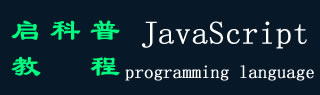
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - TypedArray findLastIndex() 方法
JavaScript TypedArray findLastIndex() 方法以相反的顺序迭代类型化数组,这意味着类型化数组的最后一个元素将被视为第一个元素,反之亦然。该方法返回满足提供的测试函数的第一个元素(从最后一个开始)的索引。如果类型化数组的元素都不满足 testing 函数,该方法将返回 '−1'。
以下是您应该了解的有关 'findLastIndex()' 方法的一些其他要点 -
- findLastIndex() 方法对 TypedArray(例如 Uint8Array、Int16Array 等)进行操作。
- 它接受 testing 函数作为参数。
- 对 TypedArray 中的每个元素执行 testing 函数。
- 如果元素满足 testing 函数指定的条件(它返回 true 值)。
- 如果没有元素满足,则返回测试函数 −1。
语法
以下是 JavaScript TypedArray findLastIndex() 方法的语法 -
findLastIndex(callbackFn, thisArg)
参数
此方法接受两个名为 'callbackFn' 和 'thisArg' 的参数,如下所述 -
- callbackFn − 此参数是一个测试函数,将针对 TypedArray 中的每个元素执行。此函数接受名为 'element'、'index' 和 'array' 的三个参数。以下是每个参数的描述 -
- element − 表示 TypedArray 中正在处理的当前元素。
- index − 指示 TypedArray 中当前元素的索引(位置)。
- array − 指整个 TypedArray。
- thisArg (可选) − 这是一个可选参数,允许您在 callbackFn 中指定 this 的值。
返回值
此方法返回满足 testing 函数的最后一个元素的索引。如果没有元素满足 testing 函数,则返回 '−1'。
示例 1
如果元素满足 testing 函数,它将返回类型化数组中最后一个元素的索引。
在下面的示例中,我们使用 JavaScript TypedArray findLastIndex() 方法来检索满足提供的测试函数的最后一个元素的索引。我们创建一个名为 isEven() 的测试函数,检查偶数,并将其作为参数传递给此方法。
<html>
<head>
<title>JavaScript TypedArray findLastIndex() Method</title>
</head>
<body>
<script>
function isEven(element, index, array){
return element %2 == 0;
}
const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80, 81]);
document.write("Typed array: ", T_array);
//calling function
document.write("<br>The index of the last even number is: ", T_array.findLastIndex(isEven));
</script>
</body>
</html>
输出
上面的程序返回最后一个偶数的索引为 7。
The index of the last even number is: 7
示例 2
在 TypedArray 中查找最后一个闰年的索引。
在下面给定的示例中,我们创建了一个名为 isLeapYear() 的函数,该函数检查此类型化数组 [2002, 2004, 2005, 2006, 2007, 2014] 中的闰年,并将此函数作为参数传递给 findLastIndex() 方法,以检索满足测试函数的最后一个闰年。
<html>
<head>
<title>JavaScript TypedArray findLastIndex() Method</title>
</head>
<body>
<script>
function isLeapYear(element, index, arrar){
return ((element % 4 == 0) && (element % 400 == 0 || element % 100 !=0));
}
const T_array = new Int16Array([2002, 2004, 2005, 2006, 2007, 2012]);
document.write("Typed array: ", T_array);
//calling function
document.write("<br>The index of the last leap year in typed array is: ", T_array.findLastIndex(isLeapYear));
</script>
</body>
</html>
输出
执行上述程序后,它将返回类型化数组中最后一个闰年的索引为 −
The index of the last leap year in typed array is: 5
示例 3
如果没有元素满足 testing 函数,findLastIndex() 方法将返回 '-1'。
以下是 JavaScript TypedArray findLastIndex() 方法的另一个示例。我们创建一个名为 isNegative() 的函数来检查负数,并将其作为参数传递给此方法,以检索满足测试函数的最后一个元素的索引。
<html>
<head>
<title>JavaScript TypedArray findLastIndex() Method</title>
</head>
<body>
<script>
function isNegative(element, index, array){
return element < 0;
}
const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]);
document.write("Typed array: ", T_array);
//calling function
document.write("<br>The index of last negative element: ", T_array.findLastIndex(isNegative));
</script>
</body>
</html>
输出
执行上述程序后,将返回 '-1'。
The index of last negative element: -1