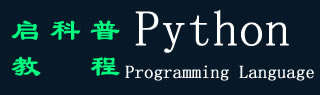
- Python 教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - 注释
Python 注释是 Python 源代码中程序员可读的解释或注释。添加它们的目的是使源代码更易于人类理解,并且被 Python 解释器忽略。注释增强了代码的可读性,并帮助程序员非常仔细地理解代码。
例如果我们执行下面给出的代码,生成的输出将简单地将 “Hello, World!” 打印到控制台,因为 Python 解释器会忽略注释,不会影响程序的执行 -
# This is a comment
print("Hello, World!")
Python 支持三种类型的注释,如下所示 -
- 单行注释
- 多行注释
- 文档字符串注释
Python 中的单行注释
Python 中的单行注释以井号 (#) 开头,并延伸到行尾。它们用于提供有关代码的简短说明或注释。它们可以放置在它们描述的代码上方的单独行上,也可以放在代码行的末尾(称为内联注释),以提供有关该特定行的上下文或说明。
示例:独立单行注释
独立的单行注释是单独占据一整行的注释,以哈希符号 (#) 开头。它位于它描述或注释的代码之上。
在此示例中,独立的单行注释位于“greet”函数“−
# Standalone single line comment is placed here
def greet():
print("Hello, World!")
greet()
示例:内联单行注释
内联单行注释是与一段代码出现在同一行的注释,位于代码后面,前面带有哈希符号 (#)。
在这里,内联单行注释遵循 print(“Hello, World!”) 语句 -
print("Hello, World!") # Inline single line comment is placed here
Python 中的多行注释
在 Python 中,多行注释用于提供跨多行的较长解释或注释。虽然 Python 没有用于多行注释的特定语法,但有两种常见的方法可以实现此目的:连续的单行注释和三引号字符串 -
连续单行注释
连续单行注释是指在每行的开头使用井号 (#)。此方法通常用于较长的解释或分割代码的某些部分。
例
在此示例中,使用多行注释来解释阶乘函数的用途和逻辑 -
# This function calculates the factorial of a number
# using an iterative approach. The factorial of a number
# n is the product of all positive integers less than or
# equal to n. For example, factorial(5) is 5*4*3*2*1 = 120.
def factorial(n):
if n < 0:
return "Factorial is not defined for negative numbers"
result = 1
for i in range(1, n + 1):
result *= i
return result
number = 5
print(f"The factorial of {number} is {factorial(number)}")
使用三引号字符串的多行注释
我们可以使用三引号字符串(''' 或 “”“) 来创建多行注释。这些字符串在技术上是字符串文本,但如果它们未分配给任何变量或在表达式中使用,则可以用作注释。
此模式通常用于块注释或记录需要详细说明的代码部分。
例
在这里,三引号字符串提供了 “gcd” 函数的详细说明,描述了其用途和使用的算法 -
"""
This function calculates the greatest common divisor (GCD)
of two numbers using the Euclidean algorithm. The GCD of
two numbers is the largest number that divides both of them
without leaving a remainder.
"""
def gcd(a, b):
while b:
a, b = b, a % b
return a
result = gcd(48, 18)
print("The GCD of 48 and 18 is:", result)
使用文档进行注释
在 Python 中,文档注释(也称为文档字符串)提供了一种将文档合并到代码中的方法。这对于解释模块、类、函数和方法的用途和用法非常有用。有效使用文档注释有助于其他开发人员理解您的代码及其用途,而无需阅读实现的所有详细信息。
Python 文档字符串
在 Python 中,文档字符串是一种特殊类型的注释,用于记录模块、类、函数和方法。它们使用三引号(''' 或 “”“)编写,并紧跟在它们记录的实体的定义之后。
文档字符串可以通过编程方式访问,这使它们成为 Python 内置文档工具不可或缺的一部分。
函数 Docstring 示例
def greet(name):
"""
This function greets the person whose name is passed as a parameter.
Parameters:
name (str): The name of the person to greet
Returns:
None
"""
print(f"Hello, {name}!")
greet("Alice")
访问文档字符串
可以使用 .__doc__ 属性或 help() 函数访问文档字符串。这样,您可以直接从交互式 Python shell 或在代码中轻松查看任何模块、类、函数或方法的文档。
示例:使用 .__doc__ 属性
def greet(name):
"""
This function greets the person whose name is passed as a parameter.
Parameters:
name (str): The name of the person to greet
Returns:
None
"""
print(greet.__doc__)
示例:使用 help() 函数
def greet(name):
"""
This function greets the person whose name is passed as a parameter.
Parameters:
name (str): The name of the person to greet
Returns:
None
"""
help(greet)