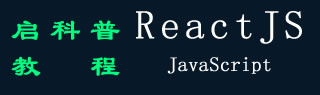
- ReactJS 教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - Material 用户界面
React 社区提供了大量的高级 UI 组件框架。Material UI 是流行的 React UI 框架之一。在本章中,让我们学习如何使用材质UI库。
安装
Material UI 可以使用 npm 包进行安装。
Material UI 建议使用 roboto 字体作为 UI。要使用 Roboto 字体,请使用 Gooogleapi 链接将其包含在内。
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" />
要使用字体图标,请使用googleapis的图标链接 -
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons" />
要使用SVG图标,请安装@material-ui/icons包 -
工作示例
让我们重新创建费用列表应用程序,并使用 Material UI 组件而不是 html 表格。
步骤1 - 首先,按照创建React应用程序一章中的说明,使用Create React App或Rollup打包器创建一个新的react应用程序react-materialui-app。
步骤2 - 安装React Transition Group库 -
在您最喜欢的编辑器中打开应用程序。
在应用程序的根目录下创建 src 文件夹。
在 src 文件夹下创建 components 文件夹。
创建一个文件,ExpenseEntryItemList.js src/components 文件夹中以创建 ExpenseEntryItemList 组件
导入 React 库和样式表。
import React from 'react';
步骤2 - 接下来,导入Material-UI库。
import Table from '@material-ui/core/Table';
import TableBody from '@material-ui/core/TableBody';
import TableCell from '@material-ui/core/TableCell';
import TableContainer from '@material-ui/core/TableContainer';
import TableHead from '@material-ui/core/TableHead';
import TableRow from '@material-ui/core/TableRow';
import Paper from '@material-ui/core/Paper';
创建 ExpenseEntryItemList 类并调用构造函数。
class ExpenseEntryItemList extends React.Component {
constructor(props) {
super(props);
}
}
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" />
创建一个 render() 函数。
render() {
}
在 render 方法中为表格行和表格单元格应用样式。
const StyledTableCell = withStyles((theme) => ({
head: {
backgroundColor: theme.palette.common.black,
color: theme.palette.common.white,
},
body: {
fontSize: 14,
},
}))(TableCell);
const StyledTableRow = withStyles((theme) => ({
root: {
'&:nth-of-type(odd)': {
backgroundColor: theme.palette.action.hover,
},
},
}))(TableRow);
使用 map 方法生成 Material UI StyledTableRow 的集合,每个集合代表列表中的单个费用分录项。
const lists = this.props.items.map((item) =>
<StyledTableRow key={item.id}>
<StyledTableCell component="th" scope="row">
{item.name}
</StyledTableCell>
<StyledTableCell align="right">{item.amount}</StyledTableCell>
<StyledTableCell align="right">
{new Date(item.spendDate).toDateString()}
</StyledTableCell>
<StyledTableCell align="right">{item.category}</StyledTableCell>
</StyledTableRow>
);
在这里,key 标识每一行,并且它在列表中必须是唯一的。
步骤3 - 在render()方法中,创建一个Material UI表,并在行部分包含列表表达式并返回它。
return (
<TableContainer component={Paper}>
<Table aria-label="customized table">
<TableHead>
<TableRow>
<StyledTableCell>Title</StyledTableCell>
<StyledTableCell align="right">Amount</StyledTableCell>
<StyledTableCell align="right">Spend date</StyledTableCell>
<StyledTableCell align="right">Category</StyledTableCell>
</TableRow>
</TableHead>
<TableBody>
{lists}
</TableBody>
</Table>
</TableContainer> );
最后,导出组件。
现在,我们已经成功创建了组件,以使用材料 ui 组件渲染费用项目。
该组件的完整源代码如下 -
import React from 'react';
import { withStyles } from '@material-ui/core/styles';
import Table from '@material-ui/core/Table';
import TableBody from '@material-ui/core/TableBody';
import TableCell from '@material-ui/core/TableCell';
import TableContainer from '@material-ui/core/TableContainer';
import TableHead from '@material-ui/core/TableHead';
import TableRow from '@material-ui/core/TableRow';
import Paper from '@material-ui/core/Paper';
class ExpenseEntryItemList extends React.Component {
constructor(props) {
super(props);
}
render() {
const StyledTableCell = withStyles((theme) => ({
head: {
backgroundColor: theme.palette.common.black,
color: theme.palette.common.white,
},
body: {
fontSize: 14,
},
}))(TableCell);
const StyledTableRow = withStyles((theme) => ({
root: {
'&:nth-of-type(odd)': {
backgroundColor: theme.palette.action.hover,
},
},
}))(TableRow);
const lists = this.props.items.map((item) =>
<StyledTableRow key={item.id}>
