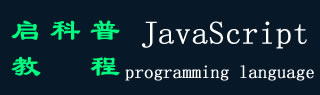
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - 扩展错误
JavaScript 中的自定义错误是您自己创建的错误,而不是 JavaScript 引发的内置错误。您可以创建自定义错误来处理代码中可能发生的特定类型的错误。
要创建自定义错误,您可以使用 Error 构造函数。Error 构造函数将字符串作为其参数,该字符串将是错误的消息。
扩展 Error 类:创建自定义错误
创建自定义错误的最佳方法是创建一个新类并使用 'extends' 关键字扩展它。它使用继承的概念,自定义 error 类继承 Error 类的属性。
在 constructor() 函数中,您可以初始化自定义 error 类的属性。
语法
您可以按照以下语法通过扩展 Error 类来创建自定义错误。
class customError extends Error {
constructor(message) {
super(message)
// 初始化属性
}
}
在上面的代码中,我们使用 super() 方法调用父类构造函数。
您还可以在构造函数中初始化 customError 类的属性。
例在下面的代码中,我们获取用户的输入。当用户单击检查年龄按钮时,它会调用 checkAge() 函数。
我们在 JavaScript 代码中定义了 ageError 类,并使用 Error 类对其进行了扩展。在 ageError 类中,我们添加了 constructor() 函数来初始化属性。
在 constructor() 函数中,我们使用 super() 方法来初始化 Error 类的 message 属性。此外,我们还在 constructor 函数中初始化了 'name' 和 'age' 属性。
在 checkAge() 函数中,如果 age 小于 18,则抛出错误,在 catch{} 块中,我们打印错误消息和 age。
<html>
<body>
<p>Enter your age: <input type = "number" id = "age" /> </p>
<button onclick = "checkAge()"> Check Age </button>
<p id = "demo"> </p>
<script>
const output = document.getElementById("demo");
class ageError extends Error {
constructor(message, age) {
super(message);
this.name = "ageError";
this.age = age // 自定义特性
}
}
function checkAge() {
const ageValue = document.getElementById('age').value;
try {
if (ageValue < 18) { // 年龄小于18岁时抛出错误
throw new ageError("You are too young", ageValue);
} else {
output.innerHTML = "You are old enough";
}
} catch (e) {
output.innerHTML = "Error: " + e.message + ". <br>";
output.innerHTML += "Age: " + e.age + "<br>";
}
}
</script>
</body>
</html>
输出
Check Age
Error: You are too young.
Age: 5
如果您只想为自定义错误创建多个新类,以提供阐明的错误类型和消息,并且不想更改 Error 类的属性,则可以使用以下语法。
class InputError extends Error {};
让我们通过下面的示例来学习它。
例在下面的代码中,我们创建了 3 个不同的自定义类,并使用 Error 类扩展它们以创建自定义错误。
在 try{} 块中,我们抛出 StringError。
在 catch{} 块中,我们使用 instanceOf 运算符来检查错误对象的类型并相应地打印错误消息。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById("demo");
class StringError extends Error { };
class NumberError extends Error { };
class BooleanError extends Error { };
try {
throw new StringError("This is a string error");
} catch (e) {
if (e instanceof StringError) {
output.innerHTML = "String Error";
} else if (e instanceof NumberError) {
output.innerHTML = "Number Error";
} else if (e instanceof BooleanError) {
output.innerHTML = "Boolean Error";
} else {
output.innerHTML = "Unknown Error";
}
}
</script>
</body>
</html>
输出
多级继承
您可以通过使用 Error 类扩展常规自定义错误来创建它。之后,您可以扩展自定义错误类以创建更通用的错误类。
让我们通过下面的示例来理解它。
例在下面的代码中,我们定义了 'NotFound' 类,并使用 Error 类对其进行扩展。
之后,我们定义了 'propertyNotFound' 和 'valueNotFound' 类,并使用 'NotFound' 类扩展了它们。在这里,我们完成了多级继承。
在 try 块中,如果数组不包含 6,则抛出 valueNotFound 错误。
在 catch 块中,我们打印错误。
<html>
<body>
<div id = "output"> </div>
<script>
const output = document.getElementById("output");
class NotFound extends Error {
constructor(message) {
super(message);
this.name = "NotFound";
}
}
// 进一步继承
class propertyNotFound extends NotFound {
constructor(message) {
super(message);
this.name = "propertyNotFound";
}
}
// 进一步继承
class ElementNotFound extends NotFound {
constructor(message) {
super(message);
this.name = "ElementNotFound";
}
}
try {
let arr = [1, 2, 3, 4, 5];
// 如果数组不包含6,则抛出错误
if (!arr.includes(6)) {
throw new propertyNotFound("Array doesn't contain 6");
}
} catch (e) {
output.innerHTML = e.name + ": " + e.message;
}
</script>
</body>
</html>
输出
如果任何对象不包含特定属性,您还可以引发 propertyNotFound 错误。