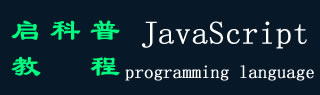
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript String at() 方法
JavaScript String at() 方法用于从指定位置的字符串中检索单个字符。它接受一个整数值,并返回一个包含单个 UTF-16 代码单元(用于 JavaScript 字符串的编码系统)的新字符串。
如果未找到给定的整数值 (index),则返回 'undefined'。
JavaScript 中的 “at()” 和 “charAt()” 方法彼此非常相似。但是,它们之间存在以下主要区别 -
- at() 是 JavaScript 的新成员,而 charAt() 已经存在了更长的时间。
- at() 方法更简洁,因为它可以读取和使用负索引,这与 charAt() 不同,后者不支持负索引,并在给定负索引时返回空字符串。
语法
以下是 JavaScript String at() 方法的语法 -
at(index)
参数
此方法接受一个名为 'index' 的参数,如下所述 -
- index − 要返回的字符串字符的索引(或位置)。
返回值
此方法返回一个包含单个字符的新字符串。
示例 1
检索给定字符串的第一个字符。
在给定的示例中,我们使用 JavaScript String at() 方法来检索给定字符串 “qikepucom” 的第一个字符。由于索引从 0 开始,到 str.length-1 结束,因此我们将索引值作为 0 传递以检索第一个字符。
<html>
<head>
<title>JavaScript String at() Method</title>
</head>
<body>
<script>
const str = "qikepucom";
document.write("String: ", str);
const index = 0;
document.write("<br>Index: ", index);
document.write("<br>The first character of string '", str, "' is: ", str.at(index));
</script>
</body>
</html>
输出
上面的程序将字符串 “qikepucom” 的第一个返回为 −
Index: 0
The first character of string 'qikepucom' is: q
示例 2
通过将负索引传递给 at() 方法来检索给定字符串的最后一个字符。
以下是 JavaScript String at() 方法的另一个示例。我们使用此方法检索给定字符串 “Hello World” 的最后一个字符。由于此方法可以使用和读取负索引,因此我们传递索引值 -1 以返回最后一个字符。
<html>
<head>
<title>JavaScript String at() Method</title>
</head>
<body>
<script>
const str = "Hello World";
document.write("String: ", str);
const index = -1;
document.write("<br>Index: ", index);
document.write("<br>The last character of string '", str, "' is: ", str.at(index));
</script>
</body>
</html>
输出
执行上述程序后,返回字符串 “Hello World” 的最后一个字符为 −
Index: -1
The last character of string 'Hello World' is: d
示例 3
比较 at() 和 charAt() 方法
在给定的示例中,我们比较了 “at()” 和 “charAt()” 方法,并尝试检索给定字符串 “JavaScript” 的倒数第二个元素。at() 方法处理负索引,因此索引值 -2 返回倒数第二个元素,而 charAt() 方法将索引值作为 str.length-2 返回倒数第二个元素。
<html>
<head>
<title>JavaScript String at() Method</title>
</head>
<body>
<script>
const str = "JavaScript";
document.write("String: ", str);
document.write("<br>The second last element using at(-2) method: ", str.at(-2));
document.write("<br>The second last element using charAt(str.length-2) method: ", str.charAt(str.length-2));
</script>
</body>
</html>
输出
以下输出显示了 at() 和 charAt() 方法之间的差异 -
The second last element using at(-2) method: p
The second last element using charAt(str.length-2) method: p