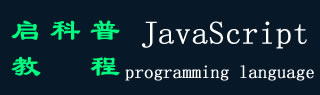
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - 对象访问器
JavaScript 中的对象访问器属性是获取或设置对象值的方法。它们是使用 get 和 set 关键字定义的。访问器属性是控制访问和修改对象方式的强大方法。
JavaScript 对象可以具有两种属性。
- 数据属性
- 访问器属性
以下属性称为 data 属性。
const obj = {
key: "value", // Data property
}
对象访问器属性
在 JavaScript 中,你可以使用 getter 来获取对象属性,使用 setter 来设置对象属性。
有两个关键字可用于定义访问器属性。
- get − get 关键字用于定义获取对象属性值的方法。
- set − set 关键字用于定义更新对象属性值的方法。
JavaScript Getter
getter 用于访问对象属性。要将方法定义为 getter,我们使用 get 关键字,后跟方法名称。按照下面的语法定义 getter。
get methodName() {
// Method body
}
obj.methodName;
在上面的语法中,我们使用 'get' 关键字后跟方法名称来定义 getter。
您可以使用方法名称作为对象属性来获取其返回值。
例
在下面的示例中,在 wall 对象中,我们定义了 getColor() getter。getColor() 返回 color 属性的值。
之后,我们使用 getColor() 方法访问对象的 color 属性值。
<html>
<body>
<p id = "output">The color of the wall is : </p>
<script>
const wall = {
color: "brown",
get getColor() {
return this.color;
}
}
document.getElementById("output").innerHTML += wall.getColor;
</script>
</body>
</html>
输出
JavaScript 资源库
setter 用于更新 JavaScript 对象属性。要将方法定义为 setter,我们使用 set 关键字后跟方法名称您可以按照以下语法在 JavaScript 对象中定义 setter。
set methodName(param) { // Setter method
return this.color = color;
}
wall.methodName = "Red";
- 在上面的语法中,'set' 关键字用于定义 setter 方法。
- method_name 可以是任何有效的标识符。
- setter 方法始终采用单个参数。如果您不传递一个或多个参数,则会出现错误。
- 在为属性分配 value 时,您可以为 setter 方法分配 value。
在下面的示例中,我们定义了 setter 方法来设置 wall 对象的 color 属性的值。我们使用 'setColor' setter 方法将 'red' 值设置为对象的 color 属性。
<html>
<body>
<p id = "output"> </p>
<script>
const wall = {
color: "brown",
set setColor(color) {
return this.color = color;
}
}
document.getElementById("output").innerHTML +=
"The color of the wall before update is : " + wall.color + "<br>";
//updating the color of wall
wall.setColor = "Red";
document.getElementById("output").innerHTML +=
"The color of the wall after update is : " + wall.color;
</script>
</body>
</html>
输出
The color of the wall after update is : Red
JavaScript 对象方法与 Getter/Setter
在 JavaScript 中,无论你可以使用 getter 和 setter 做什么,你也可以通过定义特定的对象方法来做。区别在于 getter 和 setter 提供更简单的语法。
让我们借助一些示例来理解它。
例在下面的示例中,我们在 wall 对象中定义了 getColor() getters 方法和 colorMethod() 对象方法。Both 方法都返回 color 值。
你可以观察到,定义和访问 getter 比定义和访问 object 方法更简单。
<html>
<body>
<p id = "output"> </p>
<script>
const wall = {
color: "brown",
get getColor() {
return this.color;
},
colorMethod: function () {
return this.color;
}
}
document.getElementById("output").innerHTML +=
"Getting the color using the getters : " + wall.getColor + "<br>";
document.getElementById("output").innerHTML +=
"Getting the color using the object method : " + wall.colorMethod();
</script>
</body>
</html>
输出
Getting the color using the object method : brown
数据质量和安全性
getter 和 setter 方法可以提供更好的数据质量。此外,它们还用于封装以保护对象数据。
让我们通过下面的示例了解 getter 和 setter 如何提高数据质量并提供安全性。
例在下面的示例中,getColor() 是一个 getter 方法,在将 color 属性转换为大写后返回该属性的值。此外,它还对用户隐藏了 color 属性,因为您可以使用 getColor() 方法访问其值。
<html>
<body>
<p id = "output"> </p>
<script>
const wall = {
color: "Brown",
get getColor() {
return this.color.toUpperCase();
}
}
document.getElementById("output").innerHTML +=
"Getting the color using the getters : " + wall.getColor;
</script>
</body>
</html>
输出
用 Object.defineProperty() 定义 getter/setter
您还可以使用 Object.defineProperty() 方法将 getter 或 setter 添加到对象中。
Object.defineProperty(object, "methodName", {
keyword: function () {
// Method body
},
})
使用上述语法,您可以定义与 methodName 相同的 getter 或 setter。
参数
- object − 它是需要添加 getter 或 setter 的对象。
- methodName − 它是 getter 或 setter 的方法名称。
- keyword − 它可以是 'get' 或 'set',具体取决于你想要定义 getter 或 setter 方法。
在下面的示例中,我们使用 object.defineProperty() 方法将 getSize 和 setSize getter 和 setter 添加到对象中。
之后,我们使用 getSize 和 setSize 分别获取和设置大小。
<html>
<body>
<p id = "demo"> </p>
<script>
const output = document.getElementById("demo");
const door = {
size: 20,
}
Object.defineProperty(door, "getSize", {
get: function () {
return this.size;
}
});
Object.defineProperty(door, "setSize", {
set: function (value) {
this.size = value;
},
})
output.innerHTML += "Door size is : " + door.getSize + "<br>";
door.setSize = 30;
output.innerHTML += "Door size after update is : " + door.getSize;
</script>
</body>
</html>
输出
Door size after update is : 30
使用 getter 和 setter 的原因
以下是在 JavaScript 中使用 getter 和 setter 的好处。
- 它的语法比 object 方法简单。
- 通过验证数据来提高数据质量。
- 用于封装或保护数据。
- 它还允许抽象或数据隐藏。