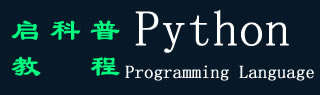
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - 文档字符串
Python 中的文档字符串
在 Python 中,文档字符串(docstrings)是一种记录模块、类、函数和方法的方法。它们用三引号 (""") 编写,并且可以跨越多行。
文档字符串是将文档与 Python 代码相关联的便捷方式。它们可以通过它们记录的相应 Python 对象的 __doc__ 属性进行访问。以下是编写文档字符串的不同方法 -
单行文档字符串
单行文档字符串用于简短的文档。它们提供了函数或方法作用的简要描述。单行文档字符串应适合三引号内的一行,并以句点结尾。
例在下面的示例中,我们使用单行文档字符串来编写文本 -
def add(a, b):
"""Return the sum of two numbers."""
return a + b
result = add(5, 3)
print("Sum:", result)
多行文档字符串
多行文档字符串用于更详细的文档。它们提供了更全面的描述,包括参数、返回值和其他相关详细信息。多行文档字符串以三引号开头和结尾,包括一个摘要行,后跟一个空行和一个更详细的描述。
例以下示例使用多行文档字符串作为代码的解释 -
def multiply(a, b):
"""
Multiply two numbers and return the result.
Parameters:
a (int or float): The first number.
b (int or float): The second number.
Returns:
int or float: The result of multiplying a and b.
"""
return a * b
result = multiply(5, 3)
print("Product:", result)
模块的文档字符串
为模块编写文档字符串时,请将文档字符串放在模块顶部,紧跟在任何 import 语句之后。模块文档字符串提供了模块功能的概述并列出了其主要组件,例如模块提供的函数、类和异常的列表。
例在此示例中,我们演示了在 Python 中对模块使用文档字符串 -
import os
"""
This module provides Utility functions for file handling operations.
Functions:
- 'read_file(filepath)': Reads and returns the contents of the file.
- 'write_file(filepath, content)': Writes content to the specified file.
Classes:
- 'FileNotFoundError': Raised when a file is not found.
Example usage:
>>> import file_utils
>>> content = file_utils.read_file("example.txt")
>>> print(content)
'Hello, world!'
>>> file_utils.write_file("output.txt", "This is a test.")
"""
print("This is os module")
类的文档字符串
类可以使用文档字符串来描述它们的用途和用法。类中的每个方法也可以有自己的文档字符串。类 docstring 应提供类及其方法的概述。
例在下面的示例中,我们展示了 Python 中类的文档字符串的使用 -
class Calculator:
"""
A simple calculator class to perform basic arithmetic operations.
Methods:
- add(a, b): Return the sum of two numbers.
- multiply(a, b): Return the product of two numbers.
"""
def add(self, a, b):
"""Return the sum of two numbers."""
return a + b
def multiply(self, a, b):
"""
Multiply two numbers and return the result.
Parameters:
a (int or float): The first number.
b (int or float): The second number.
Returns:
int or float: The result of multiplying a and b.
"""
return a * b
cal = Calculator()
print(cal.add(87, 98))
print(cal.multiply(87, 98))
访问文档字符串
Python 中的文档字符串是使用它们记录的对象的 __doc__ 属性来访问的。此属性包含与对象关联的文档字符串 (docstring),提供了一种访问和显示有关函数、类、模块或方法的用途和用法的信息的方法。
例在下面的示例中,我们定义了两个函数 “add” 和 “multiply”,每个函数都有一个描述其参数和返回值的文档字符串。然后我们使用 “__doc__” 属性来访问和打印这些文档字符串 -
# Define a function with a docstring
def add(a, b):
"""
Adds two numbers together.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
"""
return a + b
result = add(5, 3)
print("Sum:", result)
# Define another function with a docstring
def multiply(x, y):
"""
Multiplies two numbers together.
Parameters:
x (int): The first number.
y (int): The second number.
Returns:
int: The product of x and y.
"""
return x * y
result = multiply(4, 7)
print("Product:", result)
# Accessing the docstrings
print(add.__doc__)
print(multiply.__doc__)
编写文档字符串的最佳实践
以下是在 Python 中编写文档字符串的最佳实践 -
- 简洁明了 - 确保文档字符串清楚地解释了代码的用途和用法,避免了不必要的细节。
- 使用正确的语法和拼写 - 确保文档字符串编写良好,语法和拼写正确。
- 遵循约定 - 使用标准约定来格式化文档字符串,例如 Google 样式、NumPy 样式或 Sphinx 样式。
- 包括示例 - 在适用的情况下提供示例,以说明如何使用记录的代码。
Google 样式文档字符串
Google 风格的文档字符串提供了一种使用缩进和标题来记录 Python 代码的结构化方式。它们被设计为可读性和信息量大,遵循特定的格式。
例下面是一个带有 Google 样式文档字符串的函数示例 -
def divide(dividend, divisor):
"""
Divide two numbers and return the result.
Args:
dividend (float): The number to be divided.
divisor (float): The number to divide by.
Returns:
float: The result of the division.
Raises:
ValueError: If `divisor` is zero.
"""
if divisor == 0:
raise ValueError("Cannot divide by zero")
return dividend / divisor
result = divide(4, 7)
print("Division:", result)
NumPy/SciPy 样式文档字符串
NumPy/SciPy 风格的文档字符串在科学计算中很常见。它们包括 parameters、returns 和 examples 的部分。
例以下是具有 NumPy/SciPy 样式文档字符串的函数示例 -
def fibonacci(n):
"""
Compute the nth Fibonacci number.
Parameters
----------
n : int
The index of the Fibonacci number to compute.
Returns
-------
int
The nth Fibonacci number.
Examples
--------
>>> fibonacci(0)
0
>>> fibonacci(5)
5
>>> fibonacci(10)
55
"""
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
result = fibonacci(4)
print("Result:", result)
Sphinx 样式文档字符串
Sphinx 风格的文档字符串与 Sphinx 文档生成器兼容,并使用 reStructuredText 格式。
reStructuredText (reST) 是一种用于创建结构化文本文档的轻量级标记语言。Sphinx 文档生成器将 “reStructuredText” 文件作为输入,并生成各种格式的高质量文档,包括 HTML、PDF、ePub 等。
例以下是具有 Sphinx 样式文档字符串的函数示例 -
def divide(dividend, divisor):
"""
Divide two numbers and return the result.
Args:
dividend (float): The number to be divided.
divisor (float): The number to divide by.
Returns:
float: The result of the division.
Raises:
ValueError: If `divisor` is zero.
"""
if divisor == 0:
raise ValueError("Cannot divide by zero")
return dividend / divisor
result = divide(76, 37)
print("Result:", result)
Docstring 与 Comment
以下是 Python 文档字符串和注释之间突出显示的差异,分别关注它们的用途、格式、用法和可访问性 -
Docstring | Comment |
---|---|
用于记录 Python 对象,例如函数、类、方法、模块或包。 | 用于为人类读者注释代码、提供上下文或暂时禁用代码。 |
用三引号(“”“、”“”或“或”''''“)编写,并紧跟在对象的定义之后。 | 以 # 符号开头,并与带注释的代码放在同一行。 |
存储为对象的属性,可通过编程方式访问。 | 在执行过程中被 Python 解释器忽略,纯粹是为了人类理解。 |
使用对象的 __doc__ 属性访问。 | 无法以编程方式访问;仅存在于源代码中。 |