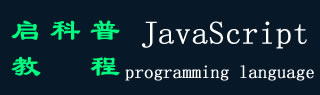
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - 函数 apply() 方法
函数 apply() 方法
JavaScript 中的 Function apply() 方法允许我们调用一个函数,给定一个特定的 this 值和作为数组提供的参数。
Function call() 和 apply() 方法非常相似,但它们之间的主要区别在于函数 apply() 方法接受包含所有函数参数的单个数组,而函数 call() 方法接受单个参数。
与 Function call() 方法相同,我们可以使用 apply() 方法来操作 this 值,并可以为 this 分配一个轨道对象。
语法
JavaScriot 中 Function apply() 方法的语法如下 -
func.apply(thisArg, [arg1, arg2, ... argN]);
参数
- thisArg − 'thisArg' grument 表示函数上下文。它是一个对象,其属性是使用 'this' 关键字访问引用函数所必需的。
- [arg1, arg2, ...argN] − 它们是要传递给函数的参数。
返回值
它返回从函数返回的结果值。
例子
让我们通过一些示例来了解 JavaScript 函数 apply() 方法。
在不传递参数的情况下使用 apply() 方法
在下面的代码中,我们定义了 test() 函数,该函数在输出中打印消息。
我们以标准方式调用函数,并使用 apply() 方法,而不传递任何参数来调用函数。输出显示 apply() 方法给出与普通函数调用相同的输出。
<html>
<head>
<title> JavaScript - Function apply() method </title>
</head>
<body>
<p id = "output1"> </p>
<p id = "output2"> </p>
<script>
function test() {
return "The function test is invoked!";
}
document.getElementById("output1").innerHTML = test();
document.getElementById("output2").innerHTML = test.apply();
</script>
</body>
</html>
输出
The function test is invoked!
仅使用带有此参数的 apply() 方法
在下面的代码中,'car' 对象包含三个不同的属性。我们将 car 对象作为 apply() 方法的 'thisArg' 参数传递。
在 showCar() 函数中,我们使用 'this' 关键字访问 car 对象的属性,并将其打印到输出中。
<html>
<head>
<title> JavaScript - Function apply() method </title>
</head>
<body>
<p id = "output"> </p>
<script>
function showCar() {
return "The price of the " + this.name + " " +
this.model + " is: " + this.price;
}
let car = {
name: "OD",
model: "Q6",
price: 10000000,
}
document.getElementById("output").innerHTML = showCar.apply(car);
</script>
</body>
</html>
输出
将 apply() 方法与函数参数数组一起使用
在下面的示例中,我们将 nums 对象作为 apply() 方法的第一个参数传递。之后,我们将参数数组作为 apply() 方法的参数传递。
在 printSum() 函数中,我们使用 'this' 关键字访问对象属性,使用函数参数访问函数参数。
<html>
<head>
<title> JavaScript - Function apply() method </title>
</head>
<body>
<p id = "output"> </p>
<script>
function printSum(p1, p2) {
return this.num1 + this.num2 + p1 + p2;
}
const nums = {
num1: 5,
num2: 10,
}
const ans = printSum.apply(nums, [40, 32]);
document.getElementById("output").innerHTML = "Total sum is " + ans;
</script>
</body>
</html>
输出
使用带有内置函数的 apply() 方法
您还可以将 apply() 方法与内置对象方法一起使用。我们可以使用 apply() 方法调用内置的对象方法(例如 Math.max 或 Math.min)。
在下面的示例中,我们使用带有内置 JavaScript 函数 Math.max 和 Math.min 的 apply() 方法。我们不能直接将这些方法用于数组。我们使用 apply() 方法调用 Math.max 和 Math.min 方法。我们将 null 作为 thisArg 传递,将 5 个整数的数组作为函数参数传递。
<html>
<head>
<title> JavaScript - Function apply() method </title>
</head>
<body>
<p id = "output-max"> Max element in the array: </p>
<p id = "output-min"> Max element in the array:</p>
<script>
const numbers = [7, 6, 4, 3, 9];
document.getElementById("output-max").innerHTML += Math.max.apply(null, numbers);
document.getElementById("output-min").innerHTML += Math.min.apply(null, numbers);
</script>
</body>
</html>
输出
Max element in the array:3
请注意,如果你直接对数组使用 Math.max() 或 Math.min() 方法来查找数组中的 max 或 min 元素,结果将是 NaN。