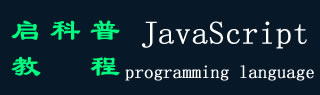
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript - TypedArray fill() 方法
JavaScript TypedArray fill() 方法用于在指定的索引范围(从开始到结束)内修改此类型化数组中的所有元素。我们需要提供一个元素(称为 value)来替换现有值。
start 和 end 参数是可选的,这意味着如果你没有将它们传递给此方法,它会通过用指定的值填充整个数组来修改整个数组。此方法修改原始数组,不返回新的类型化数组。
语法
以下是 JavaScript TypedArray fill() 方法的语法 -
fill(value, start, end)
参数
此方法接受名为 'value'、'start' 和 'end' 的三个参数。这些参数的解释如下 -
- value − 要在类型化数组中填充的值。
- start (可选)- 从零开始填充值的索引起始位置。
- end (可选)- 停止填充的从 0 开始的索引结束位置。
返回值
此方法返回修改后的类型化数组,该数组填充了指定值。
示例 1
如果我们省略 'start' 和 'end' 参数,只将 'value' 参数传递给此方法,它会更改并使用指定值填充整个类型化数组。
在下面的程序中,我们使用 JavaScript TypedArray fill() 方法更改整个类型化数组 [0, 0, 0, 0, 0] 并用指定的值 1 填充。
<html>
<head>
<title>JavaScript TypedArray fill() Method</title>
</head>
<body>
<script>
const T_array = new Uint8Array([0, 0, 0, 0, 0]);
document.write("The typed array before filled: ", T_array);
const value = 1;
document.write("<br>The value to be filled: ", value)
//using fill() method
T_array.fill(value);
document.write("<br>The typed array after filled: ", T_array);
</script>
</body>
</html>
输出
上面的程序返回一个修改后的类型化数组,其中填充了值 1 为 [1, 1, 1, 1, 1]。
The value to be filled: 1
The typed array after filled: 1,1,1,1,1
示例 2
如果我们传递 'value' 和 'start' 参数,此方法将用指定值填充指定起始位置的键入数组。
以下是 JavaScript TypedArray fill() 方法的另一个示例。我们使用此方法更改这个类型化数组 [1, 2, 3, 4, 5, 6, 7, 8],并在起始位置 2 处用指定的值 0 填充它。
<html>
<head>
<title>JavaScript TypedArray fill() Method</title>
</head>
<body>
<script>
const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]);
document.write("The typed array before filled: ", T_array);
const value = 0;
const start = 2;
document.write("<br>The value to be filled: ", value);
document.write("<br>The starting position: ", start);
//using fill() method
T_array.fill(value, start);
document.write("<br>The typed array after filled: ", T_array);
</script>
</body>
</html>
输出
执行上述程序后,它将返回一个修改后的类型化数组,从位置 2 开始填充值 0 为 −
The value to be filled: 0
The starting position: 2
The typed array after filled: 1,2,0,0,0,0,0,0
示例 3
如果所有三个参数 'value'、'start' 和 'end' 都被传递了,则 fill() 方法通过在 [start, end] 范围内填充指定值来更改类型化数组。
在这个程序中,我们使用 fill() 方法通过填充指定值 100 来更改这个类型化数组 [10, 20, 30, 40, 50, 60, 70, 80],指定范围 [2, 5]。
<html>
<head>
<title>JavaScript TypedArray fill() Method</title>
</head>
<body>
<script>
const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80]);
document.write("The typed array before filled: ", T_array);
const value = 100;
const start = 2;
const end = 5;
document.write("<br>The value to be filled: ", value);
document.write("<br>The starting position: ", start);
document.write("<br>The end position: ", end);
//using fill() method
T_array.fill(value, start, end);
document.write("<br>The typed array after filled: ", T_array);
</script>
</body>
</html>
输出
执行上述程序后,它将返回一个修改后的类型化数组 [10,20,100,100,100,60,70,80]。
The value to be filled: 100
The starting position: 2
The end position: 5
The typed array after filled: 10,20,100,100,100,60,70,80