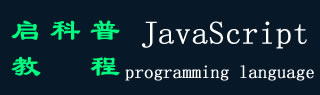
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - 严格模式
JavaScript 中的严格模式
在 JavaScript 中,严格模式(Strict Mode)是在 ES5 (ECMAScript 2009) 中引入的。引入 “严格模式” 的目的是使 JavaScript 代码更安全。
'use strict' 文本表达式用于在 JavaScript 代码中添加 严格模式(Strict Mode) 。它从代码中删除了静默错误,例如你不能在没有声明的情况下使用变量,你不能修改对象的 readable 属性等。
启用严格模式(Strict Mode)
要 enble strcit 模式,您应该将以下文本表达式写入代码顶部 -
'use strict';
使用 'use strict' 指令启用 JavaScript 的严格模式。
为什么使用 Strict 模式?
在这里,我们列出了使用严格 JavaScript 模式的一些原因 -
- 错误预防 - 严格模式可防止开发人员在编写 JavaScript 代码时犯的常见错误,例如在不声明的情况下初始化变量或使用保留关键字作为标识符。
- 更安全的代码 − 严格模式可防止意外创建全局变量。此外,它不允许使用像 'with' 这样的语句,这可能会导致代码中的漏洞。
- 未来兼容性 − 您可以使用脚本模式将代码与 JavaScript 的未来版本保持一致。例如,当前版本的 JavaScript 不包含 'public' 等关键字,而是为将来的版本保留的。因此,从现在开始,严格模式将不允许将其用作标识符。
全局范围内的 Strict 模式
当您在 JavaScript 代码顶部添加 'use strict' 时;它对整个代码使用 strict 模式。
例在下面的示例中,我们定义了 'y' 变量并使用 50 对其进行初始化。该代码在输出中打印 'y' 的值。
此外,我们初始化了变量 'x' 但没有声明它。因此,它会在控制台中提供错误,并且不会打印输出。
简而言之,strict 模式不允许在没有声明的情况下使用变量。
<html>
<head>
<title> Using the strict mode gloablly </title>
</head>
<body>
<script>
"use strict";
let y = 50; // This is valid
document.write("The value of the X is: " + y);
x = 100; // This is not valid
document.write("The value of the X is: " + x);
</script>
</body>
</html>
本地范围内的 strict 模式
您还可以在特定函数中使用 “strict mode”。因此,它只会应用于函数范围。让我们借助一个例子来理解它。
例在下面的示例中,我们只在 test() 函数中使用了 'use strict' 字面量。因此,它仅从函数中删除异常错误。
下面的代码允许您初始化变量,而无需在函数外部声明变量,但不在函数内部声明变量。
<html>
<head>
<title> Using the strict mode gloablly </title>
</head>
<body>
<script>
x = 100; // This is valid
document.write("The value of the X is - " + x);
function test() {
"use strict";
y = 50; // This is not valid
document.write("The value of the y is: " + x);
}
test();
</script>
</body>
</html>
在 strict 模式下不应犯的错误
1. 如果不声明变量,就不能用值初始化变量。
<script>
'use strict';
num = 70.90; // This is invalid
</script>
2. 同样,如果不声明对象,就不能使用它。
<script>
'use strict';
numObj = {a: 89, b: 10.23}; // This is invalid
</script>
3. 您无法使用 delete 关键字删除对象。
<script>
'use strict';
let women = { name: "Aasha", age: 29 };
delete women; // This is invalid
</script>
4. 在 strict 模式下,您无法删除对象原型。
<script>
'use strict';
let women = { name: "Aasha", age: 29 };
delete women.prototype; // This is invalid
</script>
5. 不允许使用 delete 运算符删除函数。
<script>
'use strict';
function func() { }
delete func; // This is invalid
</script>
6. 您不能拥有具有重复参数值的函数。
<script>
'use strict';
function func(param1, param1, param2) {
// Function with 2 param1 is not allowed!
}
</script>
7. 您不能为变量分配八进制数。
<script>
'use strict';
let octal = 010; // Throws an error
</script>
8. 您不能使用转义字符。
<script>
'use strict';
let octal = \010; // Throws an error
</script>
9. 您不能使用 eval、arguments、public 等保留关键字作为标识符。
<script>
'use strict';
let public = 100; // Throws an error
</script>
10. 不能写入对象的 readable 属性。
<script>
'use strict';
let person = {};
Object.defineProperty(person, 'name', { value: "abc", writable: false });
obj1.name = "JavaScript"; // throws an error
</script>
11. 您不能为 getters 函数赋值。
<script>
'use strict';
let person = { get name() { return "JavaScript"; } };
obj1.name = "JavaScript"; // throws an error
</script>
12. 在 strict 模式下,当您在函数中使用 'this' 关键字时,它引用调用函数的引用对象。如果未指定 reference 对象,则它引用 undefined 值。
<script>
'use strict';
function test() {
console.log(this); // Undefined
}
test();
</script>
13. 您不能在 strict 模式下使用 'with' 语句。
<script>
'use strict';
with (Math) {x = sin(2)}; // This will throw an error
</script>
14. 出于安全原因,您不能使用 eval() 函数来声明变量。
<script>
'use strict';
eval("a = 8")
</script>
15. 您不能将关键字用作为将来保留的标识符。以下关键字保留给将来 -
- implements
- interface
- package
- private
- protected