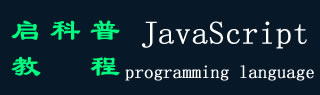
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - TypedArray lastIndexof() 方法
JavaScript TypedArray lastIndexOf() 方法从右到左在类型化数组中搜索指定的 searchElement。它返回 searchElement 最后一次出现的索引,如果未找到,则返回 -1。
假设指定的 searchElement 在类型化数组中只存在一次,则此方法返回从第 0 个索引开始的索引。如果 searchElement 多次出现,它将优先于最后一个元素。
语法
以下是 JavaScript TypedArraylastIndexof() 方法的语法 -
lastIndexOf(searchElement, fromIndex)
参数
此方法接受两个参数,即 'searchElement' 和 'fromIndex'。参数如下所述 -
- searchElement − 需要在类型化数组中搜索的元素。
- fromIndex − 从零开始的索引表示从末尾开始搜索的位置。
返回值
此方法返回类型化数组中 searchElement 的最后一个索引。如果 search 元素不存在,则返回 −1。
示例 1
如果省略 fromIndex 参数,并且只传递 searchElement 参数,则该方法返回指定 searchElement 的最后一个索引,该索引从 TypedArray 的末尾开始。
在下面的示例中,我们使用 JavaScript TypedArray 方法 lastIndexOf() 来检索类型化数组 [10, 20, 30, 40, 30, 50, 60] 中指定 searchElement 30 的最后一个索引。
<html>
<head>
<title>JavaScript TypedArray lastIndexof() Method</title>
</head>
<body>
<script>
const T_array = new Uint8Array([10, 20, 30, 30, 40, 50, 60]);
document.write("Original typed array: ", T_array);
const serachElement = 30;
document.write("<br>Search element: ", serachElement);
document.write("<br>The element ", serachElement, " found at the last index ", T_array.lastIndexOf(serachElement));
</script>
</body>
</html>
输出
上面的程序将元素 30 最后一次出现的索引返回为 3。
Search element: 30
The element 30 found at the last index 3
示例 2
如果我们将 'searchElement' 和 'fromIndex' 参数都传递给此方法,它将返回从指定的 fromIndex 开始的元素的最后一个索引。
以下是 JavaScript TypedArray lastIndexOf() 方法的另一个示例。我们使用此方法检索指定元素 6 的最后一个索引,从索引 5 开始搜索,在这个类型化的数组中:[1, 2, 4, 6, 8, 9, 6, 11]。
<html>
<head>
<title>JavaScript TypedArray lastIndexof() Method</title>
</head>
<body>
<script>
const T_array = new Uint8Array([1, 2 , 4, 6, 8, 9, 6, 11]);
document.write("Original typed array: ", T_array);
const serachElement = 6;
const fromIndex = 5;
document.write("<br>Search element: ", serachElement);
document.write("<br>From index: ", fromIndex);
document.write("<br>The element ", serachElement, " found at the last index ", T_array.lastIndexOf(serachElement, fromIndex));
</script>
</body>
</html>
输出
执行程序后,元素 6 的最后一次出现的索引返回为 3。
Search element: 6
From index: 5
The element 6 found at the last index 3
示例 3
如果在此类型化数组中找不到指定的 searchElement,则返回 -1。
在此示例中,我们在类型化数组上使用类型化数组 lastIndexOf() 方法检索给定类型化数组 [1, 3, 5, 7, 13] 中 searchElement 11 的最后一个索引。
<html>
<head>
<title>JavaScript TypedArray lastIndexof() Method</title>
</head>
<body>
<script>
const T_array = new Uint8Array( [1, 3, 5, 7, 13]);
document.write("Original typed array: ", T_array);
const serachElement = 6;
document.write("<br>Search element: ", serachElement);
document.write("<br>The element ", serachElement, " found at the last index ", T_array.lastIndexOf(serachElement));
</script>
</body>
</html>
输出
执行上述程序后,它将返回 -1。
Search element: 6
The element 6 found at the last index -1