React 组件表示网页中的一小块用户界面。React 组件的主要工作是渲染其用户界面,并在其内部状态发生变化时更新它。除了呈现 UI 之外,它还管理属于其用户界面的事件。总而言之,React 组件提供了以下功能。
在 ReactJS 中使用组件
在本章中,让我们使用新创建的组件并增强我们的 ExpenseEntryItem 组件。
第 1 步 - 在您最喜欢的编辑器中打开我们的费用管理器应用程序并打开ExpenseEntryItem.js文件。
然后,使用以下语句导入 FormattedMoney 和 FormattedDate。
步骤2 - 接下来,通过包含FormattedMoney和FormattedDater组件来更新render方法。
在这里,我们通过组件的 value 属性传递了 amount 和 spendDate。
下面给出了 ExprenseEntryItem 组件的最终更新源代码 -
index.js
打开 index.js 并通过传递 item 对象来调用 ExpenseEntryItem 组件。
接下来,使用 npm 命令为应用程序提供服务。
npm start
打开浏览器,在地址栏中输入 http://localhost:3000,然后按回车键。
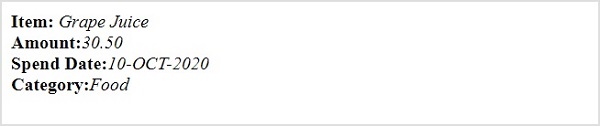