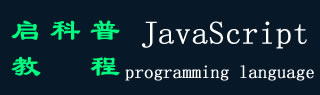
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - 类型转换
JavaScript 类型转换是指在 JavaScript 中将数据从一种数据类型转换为另一种数据类型的自动或显式过程。这些转换对于 JavaScript 有效地执行操作和比较至关重要。JavaScript 变量可以包含任何数据类型的值,因为它是一种弱类型语言。
JavaScript 中有两种类型的类型转换 -
- 隐式类型转换
- 显式类型转换
隐式类型转换也称为 强制 转换。
隐式类型转换
当 JavaScript 自动完成类型转换时,它称为隐式类型转换。例如,当我们在字符串和数字操作数中使用 '+' 运算符时,JavaScript 会将数字转换为字符串并将其与字符串连接。
下面是隐式类型转换的一些示例。
转换为 String (隐式转换)
在此示例中,我们使用 '+' 运算符将不同的值隐式转换为字符串数据类型。
"100" + 24; // 将24转换为字符串
'100' + false; // 将布尔值 false 转换为字符串
"100" + null; // 将空关键字转换为字符串
请注意,要使用 “+” 运算符将值转换为 string,一个操作数应该是 string。
让我们尝试下面的示例,并检查输出 -
<html>
<head>
<title>Implicit conversion to string </title>
</head>
<body>
<script>
document.write("100" + 24 + "<br/>");
document.write('100' + false + "<br/>");
document.write("100" + null+ "<br/>");
document.write("100" + undefined+ "<br/>");
</script>
</body>
</html>
转换为 Number (隐式转换)
当您将包含数字的字符串值与除 '+' 运算符之外的算术运算符一起使用时,它会自动将操作数转换为数字并执行算术运算,如以下示例所示。
布尔值也会转换为数字。
'100' / 50; // 将“100”转换为100
'100' - '50'; // 将“100”和“50”转换为100和50
'100' * true; // 将 true 转换为1
'100' - false; // 将 false 转换为0
'tp' / 50 // 将“tp”转换为NaN
尝试下面的示例并检查输出 -
<html>
<head>
<title> Implicit conversion to Number </title>
</head>
<body>
<script>
document.write(('100' / 50) + "<br>");
document.write(('100' - '50') + "<br>");
document.write(('100' * true) + "<br>");
document.write(('100' - false) + "<br>");
document.write(('tp' / 50) + "<br>");
</script>
</body>
</html>
转换为布尔值(隐式转换)
当您对任何变量使用 Nullish (!!) 运算符时,它会将其值隐式转换为布尔值。
num = !!0; // !0 = true, !!0 = false
num = !!1; // !1 = false, !!1 = true
str = !!""; // !"" = true, !!"" = false
str = !!"Hello"; // !"Hello" = false, !!"Hello" = true
Null 到 Number (隐式转换)
在 JavaScript 中,null 表示空。因此,当我们将 null 用作算术运算符的操作数时,它会自动转换为 0。
let num = 100 + null; // 将 null 转换为0
num = 100 * null; // 将null转换为0
未定义,带 Number 和 Boolean(隐式转换)
将 undefined 与 'number' 或 'boolean' 值一起使用总是在输出中给出 NaN。这里 NaN 的意思是不是一个数字。
<html>
<head>
<title> Using undefined with a number and boolean value </title>
</head>
<body>
<script>
let num = 100 + undefined; // Prints NaN
document.write("The value of the num is: " + num + "<br>");
num = false * undefined; // Prints NaN
document.write("The value of the num is: " + num + "<br>");
</script>
</body>
</html>
显式类型转换
在许多情况下,程序员需要手动转换变量的数据类型。这称为显式类型转换。
在 JavaScript 中,你可以使用构造函数或内置函数来转换变量的类型。
转换为 String(显式转换)
您可以使用 String() 构造函数将数字、布尔值或其他数据类型转换为字符串。
String(100); // 数字转换为字符串
String(null); // null 转换为字符串
String(true); // boolean 转换为 string
例
您可以使用 String() 构造函数将值转换为字符串。您还可以使用 typeof 运算符来检查结果值的 type。
<html>
<head>
<title> Converting to string explicitly </title>
</head>
<body>
<script>
document.write(typeof String(100) + "<br/>");
