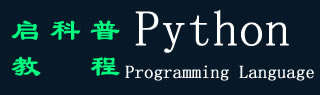
- Python 教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - 删除字典项
删除字典项
在 Python 中删除字典项是指从现有字典中删除键值对。字典是保存键对及其关联值的可变数据结构。每个键都充当唯一标识符,映射到字典中的特定值。
从字典中删除项目允许您从字典中消除不必要或不需要的数据,从而减小其大小并修改其内容。
我们可以使用各种方法删除 Python 中的字典项,例如 -
- 使用 del 关键字
- 使用 pop() 方法
- 使用 PopItem() 方法
- 使用 clear() 方法
- 使用字典推导式
使用 del 关键字删除字典项
Python 中的 del 关键字用于删除对象。在字典的上下文中,它用于根据指定的键从字典中删除一个项目或项目切片。
我们可以通过指定要删除的项目的键来使用 del 关键字删除字典项目。这将从字典中删除与指定键关联的键值对。
示例 1在以下示例中,我们将创建一个名为 numbers 的字典,其中包含整数键及其相应的字符串值。然后,使用 del 关键字删除键为 '20' 的项目 -
numbers = {10:"Ten", 20:"Twenty", 30:"Thirty",40:"Forty"}
print ("numbers dictionary before delete operation: \n", numbers)
del numbers[20]
print ("numbers dictionary before delete operation: \n", numbers)
它将产生以下输出 -
{10: 'Ten', 20: 'Twenty', 30: 'Thirty', 40: 'Forty'}
numbers dictionary before delete operation:
{10: 'Ten', 30: 'Thirty', 40: 'Forty'}
示例 2
del 关键字与 dictionary 对象一起使用时,会从内存中删除字典 -
numbers = {10:"Ten", 20:"Twenty", 30:"Thirty",40:"Forty"}
print ("numbers dictionary before delete operation: \n", numbers)
del numbers
print ("numbers dictionary before delete operation: \n", numbers)
以下是获得的输出 -
{10: 'Ten', 20: 'Twenty', 30: 'Thirty', 40: 'Forty'}
Traceback (most recent call last):
File "C:\Users\mlath\examples\main.py", line 5, in <module>
print ("numbers dictionary before delete operation: \n", numbers)
^^^^^^^
NameError: name 'numbers' is not defined
使用 pop() 方法删除字典项
Python 中的 pop() 方法用于从字典中删除指定的 key 并返回相应的值。如果未找到指定的键,则可以选择返回默认值,而不是引发 KeyError。
我们可以通过指定要删除的项目的键来使用 pop() 方法删除字典项。该方法将返回与指定键关联的值,并从字典中删除键值对。
例在此示例中,我们使用 pop() 方法从 'numbers' 字典中删除键为 '20' 的项目(将其值存储在 val 中)。然后我们检索更新的字典和弹出的值 -
numbers = {10:"Ten", 20:"Twenty", 30:"Thirty",40:"Forty"}
print ("numbers dictionary before pop operation: \n", numbers)
val = numbers.pop(20)
print ("nubvers dictionary after pop operation: \n", numbers)
print ("Value popped: ", val)
以下是上述代码的输出 -
{10: 'Ten', 20: 'Twenty', 30: 'Thirty', 40: 'Forty'}
nubvers dictionary after pop operation:
{10: 'Ten', 30: 'Thirty', 40: 'Forty'}
Value popped: Twenty
使用 popitem() 方法删除字典项
Python 中的 popitem() 方法用于从字典中删除并返回最后一个键值对。
我们可以通过调用字典上的 popitem() 方法来删除字典项,该方法会删除并返回添加到字典中的最后一个键值对。
例在下面的示例中,我们使用 popitem() 方法从字典 'numbers' 中删除任意项目(将其键值对都存储在 val 中),并检索更新的字典以及弹出的键值对 -
numbers = {10:"Ten", 20:"Twenty", 30:"Thirty",40:"Forty"}
print ("numbers dictionary before pop operation: \n", numbers)
val = numbers.popitem()
print ("numbers dictionary after pop operation: \n", numbers)
print ("Value popped: ", val)
上述代码的输出如下所示 -
{10: 'Ten', 20: 'Twenty', 30: 'Thirty', 40: 'Forty'}
numbers dictionary after pop operation:
{10: 'Ten', 20: 'Twenty', 30: 'Thirty'}
Value popped: (40, 'Forty')
使用 clear() 方法删除字典项
Python 中的 clear() 方法用于从字典中删除所有项目。它有效地清空了字典,使其长度为 0。
我们可以通过在 dictionary 对象上调用 clear() 方法来删除字典项。此方法从字典中删除所有键值对,从而有效地使其为空。
例在下面的示例中,我们使用 clear() 方法从字典 'numbers' 中删除所有项目 -
numbers = {10:"Ten", 20:"Twenty", 30:"Thirty",40:"Forty"}
print ("numbers dictionary before clear method: \n", numbers)
numbers.clear()
print ("numbers dictionary after clear method: \n", numbers)
我们得到的输出如下所示 -
{10: 'Ten', 20: 'Twenty', 30: 'Thirty', 40: 'Forty'}
numbers dictionary after clear method:
{}
使用字典推导式删除字典项
字典理解是在 Python 中创建字典的一种简洁方法。它遵循与 list comprehension 相同的语法,但生成的是字典而不是列表。使用字典推导式,您可以迭代可迭代对象(例如列表、元组或其他字典),将表达式应用于每个项目,并根据该表达式的结果构造键值对。
如果需要根据某些条件从字典中删除项目,通常使用其他方法,如 del、pop() 或 popitem()。这些方法允许您显式指定要从字典中删除的项目。
例在此示例中,我们根据要删除的预定义键列表从 'student_info' 字典中删除项目 'age' 和 'major' -
# Creating a dictionary
student_info = {
"name": "Alice",
"age": 21,
"major": "Computer Science"
}
# Removing items based on conditions
keys_to_remove = ["age", "major"]
for key in keys_to_remove:
student_info.pop(key, None)
print(student_info)
获得的输出如下所示 -