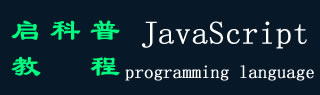
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - this 关键字
什么是 'this' 关键词?
在 JavaScript 中,'this' 关键字包含对对象的引用。它表示函数或当前代码的上下文。它用于访问当前对象的属性和方法。
在函数中使用 this 关键字时,this 将引用调用函数时使用的对象。
在 JavaScript 中,函数也是对象。因此,您也可以将 'this' 关键字与 Functions 一起使用。
“this”指的是哪个对象?
'this' 关键字引用的对象取决于您如何使用 'this' 关键字。
例如
- 'this' 关键字引用全局范围内的 window 对象。
- 当你在函数中使用 'this' 关键字时,它也表示 'window' 对象。
- 'this' 关键字是指函数中 strict 模式下的 undefined。
- 在 object 方法中使用 'this' 关键字时引用该对象。
- 在事件处理程序中,'this' 关键字引用执行事件的元素。
- call()、apply() 和 bind() 等方法中的 'this' 关键字可以引用不同的对象。
语法
按照下面的语法在 JavaScript 中使用 'this' 关键字 &minus
this.property
OR
this.method();
您可以使用 'this' 关键字访问属性并执行对象的方法。
全局范围内的 JavaScript 'this'
在全局范围内使用 'this' 关键字时,它表示全局 (window) 对象。您可以在全局范围内使用 'this' 关键字访问全局变量。
例在下面的代码中,我们在全局范围内定义了 'num' 变量和 printNum() 函数。之后,我们使用 'this' 关键字来访问全局变量和函数。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo');
var num = 10;
function printNum() {
output.innerHTML += "Inside the function: " + num + "<br>";
}
this.printNum();
output.innerHTML += "Outside the function: " + this.num + "<br>";
</script>
</body>
</html>
输出
Outside the function: 10
函数中的 JavaScript 'this'
当你在函数中使用 'this' 关键字时,它表示全局范围或 'window' 对象。
例在下面的代码中,我们在函数中使用 'this' 关键字。你可以观察到,我们使用函数内的 'this' 关键字来访问全局变量。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo');
var message = "Hello World!";
function printMessage() {
var message = "Hi! How are you?";
output.innerHTML = "The messsage is: " + this.message;
}
printMessage();
</script>
</body>
</html>
输出
严格模式下的函数中的 'this'
当你在 strict 模式下在函数中使用 'this' 关键字时,它不会引用任何对象。'this' 关键字的值变为 undefined。
例在下面的代码中,我们在 strict 模式下在函数内部使用 'this' 关键字。它打印 undefined。
<html>
<body>
<div id = "demo"> </div>
<script>
let output = document.getElementById('demo');
var message = "Hello World!";
function test() {
"use strict";
output.innerHTML = "The value of this in the strict mode is: " + this;
}
test();
</script>
</body>
</html>
输出
构造函数中的 'this'
当您使用函数作为构造函数创建对象时,“this”关键字引用该对象。
例我们在下面的代码中定义了 Animal() 构造函数。我们在构造函数中使用了 'this' 关键字来初始化对象的属性。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo');
function Animal() {
this.name = 'Lion';
this.age = 3;
this.color = 'Brown';
}
const newAnimal = new Animal();
output.innerHTML = "Animal Name: " + newAnimal.name + "<br>";
output.innerHTML += "Animal Age: " + newAnimal.age + "<br>";
output.innerHTML += "Animal Color: " + newAnimal.color;
</script>
</body>
</html>
输出
Animal Age: 3
Animal Color: Brown
箭头函数中的 'this'
当您在箭头函数中使用 'this' 关键字时,它引用其父对象的范围。
例如,当您在 object 方法中定义 arrow 函数并在其中使用 'this' 关键字时,它会呈现对象。如果你在另一个函数中定义箭头函数,则 'this' 关键字引用全局对象。
例在下面的代码中,我们在对象的 getDetails() 方法中定义了箭头函数。当我们打印 'this' 关键字的值时,它会打印对象。
<html>
<body>
<div id = "output1">Value of 'this' inside the getDetails() method: </div>
<div id = "output2">Value of 'this' inside the getInnerDetails() method: </div>
<script>
const wall = {
size: "10",
color: "blue",
getDetails() {
document.getElementById('output1').innerHTML += JSON.stringify(this);
