car, "gears", {
value: 6,
writable: true,
enumerable: true,
configurable: true
})
document.getElementById("output").innerHTML += JSON.stringify(car);
</script>
</body>
</html>
输出
JavaScript Property getter 和 setter
getter 和 setter 用于安全地访问和更新对象属性。
例在下面的代码中,watch 对象包含 brand 和 dial 属性。我们使用 get 关键字定义了 getter 来访问 brand 属性值。
此外,我们还使用 set 关键字定义了 setter,以大写形式设置 dial 属性值。
通过这种方式,您可以保护正在更新的对象属性值。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById("demo");
const watch = {
brand: "Titan",
dial: "ROUND",
// Getter to get brand property
get watchBrand() {
return this.brand;
},
// Setter to set Watch dial
set watchDial(dial) {
this.dial = dial.toUpperCase();
}
}
output.innerHTML = "The Watch brand is - " + watch.watchBrand + "<br>";
watch.watchDial = "square";
output.innerHTML += "The Watch dial is - " + watch.dial;
</script>
</body>
</html>
输出
The Watch dial is - SQUARE
JavaScript 保留字作为属性名称
在 ES5 之后,您可以使用反转的单词作为对象中的属性名称。
例在下面的代码中,'delete' 用作对象属性名称。
<html>
<body>
<div id = "output">The obj object is - </div>
<script>
const obj = {
name: "Babo",
delete: "Yes",
}
document.getElementById("output").innerHTML += JSON.stringify(obj);
</script>
</body>
</html>
输出
JavaScript “use strict”
严格模式也在 ES5 中引入。
严格模式允许您克服错误并编写明文代码。你可以使用 'use strict' 指令来声明 strict 模式。
例在下面的代码中,您可以取消注释 'num = 43' 行并观察错误。严格模式不允许开发人员在不使用 var、let 或 const 关键字的情况下定义变量。
<html>
<body>
<div id = "output">The value of num is: </div>
<script>
"use strict";
let num = 43; // This is valid
// num = 43; // This is invalid
document.getElementById("output").innerHTML += num + "<br>";
</script>
</body>
</html>
输出
JavaScript String[number] 访问权限
在 ES5 之前,charAt() 方法用于从特定索引访问字符串字符。
在 ES5 之后,您可以将字符串视为字符数组,并在访问数组元素时从特定索引访问字符串字符。
<html>
<body>
<div id = "output1">The first character in the string is: </div>
<div id = "output2">The third character in the string is: </div>
<script>
let str = "Apple";
document.getElementById("output1").innerHTML += str[0];
document.getElementById("output2").innerHTML += str[2];
</script>
</body>
</html>
输出
The third character in the string is: p
JavaScript 字符串 trim()
string.trim() 方法删除两端的空格。
例在下面的代码中,我们使用 string.trim() 方法从 str 字符串的开头和结尾删除空格。
<html>
<body>
<div id = "output"> </div>
<script>
let str = " Hi, I am a string. ";
document.getElementById("output").innerHTML = str.trim();
</script>
</body>
</html>
输出
JavaScript 尾随逗号
您可以在数组和对象中添加尾随逗号(最后一个元素后的逗号)。
例在下面的代码中,我们在最后一个对象属性和数组元素后添加了尾随逗号。代码将正常运行并打印输出。
<html>
<body>
<div id = "demo1"> </div>
<div id = "demo2"> </div>
<script>
const obj = {
name: "Pinku",
profession: "Student",
}
document.getElementById("demo1").innerHTML =JSON.stringify(obj);
let strs = ["Hello", "world!",];
document.getElementById("demo2").innerHTML = strs;
</script>
</body>
</html>
输出
Hello,world!
注意 – JSON 字符串不允许使用尾随逗号。
例如
let numbers = '{"num1": 10,"num2": 20,}';
JSON.parse(numbers); // Invalid
numbers ='{"num1": 10,"num2": 20}';
JSON.parse(numbers); // Valid
在 ES5 中,主要引入了与数组和对象相关的方法。