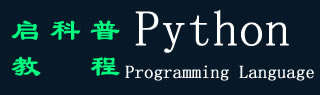
- Python 教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python math.ceil() 方法
Python math.ceil() 方法用于查找最接近的数值的较大整数。例如,浮点数 3.6 的 ceil 值为 4。
所涉及的过程几乎类似于估计或四舍五入技术。当 ceil 值 3.6 为 4 时出现差异,但 ceil 值 3.2 也将为 4;与估计技术不同,后者将 3.2 四舍五入为 3 而不是 4。
注意 − 这个函数不能直接访问,所以我们需要导入 math module,然后我们需要使用 math static object 调用这个函数。
语法
以下是 Python math.ceil() 方法的语法 -
math.ceil(x)
参数
- x − 这是一个数值对象。
返回值
此方法返回大于参数 x 的最小整数。
例以下示例显示了 Python math.ceil() 方法的用法。在这里,我们将创建两个具有正值和负值的数值对象,并且这两个对象的 ceil 值都是使用此方法计算的。
import math # This will import math module
# Create two numeric objects x and y
x = 45.17
y = -36.89
# Calculate and display the ceil value of x
res = math.ceil(x)
print("The ceil value of x:", res)
# Calculate and display the ceil value of y
res = math.ceil(y)
print("The ceil value of y:", res)
当我们运行上述程序时,它会产生以下结果——
The ceil value of x: 46
The ceil value of y: -36
The ceil value of y: -36
例
但是,此方法不会接受除数字对象以外的任何值作为其参数。
在这个例子中,让我们尝试将一个包含数字对象的列表作为参数传递给此方法,并检查它是否计算了所有对象的 ceil 值。
import math # This will import math module
# Create a list containing numeric objects
x = [12.36, 87.45, 66.33, 12.04]
# Calculate the ceil value for the list
res = math.ceil(x)
print("The ceil value of x:", res)
在执行上述程序时,将引发 TypeError ,如下所示 -
Traceback (most recent call last):
File "main.py", line 5, in
res = math.ceil(x)
TypeError: must be real number, not list
File "main.py", line 5, in
res = math.ceil(x)
TypeError: must be real number, not list
例
要使用此方法返回列表中数字对象的 ceil 值,可以使用 loop 语句。
我们正在创建一个包含 number 对象的列表,其 ceil 值将使用 ceil() 方法计算。然后,我们将使用 for 循环迭代此列表;对于每次迭代,列表中的 Number 对象将作为参数传递给此方法。由于列表中的对象都是数字,因此该方法不会引发错误。
import math # This will import math module
# Create a list containing numeric objects
x = [12.36, 87.45, 66.33, 12.04]
ln = len(x)
# Calculate the ceil value for the list
for n in range (0, ln):
res = math.ceil(x[n])
print("The ceil value of x[",n,"]:", res)
现在,如果我们执行上面的程序,结果将产生如下 -
The ceil value of x[ 0 ]: 13
The ceil value of x[ 1 ]: 88
The ceil value of x[ 2 ]: 67
The ceil value of x[ 3 ]: 13
The ceil value of x[ 1 ]: 88
The ceil value of x[ 2 ]: 67
The ceil value of x[ 3 ]: 13
例
我们可以在 Python 的许多实际应用中实现这个功能。例如,让我们尝试找到两个数字的商的 ceil 值。让我们首先创建两个数字对象,使用 “/” 运算符将它们除以,并使用 ceil() 方法找到它们的商的 ceil 值。
import math # This will import math module
# Create a list containing numeric objects
x = 54.13
y = 14.78
#Find the quotient of x and y
qt = x/y
print("The quotient of these values is:", qt)
# Calculate the ceil value for the quotient
res = math.ceil(qt)
print("The ceil value of x/y is:", res)
让我们编译并运行上面的程序,输出显示如下 -
The quotient of these values is: 3.6623815967523683
The ceil value of x/y is: 4
The ceil value of x/y is: 4