const getInnerDetails = () => {
document.getElementById('output2').innerHTML += JSON.stringify(this);
}
getInnerDetails();
}
}
wall.getDetails();
</script>
</body>
</html>
输出
Value of 'this' inside the getInnerDetails() method: {"size":"10","color":"blue"}
object 方法中的 'this'
当你在 object 方法中使用 'this' 关键字时,它表示对象本身。
例在下面的代码中,我们定义了 'fruit;对象。该对象包含 printFruitDetails() 方法,在该方法中,我们使用 'this' 关键字来访问对象的属性。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo');
const fruit = {
name: "Apple",
color: "red",
printFruitDetails() {
output.innerHTML += "Furit Name = " + this.name + "<br>";
output.innerHTML += "Fruit Color = " + this.color;
}
}
fruit.printFruitDetails();
</script>
</body>
</html>
输出
Fruit Color = red
object 方法的子函数中的 'this'
当您在 object 方法中定义函数并在函数中使用 'this' 关键字时,它表示全局对象而不是对象。
例:在下面的代码中,我们定义了 person 对象。person 对象包含 printDetails() 方法。在 printDetails() 方法中,我们定义了 printThis() 函数。
在 printThis() 函数中,我们打印 'this' 关键字的值,然后它打印全局对象。
<html>
<body>
<div id = "output">Inside the printThis() function, Value of 'this' = </div>
<script>
const person = {
name: "Salman",
isBusinessman: false,
printDetails() {
function printThis() {
document.getElementById('output').innerHTML += this;
}
printThis();
}
}
person.printDetails();
</script>
</body>
</html>
输出
事件处理程序中的 JavaScript 'this'
将 'this' 关键字与事件处理程序一起使用是指执行事件的 HTML 元素。
例在下面的代码中,我们已将 onClick 事件处理程序添加到 <div> 元素中。当用户点击 div 元素时,我们使用 'display' 属性隐藏 div 元素。
<html>
<head>
<style>
div {
height: 200px;
width: 700px;
background-color: red;
}
</style>
</head>
<body>
<p>Click the DIV below to remove it. </p>
<div onclick = "this.style.display = 'none'"> </div>
</body>
</html>
JavaScript 显式函数绑定
在 JavaScript 中,call()、apply() 或 bind() 方法用于显式绑定。
显式绑定允许您借用特定对象的方法。使用这些方法,您可以显式定义 'this' 关键字的上下文。
让我们使用下面的示例来理解显式绑定。
示例:使用 call() 方法
在下面的代码中,lion 对象包含 color 和 age 属性。它还包含 printDetails() 方法,并使用 'this' 关键字打印详细信息。
tiger 对象仅包含 color 和 age 属性。我们使用 call() 方法通过 tiger 对象的上下文调用 lion 对象的 printDetails() 方法。因此,该方法在输出中打印 tiger 的详细信息。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo');
const lion = {
color: "Yellow",
age: 10,
printDetails() {
output.innerHTML += `<p>Color: ${this.color}</p>`;
output.innerHTML += `<p>Age: ${this.age}</p>`;
}
}
const tiger = {
color: "Orange",
age: 15,
}
lion.printDetails.call(tiger);
</script>
</body>
</html>
输出
Age: 15
示例:使用 bind() 方法
下面的代码还包含 lion 和 tiger 对象。之后,我们使用 bind() 方法将 lion 对象的 printDetails() 方法绑定到 tiger 对象中。
之后,我们使用 tigerDetails() 方法打印 tiger 对象的详细信息。
<html>
<body>
<div id = "demo"> </div>
<script>
const output = document.getElementById('demo');
const lion = {
color: "Yellow",
age: 10,
printDetails() {
output.innerHTML += `<p>Color: ${this.color}</p>`;
output.innerHTML += `<p>Age: ${this.age}</p>`;
}
}
const tiger = {
color: "Orange",
age: 15,
}
const tigerDetails = lion.printDetails.bind(tiger);
tigerDetails();
</script>
</body>
</html>
输出
Age: 15
JavaScript 'this' 优先级
您应该使用以下优先顺序来确定 'this' 关键字的上下文。
- 1. bind() 方法
- 2. call 和 apply() 方法
- 3. 对象方法
- 4. 全球范围