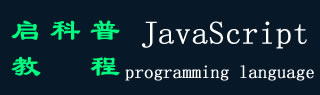
- JavaScript 教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript handler.construct() 方法
JavaScript 中的 handler.construct() 方法与 Proxy 对象结合使用,它允许您为对象上的基本操作定义自定义行为。当您将 Proxy 对象用作构造函数时,将调用此方法。在 Proxy 对象的帮助下,您可以封装另一个对象并使用它来拦截和修改其操作。
Handler.construct() 允许你修改有关代理对象实例如何构建的所有内容,包括添加更多初始化逻辑、添加日志记录,甚至完全更改行为。
语法
以下是 JavaScript handler.construct() 方法的语法 -
const p = new Proxy(target, {
construct: function(target, argumentsList, newTarget) {}
});
参数
- target − 它保存目标对象。
- argumentsList − 构造函数的 this 参数。
- newTarget - 它包含最初调用的构造函数。
返回值
此方法返回一个对象。
示例 1
让我们看一下以下示例,我们将在创建实际实例之前记录类的新实例的创建。
<html>
<style>
body {
font-family: verdana;
color: #DE3163;
}
</style>
<body>
<script>
const examp = {
construct(x, y) {
document.write(`Creating new instance of ${x.name}`);
return new x(...y);
}
};
class tp {
constructor(name) {
this.name = name;
}
}
const a = new Proxy(tp, examp);
const b = new a('Welcome');
</script>
</body>
</html>
输出
如果我们执行上述程序,它将在网页上显示文本。
示例 2
考虑另一个场景,我们将检查是否只创建了类的一个实例,并在后续调用时返回相同的实例。
<html>
<style>
body {
font-family: verdana;
color: #DE3163;
}
</style>
<body>
<script>
const examp = {
construct(x, y) {
if (!x.instance) {
x.instance = new x(...y);
}
return x.instance;
}
};
class tp {}
const a = new Proxy(tp, examp);
const x1 = new a();
const x2 = new a();
document.write(x1 === x2);
</script>
</body>
</html>
输出
在执行上述脚本时,它将在网页上显示文本。
示例 3
在以下示例中,我们将向构造函数添加其他 logic。
<html>
<style>
body {
font-family: verdana;
color: #DE3163;
}
</style>
<body>
<script>
function bike(x, y) {
this.x = x;
this.y = y;
this.fullName = function() {
return this.x + ' ' + this.y;
};
}
const examp = {
construct(a, b) {
const z = new a(...b);
z.year = new Date().getFullYear();
return z;
}
};
const c = new Proxy(bike, examp);
const d = new c("Vespa", "Bullet");
document.write(d.fullName() + " < br > ");
document.write(d.year);
</script>
</body>
</html>
当我们执行上述脚本时,会弹出输出窗口,在网页上显示文本。