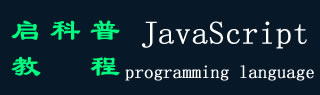
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - new 关键字
JavaScript 中的 new 关键字用于创建具有 constructor 函数的对象的实例。使用 new 关键字,我们可以创建用户定义的和内置对象类型的实例。我们可以创建 classes、prototype 或 constructor 函数的实例。
当使用 new 关键字调用 JavaScript 函数时,该函数将用作构造函数。new 关键字将执行以下操作:
- 创建一个空白/空的 JavaScript 对象。
- 设置其原型以进行继承。
- 在内部将此变量与新创建的对象绑定。
- 每当使用 new crated 对象时,使用 new crated 对象执行构造函数。
- 返回新创建的对象,除非 contractor 函数返回非 null 对象引用。
new 关键字还用于创建内置 JavaScript 对象(如 String、Boolean、Number 等)的实例。
语法
在 JavaScript 中使用 new 关键字的语法如下 -
new Constructor(arguments);
参数
- Constructor − 它是构造函数或类名称的名称。
- arguments − 可以是多个参数来使用它们初始化对象属性。
返回值
- 如果 Constructor 不返回任何内容或原始值,则返回新创建的对象。
- 如果 Constructor 返回非原始值,则返回 constructor 函数返回的值。
在函数构造函数中使用 'new' 关键字
要将函数用作构造函数,我们应该使用 new 关键字调用函数,将其放在函数名称之前。
我们可以使用 constructor 函数定义多个对象。函数构造函数用作对象的原型。
按照下面的语法使用 'new' 关键字和 constructor 函数来定义对象。
new FuncName(arguments);
例
我们在下面的代码中定义了 Watch() 构造函数。
Watch() 构造函数初始化 brand、price 和 type 属性。
之后,我们创建了 Watch() 函数的两个新实例,并将它们打印在输出中。
<html>
<body>
<div id = "output"> </div>
<script>
function Watch(brand, price, type) {
this.brand = brand;
this.price = price;
this.type = type;
}
const titan = new Watch('titen', 4000, 'analog');
const sonata = new Watch('sonata', 3000, 'digital');
document.getElementById('output').innerHTML +=
"The titan object is: " + JSON.stringify(titan) + "<br>" +
"The sonata object is: " + JSON.stringify(sonata);
</script>
</body>
</html>
输出
The sonata object is: {"brand":"sonata","price":3000,"type":"digital"}
例
在下面的代码中,我们定义了 Laptop() 构造函数,初始化与笔记本电脑相关的各种属性。此外,我们还定义了 getLaptop() 函数,该函数打印笔记本电脑的详细信息。
之后,我们创建了 Laptop() 对象的两个实例,并将 getLaptop() 方法用于这两个实例。这样,您可以与不同的对象共享单个方法。
<html>
<body>
<div id = "output"> </div>
<script>
const output = document.getElementById('output');
function Laptop(brand, model, processor) {
this.brand = brand;
this.model = model;
this.processor = processor;
this.getLaptop = function () {
output.innerHTML += this.brand + ", " +
this.model + ", " + this.processor + "<br>";
}
}
const HP = new Laptop('HP', "VIKING", "i7");
const Dell = new Laptop('Dell', "Inspiron", "i5");
HP.getLaptop();
Dell.getLaptop();
</script>
</body>
</html>
输出
Dell, Inspiron, i5
在 Class 中使用 'new' 关键字
您还可以使用 new 关键字来定义类的实例。该类还提供对象的蓝图。
在 ES6 之前,构造函数用于定义对象的模板。在 ES6 之后,类用于定义对象的模板。
例在下面的示例中,我们定义了 'WindowClass 类。在 'WindowClass' 中,我们添加了构造函数来初始化属性。我们还在类中添加了 getDetails() 方法。
之后,我们使用 'new' 关键字后跟类名来定义 WindowClass 的对象。
接下来,我们在类的实例上调用 getDetails() 方法。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo')
class WindowClass {
constructor(color, size, type) {
this.color = color;
this.size = size;
this.type = type;
}
getDetails = function () {
output.innerHTML =
"Color: " + this.color + "<br>" +
"Size: " + this.size + "<br>" +
"Type: " + this.type;
}
}
// Creating the instance of WindowClass class
const window1 = new WindowClass('blue', 'small', 'wooden');
// Calling function object
window1.getDetails();
</script>
</body>
</html>
输出
Size: small
Type: wooden
将 'new' 关键字与内置对象一起使用
您还可以使用 'new' 关键字来定义具有构造函数的内置对象的实例。
按照下面的语法创建内置对象 Number 的实例。
const num = new Number(10);
在上面的语法中,我们已将数值作为 Number() 构造函数的参数传递。
例在下面的代码中,我们使用了 Number() 和 String() 构造函数以及一个 new 关键字来定义 numeric 和 string 对象。
之后,我们使用 'typeof' 运算符来检查 num 和 str 变量的类型。在输出中,您可以看到 num 和 str 变量类型是一个对象。
<html>
<body>
<div id = "output"> </div>
<script>
const num = new Number(10);
const str = new String("Hello");
document.getElementById("output").innerHTML =
"num = " + num + ", typeof num " + typeof num + "<br>" +
"str = " + str + ", typeof str " + typeof str;
</script>
</body>
</html>
输出
str = Hello, typeof str object