document.write(typeof String(null)+ "<br/>");
document.write(typeof String(true) + "<br/>");
</script>
</body>
</html>
我们还可以使用 Number 对象的 toString() 方法将 number 转换为 string。
const num = 100;
num.toString() // 将100转换为“100”
转换为 Number (显式转换)
您可以使用 Numer() 构造函数将字符串转换为数字。我们还可以使用一元加号 (+) 运算符将字符串转换为数字。
Number('100'); // 将 '100' 转换为 100
Number(false); // 将 false 转换为 0
Number(null); // 将 null 转换为 0
num = +"200"; // 使用一元运算符
但是,您也可以使用以下方法和变量将字符串转换为数字。
Sr.No. | 方法/运算符 | 描述 |
---|---|---|
1 | parseFloat() | 从字符串中提取浮点数。 |
2 | parseInt() | 从字符串中提取整数。 |
3 | + | 它是一个一元运算符。 |
例
您可以使用 Number() 构造函数或一元 (+) 运算符将字符串、布尔值或任何其他值转换为数字。
Number() 函数还将数字的指数表示法转换为十进制数。
<html>
<head>
<title> Converting to string explicitly </title>
</head>
<body>
<script>
document.write(Number("200") + "<br/>");
document.write(Number("1000e-2") + "<br/>");
document.write(Number(false) + "<br/>");
document.write(Number(null) + "<br/>");
document.write(Number(undefined) + "<br/>");
document.write(+"200" + "<br/>");
</script>
</body>
</html>
转换为布尔值(显式转换)
您可以使用 Boolean() 构造函数将其他数据类型转换为 Boolean。
Boolean(100); // 将数字转换为布尔值(true)
Boolean(0); // 0 是假值 (false)
Boolean(""); // 空字符串是假值(false)
Boolean("Hi"); // 将字符串转换为布尔值(true)
Boolean(null); // null 是假值(false)
例
您可以使用 Boolean() 构造函数将值转换为 Boolean。所有 false 值(如 0、空字符串、null、undefined 等)都转换为 false,其他值转换为 true。
<html>
<head>
<title> Converting to string explicitly </title>
</head>
<body>
<script>
document.write(Boolean(100) + "<br/>");
document.write(Boolean(0) + "<br/>");
document.write(Boolean("") + "<br/>");
document.write(Boolean("Hi") + "<br/>");
document.write(Boolean(null) + "<br/>");
</script>
</body>
</html>
将日期转换为字符串/数字
您可以使用 Date 对象的 Number() 构造函数或 getTime() 方法将日期字符串转换为数字。数字日期表示自 1970 年 1 月 1 日以来的总毫秒数。
按照以下语法将日期转换为数字。
Number(date);
OR
date.getTime();
您可以使用 String() 构造函数或 toString() 方法将日期转换为字符串。
按照以下语法将日期转换为字符串。
String(date);
OR
date.toString();
让我们尝试在程序的帮助下演示这一点。
<html>
<head>
<title> Coverting date to string / number </title>
</head>
<body>
<script>
let date = new Date();
let numberDate = date.getTime();
document.write("The Numeric date is: " + numberDate + "<br/>");
let dateString = date.toString();
document.write("The string date is: " + dateString + "<br/>");
</script>
</body>
</html>
JavaScript 中的换算表
在下表中,当我们将原始值转换为 string、number 和 boolean 时,我们给出了原始值及其结果值。
值 | 字符串转换 | 数字转换 | 布尔值转换 |
---|---|---|---|
0 | "0" | 0 | false |
1 | "1" | 1 | true |
"1" | "1" | 1 | true |
"0" | "0" | 0 | true |
"" | "" | 0 | false |
"Hello" | "Hello" | NaN | true |
true | "true" | 1 | true |
false | "false" | 0 | false |
null | "null" | 0 | false |
undefined | "undefined" | NaN | false |
[50] | "50" | 50 | true |
[50, 100] | "[50, 100]" | NaN | true |