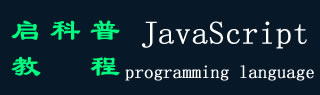
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - 变量范围
JavaScript 变量范围
JavaScript 中的变量范围决定了变量在代码不同部分的可访问性和可见性。范围是代码执行的当前上下文。这意味着变量范围由它的执行位置决定,而不是由它的声明位置决定。
在 JavaScript 中,对象和函数也是变量。因此,JavaScript 变量范围也决定了程序中对象和函数的可访问性或可见性。
学习 JavaScript 中的变量范围以编写清晰的代码并避免命名冲突至关重要。
JavaScript 中有以下类型的变量范围。
- 块范围(Block scope)
- 函数范围(Function scope)
- 本地范围(Local scope)
- 全局范围(Global scope)
在这里,我们将介绍块、函数和本地范围。我们将在 JavaScript 全局变量章节中讨论 detain 中的全局范围。
JavaScript 块范围
在 JavaScript ES6 之前,只有 Global 和 Function 范围。ES6 引入了 let 和 const 关键字。这些关键字在 JavaScript 中提供 Block Scope。
在 { } 块中使用 'let' 和 'const' 关键字定义的 JavaScript 变量只能在定义它们的块内访问。
{
let x = 10; // x is accessible here
}
//x is not accessible here
使用 var 关键字定义的变量 is 不提供块范围。
{
var x = 10; // x is accessible here
}
//x is accessible here also
例
在下面的示例中,我们在 'if' 块中定义了变量 'a'。在输出中,你可以看到变量 'a' 只能在 'if' 块内访问。如果你试图在 'if' 块之外访问变量 'a',它将抛出一个引用错误,比如 'variable a is not defined'。
<html>
<head>
<title> JavaScript - Block scope </title>
</head>
<body>
<p id = "output"> </p>
<script>
if (true) {
let a = 10;
document.getElementById("output").innerHTML = "a = " + a;
}
// a can't be accessed here
</script>
</body>
</html>
输出
例
在下面的代码中,我们定义了 test() 函数。在该函数中,我们使用大括号添加了一个 { } 块,在 { } 块中,我们定义了变量 'x'。变量 'x' 不能在 { } 块之外访问,因为它具有块范围。
<html>
<head>
<title> JavaScript - Block scope </title>
</head>
<body>
<p id = "demo"> </p>
<script>
const output = document.getElementById("demo");
function test() {
{
let x = 30;
output.innerHTML = "x = " + x;
}
// variable x is not accessible here
}
test();
</script>
</body>
</html>
输出
每当你在块内使用 'let' 或 'const' 关键字定义变量时,如 loop 块、if-else 块等,它都不能在块外访问。
JavaScript 函数范围
在 JavaScript 中,每个函数都会创建一个范围。函数中定义的变量具有函数范围。函数中定义的变量只能从同一函数中访问。这些变量不能从函数外部访问。
每当你在函数中使用 'var' 关键字定义变量时,该变量可以在整个函数中访问,即使它是在特定块内定义的。
例如
function func() {
{
var x; // function scope
let y; // Block scope
const z = 20; // Block scope
}
// x is accessible here, but not y & z
}
例
在下面的示例中,我们分别使用 var、let 和 const 关键字定义了变量 'x'、'y' 和 'z'。变量 'x' 可以在函数内部的任何地方访问,因为它具有函数范围,但 'y' 和 'z' 只能在定义它的块内访问。
<html>
<head>
<title> JavaScript - Function scope </title>
</head>
<body>
<p id = "demo"> </p>
<script>
const output = document.getElementById("demo");
function func() {
{
var x = 30;
let y = 20;
const z = 10;
output.innerHTML += "x -> Inside the block = " + x + "<br>";
output.innerHTML += "y -> Inside the block = " + y + "<br>";
output.innerHTML += "z -> Inside the block = " + z + "<br>";
}
output.innerHTML += "x -> Outside the block = " + x + "<br>";
// y and z can't be accessible here
}
func();
</script>
</body>
</html>
输出
y -> Inside the block = 20
z -> Inside the block = 10
x -> Outside the block = 30
JavaScript 本地范围
JavaScript 本地范围是函数和块范围的组合。JavaScript 编译器在调用函数时创建一个局部变量,并在函数调用完成时将其删除。
简而言之,在函数或块中定义的变量是该特定范围的本地变量。函数参数被视为函数内部的局部变量。
例在下面的代码中,我们使用 var、let 和 const 关键字定义了函数内的变量。所有变量都是函数的本地变量。它不能在函数之外访问。
同样,我们可以在 local 作用域中定义循环变量。
<html>
<head>
<title> JavaScript - Local scope </title>
</head>
<body>
<p id = "demo"> </p>
<script>
const output = document.getElementById("demo");
function func() {
let first = 34;
var second = 45;
const third = 60;
output.innerHTML += "First -> " + first + "<br>";
output.innerHTML += "Second -> " + second + "<br>";
output.innerHTML += "Third -> " + third + "<br>";
}
func();
</script>
</body>
</html>
输出
Second -> 45
Third -> 60
我们注意到,当在函数中定义变量时,该变量将成为函数的 Local。在这种情况下,变量具有函数范围。当一个变量在特定块中定义时,它将成为该块的本地变量,并具有块范围。