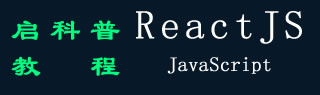
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - 门户
Portals 为组件提供了一种方法,可以将其子节点呈现到其自己的 DOM 层次结构之外的 DOM 节点中。Portal 可以在模型对话框、弹窗、工具提示等中使用,其中父节点(渲染组件)和子 DOM 节点(模型对话框)优先在不同的 DOM 节点中渲染。
在本章中,让我们了解门户是如何工作的,以及如何在我们的应用程序中应用它。
门户的概念和用法
让我们考虑一下,在主文档中有两个DOM节点,如下所示:
<div id='root'></div>
<div id='modalRoot'></div>
在这里,根 DOM 节点将与主 react 组件相连。每当 react 应用程序需要通过将模态对话框附加到 modelRoot DOM 节点而不是在其自己的 DOM 元素内渲染模型对话框来显示模态对话框时,它就会使用 modalRoot。
这将有助于将模态对话框与实际应用程序分开。将模态对话框与其父 DOM 元素分离将使其不受其父 DOM 元素的样式影响。样式可以单独应用,因为模式对话框、工具提示等在样式方面与其父项不同。
React 在 ReactDOM 包中提供了一个特殊的方法 createPortal 来创建门户。该方法的签名如下 -
ReactDOM.createPortal(child, container)
这里
- child 是由父组件呈现的模型对话框、工具提示等。
render() {
return ReactDOM.createPortal(
this.props.children, // modal dialog / tooltips
domNode // dom outside the component
);
}
container 是父 DOM 节点(上面示例中的 domNode)之外的 DOM 元素
应用门户
让我们创建一个新的 react 应用程序,以学习如何在本节中应用门户。
首先,创建一个新的 react 应用程序并使用以下命令启动它。
cd myapp
npm start
接下来,打开 App.css (src/App.css) 并删除所有 CSS 类,并为模态对话框添加 CSS。
.modal {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
display: grid;
justify-content: center;
align-items: center;
background-color: rgba(0,0,0,0.2);
}
.modalContent {
padding: 20px;
background-color: #fff;
border-radius: 2px;
display: inline-block;
min-height: 300px;
margin: 1rem;
position: relative;
min-width: 300px;
box-shadow: 0 3px 6px rgba(0,0,0,0.16), 0 3px 6px rgba(0,0,0,0.23);
justify-self: center;
}
接下来,打开 index.html (public/index.html) 并添加一个 DOM 节点以支持门户
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" />
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div style="padding: 10px;">
<div id="root"></div>
</div>
<div id="modalRoot"></div>
</body>
</html>
接下来,创建一个简单的组件 SimplePortal (src/Components/SimplePortal.js) 并渲染一个模态对话框,如下所示 -
import React from "react";
import PortalReactDOM from 'react-dom'
const modalRoot = document.getElementById('modalRoot')
class SimplePortal extends React.Component {
constructor(props) {
super(props);
}
render() {
return PortalReactDOM.createPortal(
<div
className="modal"
onClick={this.props.onClose}
>
<div className="modalContent">
{this.props.children}
<hr />
<button onClick={this.props.onClose}>Close</button>
</div>
</div>,
modalRoot,
)
}
}
export default SimplePortal;
这里
- createPortal 创建一个新的门户并呈现一个模态对话框。
- 模态对话框的内容是通过 this.props.children 从组件的子项中检索的
- 关闭按钮操作通过 props 处理,并由父组件处理。
接下来,打开 App 组件(src/App.js),并使用 SimplePortal 组件,如下图 -
import './App.css'
import React from 'react';
import SimplePortal from './Components/SimplePortal'
class App extends React.Component {
constructor(props) {
super(props);
this.state = { modal: false }
}
handleOpenModal = () => this.setState({ modal: true })
handleCloseModal = () => this.setState({ modal: false })
render() {
return (
<div className="container">
<div style={{ padding: "10px" }}>
<div>
<div><p>Main App</p></div>
<div>
<button onClick={this.handleOpenModal}>
Show Modal
</button>
{ this.state.modal ? (
<SimplePortal onClose={this.handleCloseModal}>
Hi, I am the modal dialog created using portal.
</SimplePortal>
) : null}
</div>
</div>
</div>
</div>
);
}
}
export default App;
这里
- 导入的 SimplePortal 组件
- 添加了一个按钮来打开模式对话框
- 创建了一个处理程序来打开模式对话框
- 创建了一个处理程序来关闭模态对话框,并通过 onClose 属性将其传递给 SimplePortal 组件。
最后,在浏览器中打开应用程序并检查最终结果。
总结
React 门户提供了一种简单的方法来访问和处理组件外部的 DOM。它使事件能够在不同的 DOM 节点之间冒泡,而无需任何额外的努力。