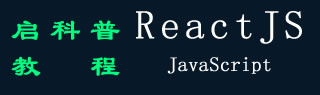
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - UNSAFE_componentWillUpdate() 方法
在 Web 开发中,UNSAFE_componentWillUpdate() 起到警告的作用。它发生在我们要对网站的一部分进行修改时。
请记住,此警告仅在我们更新任何内容时显示,而不是在我们首次创建它时显示。因此,UNSAFE_componentWillUpdate() 起到警告作用,帮助我们在更新网站时发现问题。
语法
UNSAFE_componentWillUpdate(nextProps, nextState)
参数
此方法接受两个参数:nextProps 和 nextState。
- nextProps - 这些是我们的 Web 组件将被赋予的新特性(如信息)。这就像获得一套新的指令一样。
- nextState - 这反映了我们组件的新状态。这类似于了解当前情况。
返回值
UNSAFE_componentWillUpdate() 不返回任何结果。它更像是进行更改之前的起点,因此它不会提供结果或答案。
例子
示例 1
在此示例中,我们将在 React 应用程序中使用 UNSAFE_componentWillUpdate() 函数来创建一个简单的示例。
我们将有一个基本的 React 组件,称为 App。它将让我们通过单击按钮来更改页面的背景颜色。UNSAFE_componentWillUpdate() 函数将检测背景颜色的变化并相应地调整页面的背景颜色。
import React, { Component } from 'react';
class App extends Component {
constructor(props) {
super(props);
this.state = {
backgroundColor: 'white',
};
}
// This is the UNSAFE_componentWillUpdate() function.
UNSAFE_componentWillUpdate(nextProps, nextState) {
if (nextState.backgroundColor !== this.state.backgroundColor) {
document.body.style.backgroundColor = nextState.backgroundColor;
}
}
handleChangeColor = (color) => {
this.setState({ backgroundColor: color });
}
render() {
return (
<div>
<h1>Color Change App</h1>
<button onClick={() => this.handleChangeColor('red')}>Change to Red</button>
<button onClick={() => this.handleChangeColor('blue')}>Change to Blue</button>
<button onClick={() => this.handleChangeColor('green')}>Change to Green</button>
</div>
);
}
}
export default App;
输出
示例 2
在此示例中,我们将使用 UNSAFE_componentWillUpdate() 函数将温度从摄氏度更新为华氏度。我们可以输入摄氏度或华氏度的温度,程序将立即调整另一个温度值。例如,如果我们输入以摄氏度为单位的温度,则应用程序将以华氏度显示可比温度,反之亦然。当我们输入新的温度时,它使用 UNSAFE_componentWillUpdate() 方法处理转换。
所以下面是相同的代码 -
// TempConverterApp.js
import React, { Component } from 'react';
import './App.css';
class TempConverterApp extends Component {
constructor(props) {
super(props);
this.state = {
celsius: 0,
fahrenheit: 32,
};
}
// Usage of UNSAFE_componentWillUpdate() to update the temperature values
UNSAFE_componentWillUpdate(nextProps, nextState) {
if (nextState.celsius !== this.state.celsius) {
// Convert Celsius to Fahrenheit
nextState.fahrenheit = (nextState.celsius * 9) / 5 + 32;
} else if (nextState.fahrenheit !== this.state.fahrenheit) {
// Convert Fahrenheit to Celsius
nextState.celsius = ((nextState.fahrenheit - 32) * 5) / 9;
}
}
handleChangeCelsius = (value) => {
this.setState({ celsius: value });
};
handleChangeFahrenheit = (value) => {
this.setState({ fahrenheit: value });
};
render() {
return (
<div className='App'>
<h1>Temperature Converter</h1>
<label>
Celsius:
<input
type="number"
value={this.state.celsius}
onChange={(e) => this.handleChangeCelsius(e.target.value)}
/>
</label>
<br />
<label>
Fahrenheit:
<input
type="number"
value={this.state.fahrenheit}
onChange={(e) => this.handleChangeFahrenheit(e.target.value)}
/>
</label>
</div>
);
}
}
export default TempConverterApp;
输出
正常:用于普通文本。
示例 3
这个应用程序是一个简单的计数器应用程序,可以倒计时。有两个按钮:一个用于增加计数,另一个用于减少计数。该程序还将告诉我们当前计数是偶数还是奇数。它通过调用 UNSAFE_componentWillUpdate() 方法,根据计数是偶数还是奇数来更新消息。如果我们有一个偶数,它将显示为“偶数”,如果我们有一个奇数,它将显示“奇数”。
所以这个应用程序的代码如下 -
// CounterApp.js
import React, { Component } from 'react';
import './App.css';
class CounterApp extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
message: '',
};
}
// Usage of UNSAFE_componentWillUpdate() to update the message based on the count value
UNSAFE_componentWillUpdate(nextProps, nextState) {
if (nextState.count !== this.state.count) {
nextState.message = nextState.count % 2 === 0 ? 'Even Count' : 'Odd Count';
}
}
handleIncrement = () => {
this.setState((prevState) => ({ count: prevState.count + 1 }));
};
handleDecrement = () => {
this.setState((prevState) => ({ count: prevState.count - 1 }));
};
render() {
return (
<div className='App'>
<h1>Counter App</h1>
<p>{this.state.message}</p>
<button onClick={this.handleIncrement}>Increment</button>
<button onClick={this.handleDecrement}>Decrement</button>
<p>Count: {this.state.count}</p>
</div>
);
}
}
export default CounterApp;
输出
注意
因此,我们可以在如上所示的输出图像中看到,有两个按钮可用:一个用于增量,另一个用于减少。并且根据偶数和奇数计数显示的消息。
总结
UNSAFE_componentWillUpdate() 是一个 React 函数,在组件更新和重新渲染之前不久使用。它让我们有机会为未来的变化做好准备。