在 Python 中发送电子邮件
您可以使用多个库在 Python 中发送电子邮件,但最常见的是 smtplib 和 email。
Python 中的 “smtplib” 模块定义了一个 SMTP 客户端会话对象,该对象可用于将邮件发送到任何具有 SMTP 或 ESMTP 侦听器守护程序的 Internet 计算机。电子邮件“包”是一个用于管理电子邮件消息的库,包括 MIME 和其他基于 RFC 2822 的消息文档。
Python smptlib 的SMTP() 函数
Python smtplib.SMTP() 函数用于创建 SMTP 客户端会话对象,该对象建立与 SMTP 服务器的连接。此连接允许您通过服务器发送电子邮件。
设置 SMTP 服务器
在发送电子邮件之前,您需要设置 SMTP 服务器。常见的 SMTP 服务器包括 Gmail、Yahoo 或其他邮件服务提供商提供的服务器。
创建 SMTP 对象
要发送电子邮件,您需要使用以下函数获取 SMTP 类的对象 -
以下是参数的详细信息 -
- host - 这是运行 SMTP 服务器的主机。您可以指定主机的 IP 地址或域名,例如 qikepu.com。这是一个可选参数。
- port − 如果您提供 host 参数,则需要指定一个端口,SMTP 服务器正在侦听该端口。通常此端口为 25。
- local_hostname − 如果您的 SMTP 服务器在本地计算机上运行,则只需指定 localhost 作为选项。
例
以下脚本连接到端口 25 上“smtp.example.com”处的 SMTP 服务器,可以选择识别并保护连接、登录(如果需要)、发送电子邮件,然后退出会话 -
Python smtpd 模块
Python smtpd 模块用于创建和管理简单的邮件传输协议 (SMTP) 服务器。此模块允许您设置一个 SMTP 服务器,该服务器可以接收和处理传入的电子邮件消息,这对于测试和调试应用程序中的电子邮件功能很有价值。
设置 SMTP 调试服务器
smtpd 模块预装了 Python,并包括一个本地 SMTP 调试服务器。此服务器可用于测试电子邮件功能,而无需实际将电子邮件发送到指定地址;相反,它会将电子邮件内容打印到控制台。
运行此本地服务器无需处理邮件加密或使用凭据登录电子邮件服务器。
启动 SMTP 调试服务器
您可以在命令提示符或终端中使用以下命令启动本地 SMTP 调试服务器 -
例
以下示例演示了如何使用 smtplib 功能以及本地 SMTP 调试服务器发送虚拟电子邮件 -
在此示例中 -
- From − 您输入发件人的电子邮件地址 (fromaddr)。
- To − 您输入收件人的电子邮件地址 (toaddrs),该地址可以是多个地址,用空格分隔。
- Message − 您输入消息内容,以 ^D (Unix) 或 ^Z (Windows) 结尾。
“smtplib” 的 sendmail() 方法使用指定的发件人、收件人和邮件内容将电子邮件发送到在端口“1025”的“localhost”上运行的本地 SMTP 调试服务器。
输出
运行程序时,控制台将输出程序与 SMTP 服务器之间的通信。同时,运行 SMTPD 服务器的终端会显示传入的消息内容,帮助您调试和验证邮件发送过程。
From: abc@xyz.com
To: xyz@abc.com
Enter message, end with ^D (Unix) or ^Z (Windows):
Hello World
^Z
控制台反映以下日志 -
reply: retcode (250); Msg: b'OK'
send: 'rcpt TO:<xyz@abc.com>\r\n'
reply: b'250 OK\r\n'
reply: retcode (250); Msg: b'OK'
send: 'data\r\n'
reply: b'354 End data with <CR><LF>.<CR><LF>\r\n'
reply: retcode (354); Msg: b'End data with <CR><LF>.<CR><LF>'
data: (354, b'End data with <CR><LF>.<CR><LF>')
send: b'From: abc@xyz.com\r\nTo: xyz@abc.com\r\n\r\nHello
World\r\n.\r\n'
reply: b'250 OK\r\n'
reply: retcode (250); Msg: b'OK'
data: (250, b'OK')
send: 'quit\r\n'
reply: b'221 Bye\r\n'
reply: retcode (221); Msg: b'Bye'
运行 SMTPD 服务器的终端显示此输出 -
b'From: abc@xyz.com'
b'To: xyz@abc.com'
b'X-Peer: ::1'
b''
b'Hello World'
------------ END MESSAGE ------------
使用 Python 发送 HTML 电子邮件
要使用 Python 发送 HTML 电子邮件,您可以使用 smtplib 库连接到 SMTP 服务器,并使用 email.mime 模块来适当地构建和格式化您的电子邮件内容。
构建 HTML 电子邮件
发送 HTML 电子邮件时,您需要指定某些标头并相应地构建消息内容,以确保收件人的电子邮件客户端能够识别它并将其呈现为 HTML。
例
以下是在 Python 中将 HTML 内容作为电子邮件发送的示例 -
以电子邮件形式发送附件
要在 Python 中发送电子邮件附件,您可以使用 smtplib 库连接到 SMTP 服务器,并使用 email.mime 模块来构建和格式化您的电子邮件内容,包括附件。
构建带有附件的电子邮件
发送带有附件的电子邮件时,您需要使用 MIME(多用途 Internet 邮件扩展)正确格式化电子邮件。这涉及将 Content-Type 标头设置为 multipart/mixed 以表示电子邮件同时包含文本和附件。电子邮件的每个部分(文本和附件)都由边界分隔。
例
以下是示例,该示例发送一封附件为 /tmp/test.txt 的电子邮件 -
使用 Gmail 的 SMTP 服务器发送电子邮件
要在 Python 中使用 Gmail 的 SMTP 服务器发送电子邮件,您需要使用“TLS”加密在端口“587”上设置与 smtp.gmail.com 的连接,使用您的 Gmail 凭据进行身份验证,使用 Python 的 smtplib 和 email.mime 库构建电子邮件,并使用 sendmail() 方法发送它。最后,使用 quit() 关闭 SMTP 连接。
例下面是一个示例脚本,演示如何使用 Gmail 的 SMTP 服务器发送电子邮件 -
在运行上述脚本之前,必须将发件人的 gmail 帐户配置为允许访问“less 保护应用程序”。请访问以下链接。
https://myaccount.google.com/lesssecureapps 将显示的切换按钮设置为 ON。
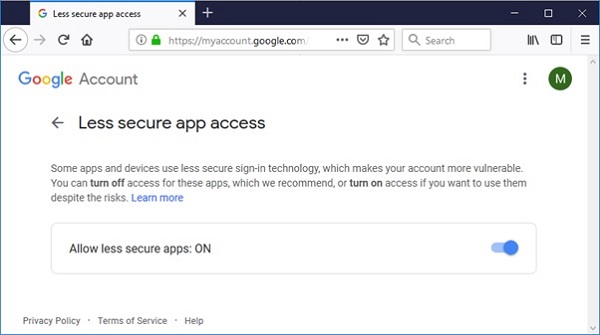
如果一切顺利,请执行上述脚本。邮件应投递到收件人的收件箱。