<StyledTableCell component="th" scope="row">
{item.name}
</StyledTableCell>
<StyledTableCell align="right">{item.amount}</StyledTableCell>
<StyledTableCell align="right">{new Date(item.spendDate).toDateString()}</StyledTableCell>
<StyledTableCell align="right">{item.category}</StyledTableCell>
</StyledTableRow>
);
return (
<TableContainer component={Paper}>
<Table aria-label="customized table">
<TableHead>
<TableRow>
<StyledTableCell>Title</StyledTableCell>
<StyledTableCell align="right">Amount</StyledTableCell>
<StyledTableCell align="right">Spend date</StyledTableCell>
<StyledTableCell align="right">Category</StyledTableCell>
</TableRow>
</TableHead>
<TableBody>
{lists}
</TableBody>
</Table>
</TableContainer> );
}
}
export default ExpenseEntryItemList;
index.js:
打开 index.js 并导入 react 库和我们新创建的 ExpenseEntryItemList 组件。
import React from 'react';
import ReactDOM from 'react-dom';
import ExpenseEntryItemList from './components/ExpenseEntryItemList';
声明一个列表(费用分录项目),并在文件中用一些随机值填充index.js。
const items = [
{ id: 1, name: "Pizza", amount: 80, spendDate: "2020-10-10", category: "Food" },
{ id: 1, name: "Grape Juice", amount: 30, spendDate: "2020-10-12", category: "Food" },
{ id: 1, name: "Cinema", amount: 210, spendDate: "2020-10-16", category: "Entertainment" },
{ id: 1, name: "Java Programming book", amount: 242, spendDate: "2020-10-15", category: "Academic" },
{ id: 1, name: "Mango Juice", amount: 35, spendDate: "2020-10-16", category: "Food" },
{ id: 1, name: "Dress", amount: 2000, spendDate: "2020-10-25", category: "Cloth" },
{ id: 1, name: "Tour", amount: 2555, spendDate: "2020-10-29", category: "Entertainment" },
{ id: 1, name: "Meals", amount: 300, spendDate: "2020-10-30", category: "Food" },
{ id: 1, name: "Mobile", amount: 3500, spendDate: "2020-11-02", category: "Gadgets" },
{ id: 1, name: "Exam Fees", amount: 1245, spendDate: "2020-11-04", category: "Academic" }
]
通过使用 ExpenseEntryItemList 组件,将项目传递给项目属性。
ReactDOM.render(
<React.StrictMode>
<ExpenseEntryItemList items={items} />
</React.StrictMode>,
document.getElementById('root')
);
index.js的完整代码如下:
import React from 'react';
import ReactDOM from 'react-dom';
import ExpenseEntryItemList from './components/ExpenseEntryItemList';
const items = [
{ id: 1, name: "Pizza", amount: 80, spendDate: "2020-10-10", category: "Food" },
{ id: 1, name: "Grape Juice", amount: 30, spendDate: "2020-10-12", category: "Food" },
{ id: 1, name: "Cinema", amount: 210, spendDate: "2020-10-16", category: "Entertainment" },
{ id: 1, name: "Java Programming book", amount: 242, spendDate: "2020-10-15", category: "Academic" },
{ id: 1, name: "Mango Juice", amount: 35, spendDate: "2020-10-16", category: "Food" },
{ id: 1, name: "Dress", amount: 2000, spendDate: "2020-10-25", category: "Cloth" },
{ id: 1, name: "Tour", amount: 2555, spendDate: "2020-10-29", category: "Entertainment" },
{ id: 1, name: "Meals", amount: 300, spendDate: "2020-10-30", category: "Food" },
{ id: 1, name: "Mobile", amount: 3500, spendDate: "2020-11-02", category: "Gadgets" },
{ id: 1, name: "Exam Fees", amount: 1245, spendDate: "2020-11-04", category: "Academic" }
]
ReactDOM.render(
<React.StrictMode>
<ExpenseEntryItemList items={items} />
</React.StrictMode>,
document.getElementById('root')
);
使用 npm 命令为应用程序提供服务。
index.html
打开公用文件夹中index.html文件,并包含材质 UI 字体和图标。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Material UI App</title>
<link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" />
<link rel="stylesheet" href="https://fonts.googleapis.com/icon?family=Material+Icons" />
</head>
<body>
<div id="root"></div>
<script type="text/JavaScript" src="./index.js"></script>
</body>
</html>
打开浏览器,在地址栏中输入 http://localhost:3000,然后 按 Enter 键。
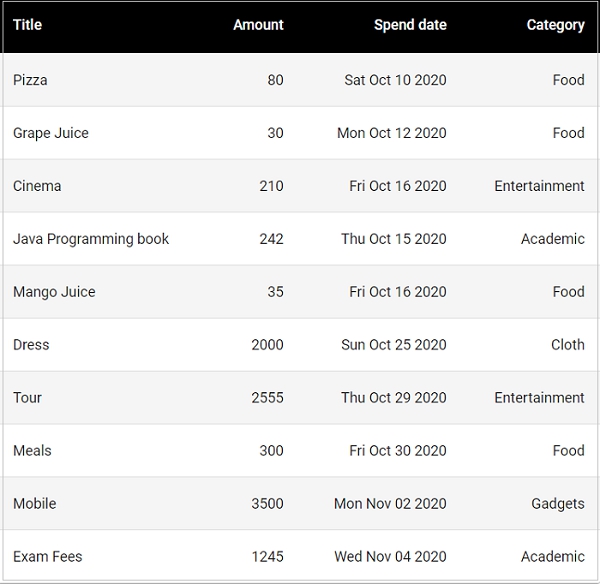