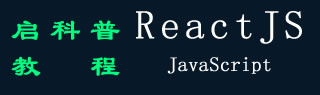
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - TransitionEvent 处理程序
TransitionEvent 处理程序方法类似于计算机程序中的特殊侦测器,用于监视网页上事物的外观(如颜色或大小)的变化。
假设我们在网站上有一个按钮,当我们点击它时,它很容易改变颜色。TransitionEvent 处理程序方法用于识别颜色更改的发生时间,并可用于在更改完成时执行操作。
简单来说,TransitionEvent 处理程序方法可以帮助我们的网站在视觉效果时做出反应。
语法
<div
onTransitionEnd={e => console.log('onTransitionEnd')}
/>
参数
- e - 这是一个React事件对象,我们可以与TransitionEvent属性一起使用。
TransitionEvent 的类型
有四种主要的过渡事件需要注意 -
类型 | 描述 |
---|---|
transitioncancel |
当动画或过渡在完成之前停止或取消时,将发生此事件。 |
transitionend |
当动画或过渡成功完成时,将发生此事件。 |
transitionrun |
当动画或过渡准备开始,但它可能尚未实际开始移动时,将发生此事件。 |
transitionstart |
当动画或过渡开始进行更改时,将发生此事件。 |
TransitionEvent 的属性
有一些过渡事件属性需要注意 -
属性 | 描述 |
---|---|
elapsedTime |
此属性告诉我们自过渡或动画开始以来已经过去了多少时间。 |
propertyName |
此属性指示在过渡或动画期间更改的 CSS 属性的名称。 |
pseudoElement |
它有助于识别动画是在元素上还是在用 CSS 添加的东西上。 |
例子
示例 - CSS 过渡应用程序
为了展示 TransitionEvent 处理程序方法的概念,我们可以创建一个组件来更改 CSS 过渡,并使用 transitionend 事件来识别过渡何时完成。因此,我们将创建一个按钮,该按钮通过过渡更改其背景颜色,并在过渡结束时显示一条消息。代码如下 -
import React, { useState } from "react";
function App() {
const [isTransition, setTransition] = useState(false);
const [message, setMessage] = useState("");
const handleButtonClick = () => {
setTransition(true);
setMessage("Transition...");
// delay and then reset the transition state
setTimeout(() => {
setTransition(false);
setMessage("Transition Complete");
}, 1000);
};
return (
<div className="App">
<button
className={`transition-button ${
isTransition ? "Transition" : ""
}`}
onClick={handleButtonClick}
>
Click me to start the transition
</button>
<div className="message">{message}</div>
</div>
);
}
export default App;
输出
此示例使用基本过渡来响应按钮单击,并使用 TransitionEvent 概念。当我们单击该按钮时,过渡开始,消息将在完成时通知我们。
示例 - 使用TransitionEvent的简单动画
此应用程序在单击时显示 div 元素的不透明度,并记录过渡持续时间。当我们单击“动画”按钮时,div 的不透明度将在 1 秒内从 1(完全不透明)变为 0(完全透明)。onTransitionEnd 处理程序记录完成转换所花费的时间。
import React, { useState } from "react";
function App() {
const [isAnimating, setIsAnimating] = useState(false);
const handleClick = () => {
setIsAnimating(true);
};
const handleTransitionEnd = (e) => {
console.log(`Transition completed: ${e.elapsedTime} seconds`);
setIsAnimating(false);
};
return (
<div>
<button onClick={handleClick}>Animate</button>
<div
style={{ opacity: isAnimating ? 0 : 1 }}
transition="opacity 1s ease"
onTransitionEnd={handleTransitionEnd}
>
Click me to animate!
</div>
</div>
);
}
export default App;
输出
示例 - 带有展开和折叠动画的手风琴
此应用程序显示一个手风琴,其内容面板在单击时会展开和折叠。单击面板标题可在 0.5 秒内平滑地展开或折叠其内容。onTransitionEnd 处理程序函数记录完成过渡的面板的标题。
import React, { useState } from "react";
const panels = [
{ title: "Panel 1", content: "This is the content of panel 1." },
{ title: "Panel 2", content: "This is the content of panel 2." },
{ title: "Panel 3", content: "This is the content of panel 3." },
];
function App() {
const [activePanelIndex, setActivePanelIndex] = useState(null);
const handleClick = (index) => {
setActivePanelIndex(index === activePanelIndex ? null : index);
};
const handleTransitionEnd = (e) => {
console.log(
`Panel transitioned: ${e.target.parentNode.querySelector(".panel-title").textContent}`
);
};
return (
<div>
{panels.map((panel, index) => (
<div key={index} className="panel">
<h3
className="panel-title"
onClick={() => handleClick(index)}
>
{panel.title}
</h3>
<div
className="panel-content"
style={{ maxHeight: activePanelIndex === index ? "500px" : "0" }}
transition="max-height 0.5s ease"
onTransitionEnd={handleTransitionEnd}
>
{panel.content}
</div>
</div>
))}
</div>
);
}
export default App;
输出
总结
TransitionEvent 处理函数是一种 Web 开发工具,用于识别网页上的过渡或动画并做出反应。它就像一个观察者,可以对某些 CSS 过渡事件做出反应。
这些方法允许开发人员向这些过渡事件添加自定义操作或响应,从而允许开发人员创建交互式和动态的 Web 应用程序。