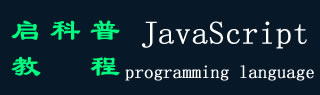
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - Abstraction(抽象)
JavaScript 中的Abstraction(抽象)
JavaScript 的抽象可以使用 abstract 类来实现。在面向对象的编程中,抽象概念允许您隐藏实现细节并仅向用户公开功能。
例如,您可以通过使用其名称访问方法,在 JavaScript 中执行 Math 对象方法,但无法查看其实现方式。以同样的方式执行 push()、pop() 等数组方法,但您不知道它是如何在内部实现的。
因此,抽象通过公开所需的功能并隐藏内部实现细节来使代码更简洁。
如何在 JavaScript 中实现抽象?
在大多数编程语言中,您可以使用 abstract 类实现抽象。抽象类仅包含方法声明,而不包含实现。此外,您需要将抽象类中声明的方法实现到子类中。此外,您不能创建抽象类的实例。
JavaScript 不允许原生创建像 Java 或 CPP 这样的抽象类,但你可以使用对象构造函数实现相同的功能。
首先,让我们使用下面的示例创建一个抽象类。
创建 Abstract 类
在下面的示例中,fruit() 函数初始化 name 属性。当任何人创建 fruit() 的实例时,constructor 属性的值将等于 'fruit'。因此,我们抛出一个错误来阻止创建 fruit 的实例。
此外,我们还将 getName() 方法添加到原型中。之后,我们创建一个 fruit() 构造函数的实例,你可以在输出中观察到错误。
<html>
<body>
<div id = "output"> </div>
<script>
try {
// Object constructor
function fruit() {
this.name = "Fruit";
if (this.constructor === fruit) {// Preventing the instance of the object
throw new Error("You can't create the instance of the fruit.");
}
}
// Implementing method in the prototype
fruit.prototype.getName = function () {
return this.name;
}
const apple = new fruit();
} catch (error) {
document.getElementById("output").innerHTML = error;
}
</script>
</body>
</html>
输出
在上面的示例中,您学习了如何实现抽象类功能。
现在,您将通过以下示例学习扩展抽象类并实现抽象类中定义的方法。
扩展 Abstract 类
在下面的示例中,fruit() 构造函数与上面的示例类似。我们已经实现了 Apple() 构造函数,初始化了 name 属性。
之后,我们使用 Object.create() 方法将 fruit() 函数的原型分配给 Apple() 函数。这意味着 Apple() 函数继承了 fruit() 类的属性和方法。
之后,我们创建了 Apple() 类的实例并调用了 getName() 方法。
<html>
<body>
<div id = "output">The name of the fruit is: </div>
<script>
// Abstract class
function fruit() {
this.name = "Fruit";
if (this.constructor === fruit) { // Preventing the instance of the object
throw new Error("You can't create the instance of the fruit.");
}
}
// Implementing method in the prototype
fruit.prototype.getName = function () {
return this.name;
}
// Child class
function Apple(fruitname) {
this.name = fruitname;
}
// Extending the Apple class with the fruit class
Apple.prototype = Object.create(fruit.prototype);
const apple = new Apple("Apple");
document.getElementById("output").innerHTML += apple.getName();
</script>
</body>
</html>
输出
原型在上面的代码中实现了 getName() 方法。所以,它是隐藏的。
这样,你可以使用对象构造函数在 JavaScript 中实现抽象。