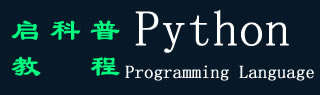
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - 更新元组
在 Python 中更新元组
在 Python 中,元组是一个不可变的序列,这意味着一旦创建了元组,就无法更改、添加或删除其元素。
要在 Python 中更新元组,您可以组合各种操作来创建新的元组。例如,您可以连接 Tuples、对 Tuples 进行切片或使用 Tuples 解包来实现所需的结果。这通常涉及将 Tuples 转换为 List,进行必要的修改,然后将其转换回 Tuples。
使用串联运算符更新元组
Python 中的串联运算符(用 + 表示)用于将两个序列(例如字符串、列表或元组)联接到一个序列中。当应用于元组时,串联运算符将连接两个(或多个)元组的元素,以创建一个包含两个元组中的所有元素的新元组。
我们可以通过创建将原始元组与其他元素组合在一起的新元组,从而使用串联运算符更新元组。
例
在下面的示例中,我们通过使用 “+” 运算符 − 将 “T1” 与 “T2” 连接起来来创建一个新元组
# Original tuple
T1 = (10, 20, 30, 40)
# Tuple to be concatenated
T2 = ('one', 'two', 'three', 'four')
# Updating the tuple using the concatenation operator
T1 = T1 + T2
print(T1)
它将产生以下输出 -
使用切片更新元组
Python 中的切片用于通过指定索引范围来提取序列的一部分(例如列表、元组或字符串)。切片的语法如下 -
sequence[start:stop:step]
哪里
- start 是切片开始的索引 (含)。
- stop 是切片结束的索引 (不包括)。
- step 是切片中元素之间的间隔(可选)。
我们可以通过创建一个新元组来使用切片来更新元组,该元组包含原始元组的切片和新元素。
例在此示例中,我们将元组分成两部分并在切片之间插入新元素来更新元组 -
# Original tuple
T1 = (37, 14, 95, 40)
# Elements to be added
new_elements = ('green', 'blue', 'red', 'pink')
# Extracting slices of the original tuple
# Elements before index 2
part1 = T1[:2]
# Elements from index 2 onward
part2 = T1[2:]
# Create a new tuple
updated_tuple = part1 + new_elements + part2
# Printing the updated tuple
print("Original Tuple:", T1)
print("Updated Tuple:", updated_tuple)
Original Tuple: (37, 14, 95, 40)
Updated Tuple: (37, 14, 'green', 'blue', 'red', 'pink', 95, 40)
以下是上述代码的输出 -
Updated Tuple: (37, 14, 'green', 'blue', 'red', 'pink', 95, 40)
使用列表推导式更新元组
Python 中的列表推导式是创建列表的一种简洁方法。它允许您通过将表达式应用于现有可迭代对象(如列表、元组或字符串)中的每个项目来生成新列表,并可选择包括用于筛选元素的条件。
由于元组是不可变的,因此更新元组涉及将其转换为列表,使用列表推导式进行所需的更改,然后将其转换回元组。
例在下面的示例中,我们首先将元组转换为列表,然后使用列表推导式将 100 添加到每个元素。然后,我们将列表转换回元组以获取更新的元组 -
# Original tuple
T1 = (10, 20, 30, 40)
# Converting the tuple to a list
list_T1 = list(T1)
# Using list comprehension
updated_list = [item + 100 for item in list_T1]
# Converting the updated list back to a tuple
updated_tuple = tuple(updated_list)
# Printing the updated tuple
print("Original Tuple:", T1)
print("Updated Tuple:", updated_tuple)
上述代码的输出如下 -
Updated Tuple: (110, 120, 130, 140)
使用 append() 函数更新元组
append() 函数用于将单个元素添加到列表的末尾。但是,由于 Tuples 是不可变的,因此 append() 函数不能直接用于更新 Tuples。
要使用 append() 函数更新元组,我们需要先将元组转换为列表,然后使用 append() 添加元素,最后将列表转换回元组。
例在下面的示例中,我们首先将原始元组 “T1” 转换为列表 “list_T1”。然后,我们使用循环迭代新元素,并使用 append() 函数将每个元素附加到列表中。最后,我们将更新后的列表转换回元组以获取更新的元组 -
# Original tuple
T1 = (10, 20, 30, 40)
# Convert tuple to list
list_T1 = list(T1)
# Elements to be added
new_elements = [50, 60, 70]
# Updating the list using append()
for element in new_elements:
list_T1.append(element)
# Converting list back to tuple
updated_tuple = tuple(list_T1)
# Printing the updated tuple
print("Original Tuple:", T1)
print("Updated Tuple:", updated_tuple)
我们得到的输出如下所示 -
Updated Tuple: (10, 20, 30, 40, 50, 60, 70)