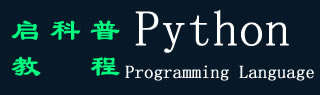
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python math.log() 方法
Python math.log() 方法用于计算正数值的自然对数(以 e 为底)。
对数的一般和简单定义是指数的倒数。这意味着,对数的结果是对数底数将提高到的幂。因此,此方法的结果始终是浮点值。
底数 'e' 的对数可以表示如下:
logba = p
哪里
- A:任意数字
- b:对数的底数
- p:基数升高到的功率
注意 − 这个函数不能直接访问,所以我们需要导入 math 模块,然后我们需要使用 math 静态对象调用这个函数。
语法
以下是 Python math.log() 方法的语法 -
math.log( x )
参数
- x − 这必须是一个正数值。
返回值
此方法返回 x 的自然对数,对于 x > 0。
例以下示例显示了 Python math.log() 方法的用法。我们在这里将正值作为参数传递给此方法,因此结果也将是一个正浮点值。
import math # This will import the math module
# Create a numeric object
x = 36.789
# Calculate the natural logarithm of x
ln = math.log(x)
# Display the log value
print("The logarithm of x is:", ln)
当我们运行上述程序时,它会产生以下结果——
The logarithm of x is: 3.6051988874479988
例
但是,如果我们将负值作为参数传递给此方法,则会引发 ValueError,因为自然对数的域仅为正实数。
import math # This will import the math module
# Create a numeric object
x = -76.43
# Calculate the natural logarithm of x
ln = math.log(x)
# Display the log value
print("The logarithm of x is:", ln)
如果我们编译并运行上面的程序,输出显示如下 -
Traceback (most recent call last):
File "main.py", line 7, in
ln = math.log(x)
ValueError: math domain error
File "main.py", line 7, in
ln = math.log(x)
ValueError: math domain error
例
该方法也不接受数值以外的参数。
在下面的示例中,让我们创建一个包含数值的列表,并将其作为参数传递给此方法。当 list 是 iterable 时,预计会引发 ValueError。
import math # This will import the math module
# Create a list object
x = [12, 45.68, 112, 66.33]
# Calculate the natural logarithm of x
ln = math.log(x)
# Display the log value
print("The logarithm of x is:", ln)
如果我们执行上面的程序,则会引发 ValueError ,如下所示 -
Traceback (most recent call last):
File "main.py", line 7, in
ln = math.log(x)
TypeError: must be real number, not list
File "main.py", line 7, in
ln = math.log(x)
TypeError: must be real number, not list
例
但是,我们可以使用 loop 语句来遍历上面示例中给出的列表,并找到其中包含的数值的自然对数。
import math # This will import the math module
# Create a list object
x = [12, 45.68, 112, 66.33]
# Using a for loop, iterate through the list
for n in range(0, len(x)):
# Calculate the natural logarithm of x
ln = math.log(x[n])
# Display the log value
print("The logarithm of x[",n,"] is:", ln)
在编译和执行上述程序时,结果如下 -
The logarithm of x[ 0 ] is: 2.4849066497880004
The logarithm of x[ 1 ] is: 3.821660565347755
The logarithm of x[ 2 ] is: 4.718498871295094
The logarithm of x[ 3 ] is: 4.194642283537465
The logarithm of x[ 1 ] is: 3.821660565347755
The logarithm of x[ 2 ] is: 4.718498871295094
The logarithm of x[ 3 ] is: 4.194642283537465
例
这个 log() 方法的工作方式与 exp() 方法完全相反。这意味着,log() 方法的参数是 exp() 方法的返回值。
在这个例子中,我们正在创建一个对象 'x' 并将其作为参数传递给 exp() 方法。从此方法获取的返回值存储在另一个变量 'var' 中。现在,如果存储在 'var' 中的值作为参数传递给 log() 方法,则返回值应为存储在 'x' 中的值。
import math # This will import the math module
# Create a list object
x = 12.34
# Pass x as an argument to the exp() method
var = math.exp(x)
print("The exponent value of x is:", var)
# Calculate the natural logarithm of x
ln = math.log(var)
# Display the log value
print("The logarithm of x is:", ln)
上述程序的输出显示如下 -
The exponent value of x is: 228661.9520568098
The logarithm of x is: 12.34
The logarithm of x is: 12.34