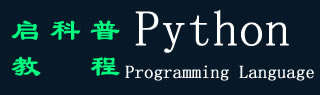
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - 文字
什么是 Python 文字?
Python 文字或常量是在源代码中表示固定值的表示法。与变量相反,文字(123、4.3、“Hello”)是静态值,或者你可以说是常量,它们在程序或应用程序的整个操作过程中不会改变。例如,在以下赋值语句中。
x = 10
这里 10 是一个表示 10 的数值,它直接存储在内存中。然而
y = x*2
在这里,即使表达式的计算结果为 20,它也不会真正包含在源代码中。您还可以使用内置的 int() 函数声明 int 对象。但是,这也是一种间接的实例化方式,而不是文字。
x = int(10)
Python 文字的类型
Python 提供了以下文本,本教程将对此进行解释:
- Python - Integer 文字
- Python - Float 文字
- Python - Complex 文字
- Python - String 文字
- Python - List 文字
- Python - Tuple 文字
- Python - Dictionary 文字
Python - Integer 文字
任何仅涉及数字符号(0 到 9)的表示都会创建一个 int 类型的对象。如此声明的对象可以由使用赋值运算符的变量引用。
示例:十进制文字
请看以下示例 -
x = 10
y = -25
z = 0
示例:八进制文字
Python 允许将整数表示为八进制数或十六进制数。在 Python 中,只有八位符号(0 到 7)但以 0o 或 0O 为前缀的数字表示是八进制数。
x = 0O34
示例:十六进制文本
同样,以 0x 或 0X 为前缀的一系列十六进制符号(0 到 9 和 a 到 f)在 Python 中以十六进制形式表示整数。
x = 0X1C
示例:将八进制和十六进制表示法演示为整数
但是,可能会注意到,即使您使用八进制或十六进制文字表示法,Python 也会在内部将它们视为 int 类型。
# Using Octal notation
x = 0O34
print ("0O34 in octal is", x, type(x))
# Using Hexadecimal notation
x = 0X1c
print ("0X1c in Hexadecimal is", x, type(x))
当您运行此代码时,它将产生以下输出 -
0X1c in Hexadecimal is 28 <class 'int'>
Python - Float 文字
浮点数由一个整数部分和一个小数部分组成。通常,小数点符号 (.) 在浮点数的文字表示中将这两个部分分隔开来。例如
示例:Float Literal
x = 25.55
y = 0.05
z = -12.2345
对于太大或太小的浮点数,其中小数点前或后的数字数较多,则使用科学记数法进行紧凑的文字表示。符号 E 或 e 后跟正整数或负整数,紧跟在整数部分之后。
示例:浮点科学记数法文字
例如,数字 1.23E05 等效于 123000.00。同样,1.23e-2 相当于 0.0123
# Using normal floating point notation
x = 1.23
print ("1.23 in normal float literal is", x, type(x))
# Using Scientific notation
x = 1.23E5
print ("1.23E5 in scientific notation is", x, type(x))
x = 1.23E-2
print ("1.23E-2 in scientific notation is", x, type(x))
在这里,您将获得以下输出 -
1.23E5 in scientific notation is 123000.0 <class 'float''>
1.23E-2 in scientific notation is 0.0123 <class 'float''>
Python - Complex 文字
Complex 由实数分量和虚数分量组成。虚部是任何数字(整数或浮点数)乘以“-1”的平方根
(√ −1)。在文字表示中 (−1−−−√−1) 是用“j”或“J”表示。因此,复数的文字表示采用 x+yj 的形式。
示例:Complex 类型文本
#Using literal notation of complex number
x = 2+3j
print ("2+3j complex literal is", x, type(x))
y = 2.5+4.6j
print ("2.5+4.6j complex literal is", x, type(x))
此代码将产生以下输出 -
2.5+4.6j complex literal is (2+3j) <class 'complex'>
Python - String 文字
String 对象是 Python 中的序列数据类型之一。它是 Unicode 码位的不可变序列。码位是根据 Unicode 标准对应于字符的数字。字符串是 Python 内置类“str”的对象。
字符串文字是通过将一系列字符括在单引号 ('你好')、双引号 (“你好”) 或三引号 ('''你好''' 或 “”你好“”“) 中来编写的。
示例:String Literal
var1='hello'
print ("'hello' in single quotes is:", var1, type(var1))
var2="hello"
print ('"hello" in double quotes is:', var1, type(var1))
var3='''hello'''
print ("''''hello'''' in triple quotes is:", var1, type(var1))
var4="""hello"""
print ('"""hello""" in triple quotes is:', var1, type(var1))
在这里,您将获得以下输出 -
"hello" in double quotes is: hello <class 'str'>
''''hello'''' in triple quotes is: hello <class 'str'>
"""hello""" in triple quotes is: hello <class 'str'>
示例:字符串内带双引号的字符串文本
如果需要将双引号嵌入到字符串的一部分,则应将字符串本身放在单引号中。另一方面,如果要嵌入单引号文本,则字符串应用双引号编写。
var1='Welcome to "Python qikepu" from qikepu.com'
print (var1)
var2="Welcome to 'Python qikepu' from qikepu.com"
print (var2)
它将产生以下输出 -
Welcome to 'Python Tutoriqikepual' from qikepu.com
Python - List 文字
Python 中的 List 对象是其他数据类型的对象的集合。List 是一个有序的集合 的物品不一定属于同一类型。集合中的单个对象通过从零开始的索引进行访问。
List 对象的文字表示是用一个或多个项目完成的,这些项目用逗号分隔并用方括号 [] 括起来。
示例:列表类型文本
L1=[1,"Ravi",75.50, True]
print (L1, type(L1))
它将产生以下输出 -
Python - Tuple 文字
Python 中的Tuple 对象是其他数据类型的对象的集合。Tuple 是项的有序集合,不一定是同一类型的。集合中的单个对象通过从零开始的索引进行访问。
Tuple 对象的文字表示是用一个或多个项完成的,这些项用逗号分隔并用括号 () 括起来。
示例:Tuple 类型文本
T1=(1,"Ravi",75.50, True)
print (T1, type(T1))
它将产生以下输出 -
示例:不带括号的 Tuple 类型文本
Python 序列的默认分隔符是括号,这意味着没有括号的逗号分隔序列也相当于Tuple的声明。
T1=1,"Ravi",75.50, True
print (T1, type(T1))
在这里,你也会得到相同的输出 -
Python - Dictionary 文字
与 List 或 Tuple 一样,Dictionary 也是一种集合数据类型。但是,它不是一个序列。它是项的无序集合,每个项都是一个键值对。值通过 “:” 符号绑定到键。将一个或多个用逗号分隔的键值对放在大括号内,以形成字典对象。
示例:Dictionary 类型文本
capitals={"USA":"New York", "France":"Paris", "Japan":"Tokyo",
"India":"New Delhi"}
numbers={1:"one", 2:"Two", 3:"three",4:"four"}
points={"p1":(10,10), "p2":(20,20)}
print (capitals, type(capitals))
print (numbers, type(numbers))
print (points, type(points))
键应该是一个不可变的对象。数字、字符串或 Tuple 可以用作键。键不能在一个集合中多次出现。如果一个键多次出现,则只会保留最后一个键。值可以是任何数据类型。可以将一个值分配给多个键。例如
staff={"Krishna":"Officer", "Rajesh":"Manager", "Ragini":"officer", "Anil":"Clerk", "Kavita":"Manager"}