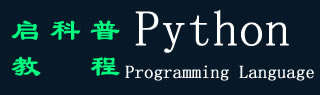
- Python 教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - 更改字典项
更改字典项
在 Python 中更改字典项是指修改与字典中特定键关联的值。这可能涉及更新现有键的值、添加新的键值对或从字典中删除键值对。
字典是可变的,这意味着其内容在创建后可以修改。
修改字典值
修改 Python 字典中的值是指更改与现有键关联的值。为此,您可以直接为该键分配新值。
例在以下示例中,我们将定义一个名为“person”的字典,其中包含键“name”、“age”和“city”及其相应的值。然后,我们将与键 'age' 关联的值修改为 26 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Modifying the value associated with the key 'age'
person['age'] = 26
print(person)
它将产生以下输出 -
更新多个字典值
如果需要一次更新字典中的多个值,可以使用 update() 方法。此方法用于使用来自另一个字典或键值对可迭代对象的元素更新字典。
update() 方法将提供的字典或 iterable 中的键值对添加到原始字典中,如果原始字典中已存在新值,则用新值覆盖任何现有键。
例在下面的示例中,我们使用 update() 方法来修改与 'persons' 字典中键 'age' 和 'city' 关联的值 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Updating multiple values
person.update({'age': 26, 'city': 'Los Angeles'})
print(person)
我们得到的输出如下所示 -
条件字典修改
Python 字典中的条件修改是指仅在满足特定条件时更改与键关联的值。
在修改 key 关联的值之前,您可以使用 if 语句检查某个条件是否为 true。
例在此示例中,如果 'persons' 字典中的当前值为 '25',我们将有条件地将与键 'age' 关联的值修改为 '26' -
# Initial dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Conditionally modifying the value associated with 'age'
if person['age'] == 25:
person['age'] = 26
print(person)
获得的输出如下所示 -
通过添加新的键值对来修改字典
向 Python 字典添加新的键值对是指将新键及其相应的值插入字典中。
此过程允许您根据需要包含其他信息,从而动态扩展存储在字典中的数据。
示例:使用赋值运算符
您可以通过直接为新键赋值来向字典添加新的键值对,如下所示。在下面的示例中,值为 'New York' 的键 'city' 被添加到 'person' 字典中 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25}
# Adding a new key-value pair 'city': 'New York'
person['city'] = 'New York'
print(person)
生成的结果如下 -
示例:使用 setdefault() 方法
如果键尚不存在,则可以使用 setdefault() 方法将新的键值对添加到字典中。
在此示例中,仅当键 'city' 尚不存在时,setdefault() 方法才会将值为 'New York' 的新键 'city' 添加到 'person' 字典中 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25}
# Adding a new key-value pair 'city': 'New York'
person.setdefault('city', 'New York')
print(person)
以下是上述代码的输出 -
通过删除键值对来修改字典
从 Python 字典中删除键值对是指从字典中删除特定键及其相应的值。
此过程允许您根据要消除的键有选择地从字典中删除数据。
示例:使用 del 语句
您可以使用 del 语句从字典中删除特定的键值对。在此示例中,del 语句从 'person' 字典中删除键 'age' 及其关联值 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Removing the key-value pair associated with the key 'age'
del person['age']
print(person)
上述代码的输出如下所示 -
示例:使用 pop() 方法
您还可以使用 pop() 方法从字典中删除特定的键值对,并返回与已删除的键关联的值。
在这里,pop() 方法从 'person' 字典中删除键 'age' 及其关联值 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Removing the key-value pair associated with the key 'age'
removed_age = person.pop('age')
print(person)
print("Removed age:", removed_age)
它将产生以下输出 -
Removed age: 25
示例:使用 popitem() 方法
您也可以使用 popitem() 方法从字典中删除最后一个键值对并将其作为 Tuples 返回。
现在,popitem() 方法从 'person' 字典中删除最后一个键值对,并将其作为元组返回 -
# Initial dictionary
person = {'name': 'Alice', 'age': 25, 'city': 'New York'}
# Removing the last key-value pair
removed_item = person.popitem()
print(person)
print("Removed item:", removed_item)
我们得到的输出如下所示 -
Removed item: ('city', 'New York')