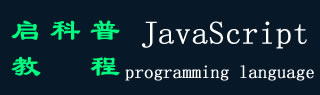
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript String padEnd() 方法
JavaScript String padEnd() 方法使用指定的 padString 填充(或扩展)当前字符串以达到给定的长度。填充将添加到当前字符串的末尾。如有必要,可以重复多次,如果我们没有为此方法指定此可选参数 padString,它会在此字符串的末尾添加空格作为填充以达到指定的长度。
填充字符串时,将字符添加到字符串的末尾,直到它达到特定长度。
语法
以下是 JavaScript String padEnd() 方法的语法 -
padEnd(targetLength, padString)
参数
此方法接受两个名为 “targetLength” 和 “padString” 的参数,如下所述 -
- targetLength − 新字符串的长度。
- padString − 用于填充(扩展)当前字符串的字符串。
返回值
此方法返回指定 targetLength 的新字符串,并在末尾附加 padString。
示例 1
如果省略 padString 参数,它将通过在当前字符串的末尾添加空格作为填充来扩展字符串,直到它达到指定的 targetLength。
在以下示例中,我们使用 JavaScript String padEnd() 方法填充(扩展)字符串,并在此字符串 “QikepuCom” 的末尾填充(扩展)带空格的字符串,直到它达到指定的 targetLength 30。
<html>
<head>
<title>JavaScript String padEnd() Method</title>
</head>
<body>
<script>
const str = "QikepuCom";
document.write("原始字符串: ", str);
new_str = "";
document.write("<br>添加填充之前的新字符串长度: ",new_str.length);
const targetLength = 30;
document.write("<br>目标长度: ", targetLength);
// 使用padEnd()方法
new_str = str.padEnd(targetLength);
document.write("<br>将填充后的新字符串添加到此字符串的末尾: ", new_str);
document.write("<br>添加填充后的新字符串长度: ",new_str.length);
</script>
</body>
</html>
输出
上面的程序返回一个新的字符串填充,字符串 “QikepuCom” 的末尾有空格。
添加填充之前的新字符串长度: 0
目标长度: 30
将填充后的新字符串添加到此字符串的末尾: QikepuCom
添加填充后的新字符串长度: 30
示例 2
如果 padString 和 targetLength 参数都传递给此方法,它会通过在末尾添加 padString 来扩展字符串,直到它达到一定长度。
以下是 JavaScript String padEnd() 方法的另一个示例。我们使用此方法在字符串末尾添加指定的 padString “#” 来扩展此字符串 “Hello World”,直到它达到指定的 targetLength 15。
<html>
<head>
<title>JavaScript String padEnd() Method</title>
</head>
<body>
<script>
const str = "Hello World";
document.write("原始字符串: ", str);
new_str = "";
document.write("<br>添加填充之前的新字符串长度: ",new_str.length);
const targetLength = 15;
const padString = "#";
document.write("<br>目标长度: ", targetLength);
document.write("<br>Pad 字符串: ", padString);
// 使用padEnd()方法
new_str = str.padEnd(targetLength, padString);
document.write("<br>将填充后的新字符串添加到此字符串的末尾: ", new_str);
document.write("<br>添加填充后的新字符串长度: ",new_str.length);
</script>
</body>
</html>
输出
执行上述程序后,它将返回一个末尾添加填充的新字符串。
添加填充之前的新字符串长度: 0
目标长度: 15
Pad 字符串: #
将填充后的新字符串添加到此字符串的末尾: Hello World####
添加填充后的新字符串长度: 15