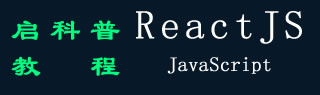
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - TouchEvent 处理程序
触摸事件对于增强用户体验非常重要,尤其是对于智能手机和平板电脑等触摸敏感设备。我们将了解几个触摸事件、它们的类型以及如何在 React 应用程序中处理它们。
触摸事件是更大的 Web 开发 UI 事件系列的一部分。通过这些事件,我们可以记录和响应用户活动,例如点击、滑动和捏合。
语法
<div
onTouchStart={e => console.log('onTouchStart')}
onTouchMove={e => console.log('onTouchMove')}
onTouchEnd={e => console.log('onTouchEnd')}
onTouchCancel={e => console.log('onTouchCancel')}
/>
参数
- e − 这是一个React事件对象,我们可以与TouchEvent属性一起使用。
TouchEvent 的类型
有四种主要类型的触摸事件需要注意 -
类型 | 描述 |
---|---|
touchstart |
当用户将手指放在触摸表面上时,将触发此事件。 |
touchend |
当用户将手指从表面上移开或触摸点离开表面边缘时,将发生触摸端事件。 |
touchmove |
当用户在表面上移动手指时,就会发生这种情况。它持续监控接触点的运动。 |
touchcancel |
当触摸点以某种方式受到干扰时,将发送此消息。 |
TouchEvent 的属性
有一些触摸事件属性需要注意 -
属性 | 描述 |
---|---|
altKey |
显示在事件发生时是否按下了 Alt 键。 |
ctrlKey |
指示在事件期间是否单击了 Ctrl 键。 |
changedTouches |
显示已更改的触摸的 Touch 对象列表。 |
getModifierState(key) |
一个函数,返回一个布尔值,显示是否按下了修饰键(例如,Shift、Alt 或 Ctrl)。 |
metaKey |
显示在事件期间是否按下了 Meta 键。 |
shiftKey |
指示在事件期间是否单击了 Shift 键。 |
touches |
表示触摸表面上所有当前触摸的 Touch 对象列表。 |
targetTouches |
显示对目标元素的触摸的 Touch 对象列表。 |
detail |
显示其他特定于事件的信息的整数值。 |
view |
引用生成事件的 Window 对象。 |
例子
示例 - 简单的触摸应用程序
我们将创建一个简单的 React 应用程序来使用以下代码显示 TouchEvent 处理程序。这是一个小型的 React 应用程序,它在控制台中显示触摸事件 -
import React from "react";
class App extends React.Component {
render() {
return (
<div
onTouchStart={(e) => console.log("onTouchStart")}
onTouchMove={(e) => console.log("onTouchMove")}
onTouchEnd={(e) => console.log("onTouchEnd")}
onTouchCancel={(e) => console.log("onTouchCancel")}
style={{
width: "200px",
height: "200px",
backgroundColor: "lightblue",
textAlign: "center",
lineHeight: "200px"
}}
>
TouchEvent
</div>
);
}
}
export default App;
输出
示例 - 长按应用程序
给定的 React 应用程序名为“LongPressApp”,旨在检测支持触摸的设备上的长按。该应用程序有一个浅蓝色背景的 div 元素,它的宽度和高度都跨度为 200 像素。当我们触摸并按住该应用程序至少 1000 毫秒时,它会向控制台记录一条消息,指出“检测到长按”。
import React from 'react';
class App extends React.Component {
handleTouchStart = () => {
this.longPressTimer = setTimeout(() => {
console.log('Long press detected');
}, 1000); // Adjust the duration for long press threshold
};
handleTouchEnd = () => {
clearTimeout(this.longPressTimer);
};
render() {
return (
<div
onTouchStart={this.handleTouchStart}
onTouchEnd={this.handleTouchEnd}
style={{
width: '200px',
height: '200px',
backgroundColor: 'lightblue',
textAlign: 'center',
lineHeight: '200px',
}}
>
LongPressApp
</div>
);
}
}
export default App;
输出
示例 - 捏合缩放应用程序
Pinch Zoom React 应用程序旨在检测捏合手势的开始和结束,通常用于在支持触摸的设备上进行缩放。该应用程序有一个浅蓝色背景的 div 元素,宽度和高度均为 200 像素。当用户开始捏合手势时,它会将消息“捏合开始”记录到控制台。同样,当用户结束捏合手势时,它会记录消息“捏合结束”。
import React from 'react';
class App extends React.Component {
handleTouchStart = (e) => {
if (e.touches.length === 2) {
console.log('Pinch started');
}
};
handleTouchEnd = (e) => {
if (e.touches.length < 2) {
console.log('Pinch ended');
}
};
render() {
return (
<div
onTouchStart={this.handleTouchStart}
onTouchEnd={this.handleTouchEnd}
style={{
width: '200px',
height: '200px',
backgroundColor: 'lightblue',
textAlign: 'center',
lineHeight: '200px',
}}
>
PinchZoomApp
</div>
);
}
}
export default App;
输出
总结
触摸事件在 React 应用程序中发挥着重要作用,用于创建引人入胜的交互式用户界面,尤其是在触摸敏感设备上。了解各种触摸事件以及如何管理它们,使我们能够为网站或应用程序用户提供轻松的用户体验。