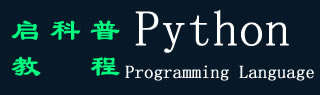
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - JSON
Python 中的 JSON
Python 中的 JSON 是一种流行的数据格式,用于系统之间的数据交换。json 模块提供了处理 JSON 数据的函数,允许您将 Python 对象序列化为 JSON 字符串,并将 JSON 字符串反序列化回 Python 对象。
JSON 序列化
JSON 序列化是将 Python 对象转换为 JSON 格式的过程。这对于以易于传输或存储的格式保存数据,并在以后重建回其原始形式非常有用。
Python 提供了 json 模块来处理 JSON 序列化和反序列化。我们可以在这个模块中使用 json.dumps() 方法进行序列化。
您可以将以下 Python 对象类型序列化为 JSON 字符串 -
- dict
- list
- tuple
- str
- int
- float
- bool
- None
例
以下是如何将 Python 字典序列化为 JSON 字符串的基本示例 -
import json
# Python dictionary
data = {"name": "Alice", "age": 30, "city": "New York"}
# Serialize to JSON string
json_string = json.dumps(data)
print(json_string)
它将产生以下输出 -
JSON 反序列化
JSON 反序列化是将 JSON 字符串转换回 Python 对象的过程。这对于读取和处理以 JSON 格式传输或存储的数据至关重要。
在 Python 中,我们可以使用 json.loads() 方法从字符串反序列化 JSON 数据,使用 json.load() 方法从文件中反序列化 JSON 数据。
示例:将 JSON 字符串反序列化为 Python 对象
在下面的示例中,我们使用 json.loads() 方法将 JSON 字符串反序列化为 Python 字典 -
import json
# JSON string
json_string = '{"name": "John", "age": 30, "is_student": false, "courses": ["Math", "Science"], "address": {"city": "New York", "state": "NY"}}'
# Deserialize JSON string to Python object
python_obj = json.loads(json_string)
print(python_obj)
以下是上述代码的输出 -
示例:从文件中反序列化 JSON
现在,要从文件中读取和反序列化 JSON 数据,我们使用 json.load() 方法 -
import json
# Read and deserialize from file
with open("data.json", "r") as f:
python_obj = json.load(f)
print(python_obj)
上述代码的输出如下 -
高级 JSON 处理
如果您的 JSON 数据包含需要特殊处理的对象(例如,自定义类),则可以定义自定义反序列化函数。使用 json.loads() 或 json.load() 方法的 object_hook 参数指定一个函数,该函数将与每个 JSON 对象解码的结果一起调用。
例在下面的示例中,我们将演示自定义对象序列化的用法 -
import json
from datetime import datetime
# Custom deserialization function
def custom_deserializer(dct):
if 'joined' in dct:
dct['joined'] = datetime.fromisoformat(dct['joined'])
return dct
# JSON string with datetime
json_string = '{"name": "John", "joined": "2021-05-17T10:15:00"}'
# Deserialize with custom function
python_obj = json.loads(json_string, object_hook=custom_deserializer)
print(python_obj)
我们得到的输出如下所示 -
JSONEncoder 类
Python 中的 JSONEncoder 类用于将 Python 数据结构编码为 JSON 格式。每种 Python 数据类型都转换为其相应的 JSON 类型,如下表所示 -
Python | JSON |
---|---|
Dict | object |
list, tuple | array |
Str | string |
int, float, int- & float-derived Enums | number |
True | true |
False | false |
None | null |
JSONEncoder 类使用 JSONEncoder() 构造函数进行实例化。此类中定义了以下重要方法 -
- encode(obj) - 将 Python 对象序列化为 JSON 格式的字符串。
- iterencode(obj) − 对对象进行编码并返回一个迭代器,该迭代器生成对象中每个项目的编码形式。
- indent − 确定编码字符串的缩进级别。
- sort_keys − 如果为 True,则键将按排序顺序显示。
- check_circular − 如果为 True,则检查容器类型对象中的循环引用。
在以下示例中,我们将对 Python 列表对象进行编码。我们使用 iterencode() 方法来显示编码字符串的每个部分 -
import json
data = ['Rakesh', {'marks': (50, 60, 70)}]
e = json.JSONEncoder()
# Using iterencode() method
for obj in e.iterencode(data):
print(obj)
它将产生以下输出 -
,
{
"marks"
:
[50
, 60
, 70
]
}
]
JSONDecoder 类
JSONDecoder 类用于将 JSON 字符串解码回 Python 数据结构。这个类中的主要方法是 decode()。
例在此示例中,“JSONEncoder”用于将 Python 列表编码为 JSON 字符串,然后使用“JSONDecoder”将 JSON 字符串解码回 Python 列表 -
import json
data = ['Rakesh', {'marks': (50, 60, 70)}]
e = json.JSONEncoder()
s = e.encode(data)
d = json.JSONDecoder()
obj = d.decode(s)
print(obj, type(obj))
获得的结果如下所示 -