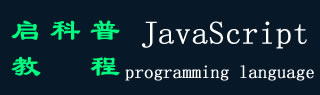
- JavaScript 菜鸟教程
- JavaScript 教程
- Javascript - 概述
- Javascript - 功能
- 在浏览器中启用 JavaScript
- JavaScript - 放置在 HTML 文件中
- JavaScript - 语法
- JavaScript - Hello World 程序
- JavaScript - Console.log()方法
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - Nullish 合并运算符
- JavaScript - Delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - Spread 运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else 语句
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in 循环
- JavaScript - For...of 循环
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case 语句
- JavaScript - 用户定义的迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自调用函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - 函数 call() 方法
- JavaScript - 函数 apply() 方法
- JavaScript - 函数 bind() 方法
- JavaScript - 闭包
- JavaScript - 变量范围
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number 对象
- JavaScript - 布尔对象
- JavaScript - String 对象
- JavaScript - Array 对象
- JavaScript - 日期对象
- JavaScript - DataView 对象
- JavaScript - 处理程序
- JavaScript - math 对象
- JavaScript - 正则表达式
- JavaScript - Symbol 对象
- JavaScript - Set(集)对象
- JavaScript - WeakSet 对象
- JavaScript - Maps (地图) 对象
- JavaScript - WeakMap 对象
- JavaScript - Iterables 对象
- JavaScript - Reflect 对象
- JavaScript - TypedArray 对象
- JavaScript - 模板文本
- JavaScript - tagged 模板
- 面向对象的 JavaScript
- JavaScript - 对象概述
- JavaScript - 类(Classes)
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - Static(静态)方法
- JavaScript - display(显示)对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - Encapsulation(封装)
- JavaScript - Inheritance(继承)
- JavaScript - Abstraction(抽象)
- JavaScript - Polymorphism(多态性)
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链接
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxies(代理)
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - history API( 历史 API)
- JavaScript - Storage API(存储 API)
- JavaScript - Forms API(表单 API)
- JavaScript - Worker API
- JavaScript - Fetch API (获取 API)
- JavaScript - Geolocation API (地理位置 API)
- JavaScript 事件
- JavaScript - Events (事件简介)
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委派
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误和异常处理
- JavaScript - try...catch 语句
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键词
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - 文档对象模型或 DOM
- JavaScript - DOM 方法
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM 节点列表
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象 (原子对象)
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 表单验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - Image Map(图像映射)
- JavaScript - 浏览器兼容性
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt 数据类型
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅层复制
- JavaScript - 调用堆栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - Currying (局部套用)
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - Debouncing (防抖)
- JavaScript - 性能
- JavaScript - 样式指南
- JavaScript - 内置函数
JavaScript DataView setBigUint64() 方法
JavaScript DataView setBigUint64() 方法接受一个大整数,并将其存储为 64 位无符号整数,从 DataView 中的指定字节偏移量开始,开始 8 字节段。
没有严格的对齐要求;您可以在 DataView 边界内的任何偏移量处存储多个字节。
如果 byteOffset 参数的值超出此范围,则此方法将引发 'RangeError' 异常,如果给定值不适合 bigInt 无符号整数,则将引发 'TypeError' 异常。
语法
以下是 JavaScript DataView setBigUint64() 方法的语法 -
setBigUint64(byteOffset, value, littleEndian)
参数
此方法接受名为 'byteOffset'、'value' 和 'littleEndian' 的三个参数,如下所述 -
- byteOffset - DataView 中将存储字节的位置。
- value − 需要存储的无符号 64 位大整数。
- littleEndian − 它指示数据是以 little-endian 还是 big-endian 格式存储。
返回值
此方法返回 'undefined',因为它只存储字节值。
示例 1
以下是 JavaScript DataView setBigUint64() 方法的基本示例。
<html>
<body>
<script>
const buffer = new ArrayBuffer(16);
const data_view = new DataView(buffer);
const byteOffset = 0;
//find the highest possible BigInt value that fits in an unsigned 64-bit integer
const value = 2n ** 64n - 1n;
document.write("The byte offset: ", byteOffset);
document.write("<br>Value: ", value);
//using the setBigUnit64() method
data_view.setBigUint64(byteOffset, value);
document.write("<br>The stored value: ", data_view.getBigUint64(byteOffset));
</script>
</body>
</html>
输出
上述程序将指定的 bigInt unsigned 值存储在当前 DataView 中,并将其显示为 -
The byte offset: 0
Value: 18446744073709551615
The stored value: 18446744073709551615
Value: 18446744073709551615
The stored value: 18446744073709551615
示例 2
如果你尝试打印此方法的结果,它将返回 'undefined' 作为输出。
<html>
<body>
<script>
const buffer = new ArrayBuffer(16);
const data_view = new DataView(buffer);
const byteOffset = 1;
//find the highest possible BigInt value that fits in an unsigned 64-bit integer
const value = 2n ** 64n - 1n;
document.write("The byte offset: ", byteOffset);
document.write("<br>Value: ", value);
//using the setBigUnit64() method
document.write("<br>The data_view.setBigUnit64() method returns: ", data_view.setBigUint64(byteOffset, value));
</script>
</body>
</html>
输出
执行上述程序后,它将返回 'undefined' 结果。
The byte offset: 1
Value: 18446744073709551615
The data_view.setBigUnit64() method returns: undefined
Value: 18446744073709551615
The data_view.setBigUnit64() method returns: undefined
示例 3
如果 byteOffset 参数的值超出此数据视图的边界,它将引发 'RangeError' 异常。
<html>
<body>
<script>
const buffer = new ArrayBuffer(16);
const data_view = new DataView(buffer);
const byteOffset = 0;
//find the highest possible BigInt value that fits in an unsigned 64-bit integer
const value = 23456543212;
document.write("The byte offset: ", byteOffset);
document.write("<br>Value: ", value);
try {
//using the setBigUnit64() method
data_view.setBigUint64(byteOffset, value);
} catch (error) {
document.write("<br>", error);
}
</script>
</body>
</html>
输出
执行上述程序后,它将引发 'RangeError' 异常。
The byte offset: 0
Value: 23456543212
TypeError: Cannot convert 23456543212 to a BigInt
Value: 23456543212
TypeError: Cannot convert 23456543212 to a BigInt
示例 4
如果给定的值不适合 bigInt 无符号整数,此方法将引发 'TypeError' 异常。
<html>
<head>
<title>JavaScript DataView setBigUint64() Method</title>
</head>
<body>
<script>
const buffer = new ArrayBuffer(16);
const data_view = new DataView(buffer);
const byteOffset = 0;
//find the highest possible BigInt value that fits in an unsigned 64-bit integer
const value = 23456543212;
document.write("The byte offset: ", byteOffset);
document.write("<br>Value: ", value);
try {
//using the setBigUnit64() method
data_view.setBigUint64(byteOffset, value);
} catch (error) {
document.write("<br>", error);
}
</script>
</body>
</html>
输出
上述程序将 'TypeError' 异常抛出为 −
The byte offset: 0
Value: 23456543212
TypeError: Cannot convert 23456543212 to a BigInt
Value: 23456543212
TypeError: Cannot convert 23456543212 to a BigInt