Python random.paretovariate() 方法生成遵循帕累托分布的随机数。它是一种幂律概率分布,也称为“80-20 规则”。
这种分布通常用于社会科学、质量控制、金融和自然现象。它取决于名为 alpha 的形状参数,该参数决定了分布的行为。
这个函数不能直接访问,所以我们需要导入 random 模块,然后我们需要使用 random 静态对象调用这个函数。
语法
以下是 paretovariate() 方法的语法 -
random.paretovariate(alpha)
参数
此方法接受单个参数 -
- alpha:这是 Pareto 分布的形状参数。
返回值
此方法返回遵循 Pareto 分布且具有指定 alpha 的随机数。
示例 1
让我们看一个使用 Python random.paretovariate() 方法生成单个随机数的基本示例。
import random
# alpha for the Pareto distribution
alpha_ = 2
# Generate a random number from the Pareto distribution
random_value = random.paretovariate(alpha_)
print("Random value from Pareto distribution:", random_value)
以下是输出 -
Random value from Pareto distribution: 1.101299278142964
注意:由于程序的随机性,每次运行程序时生成的 Output 都会有所不同。
示例 2
下面是一个示例,它使用 random.paretovariate() 方法生成并显示一个直方图,该直方图显示形状参数为 3 的 Pareto 分布中样本的频率分布。
import random
import numpy as np
import matplotlib.pyplot as plt
# Generate 10000 samples from an Pareto distribution with rate parameter of 100
alpha = 3
num_samples = 10000
# Generate Pareto data
d = [random.paretovariate(alpha) for _ in range(num_samples)]
# Create a histogram of the data with bins from 0 to 500
h, b = np.histogram(d, bins=500)
# Plot the histogram
plt.figure(figsize=(7, 4))
plt.bar(b[:-1], h, width=1, edgecolor='none')
plt.title('Histogram of the Pareto Distributed Data')
plt.show()
上述代码的输出如下 -
示例 3
此示例生成并绘制不同 alpha 值的 Pareto 分布数据,以使用 random.paretovariate() 方法显示分布如何随 shape 参数的变化。
import random
import numpy as np
import matplotlib.pyplot as plt
def plot_pareto(alpha, order):
# Generate Pareto data
data = [random.paretovariate(alpha) for _ in range(500)]
# Plot histogram of the generated data
h, b = np.histogram(data, bins=np.arange(0, max(data)+1))
plt.bar(b[:-1], h, width=1, edgecolor='none', alpha=0.7, label=r'($\alpha={}$)'.format(alpha), zorder=order)
# Create a figure for the plots
fig = plt.figure(figsize=(7, 4))
# Plotting for each set of parameters
plot_pareto(3, 3)
plot_pareto(2, 2)
plot_pareto(1, 1)
# Adding labels and title
plt.title('Pareto Distributions for Different Alpha Values')
plt.legend()
plt.ylim(1, 150)
plt.xlim(1, 30)
# Show plot
plt.show()
在执行上述代码时,您将获得如下所示的类似输出 -
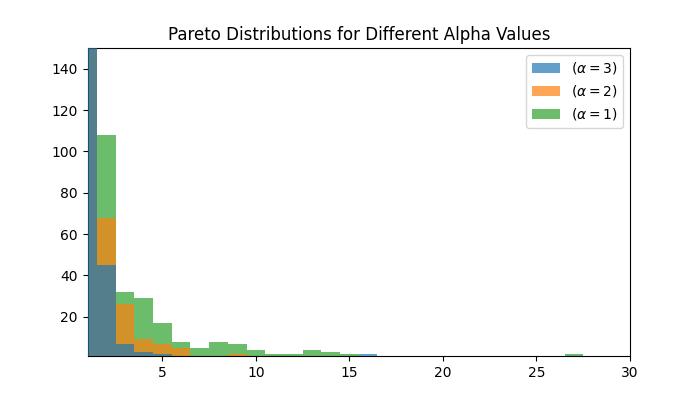