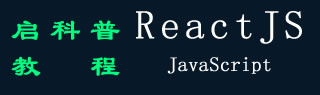
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - UNSAFE_componentWillReceiveProps() 方法
UNSAFE_componentWillReceiveProps 是一个 React 函数。它用于显示组件即将获取新的属性或上下文。请记住,如果组件使用 getDerivedStateFromProps 或 getSnapshotBeforeUpdate,则不会调用 UNSAFE_componentWillReceiveProps。此外,它不能保证组件会接收到新的道具,这对于最近的 React 特性(如 Suspense)来说尤其重要。
语法
UNSAFE_componentWillReceiveProps(nextProps, nextContext)
参数
- nextProps - 这些是组件从其父组件接收的新属性。我们可以通过比较 nextProps 和 this.props 来查看发生了什么变化。
- nextContext - 组件需要从最近的提供者处获取新的上下文。要找出不同之处,请将 nextContext 与 this.context 进行比较。仅当给定静态 contextType 或静态 contextTypes 时,此选项才可用。
返回值
该方法不返回任何内容。它只是 React 在属性或上下文即将更改时调用的一个函数。
例子
示例 - 消息应用程序
下面是一个如何在小型 React 应用程序中使用 UNSAFE_componentWillReceiveProps 的示例。在这个例子中,我们将创建一个组件,该组件显示一条消息,并在收到新的属性时更新它。
import React, { Component } from 'react';
class MessageComponent extends Component {
constructor(props) {
super(props);
this.state = {
message: props.initialMessage,
};
}
// This is the UNSAFE_componentWillReceiveProps function
UNSAFE_componentWillReceiveProps(nextProps) {
if (nextProps.newMessage !== this.props.newMessage) {
// Check if the new message is different
this.setState({ message: nextProps.newMessage });
}
}
render() {
return <div>{this.state.message}</div>;
}
}
class App extends Component {
constructor() {
super();
this.state = {
message: 'Hello, Tutorialspoint!',
newMessage: 'Welcome to the React App!',
};
}
updateMessage = () => {
this.setState({ newMessage: 'Message is Updated!' });
};
render() {
return (
<div>
<MessageComponent initialMessage={this.state.message} newMessage={this.state.newMessage} />
<button onClick={this.updateMessage}>Update Message</button>
</div>
);
}
}
export default App;
输出
App 和 MessageComponent 是上述示例中的两个组件。当收到新的属性时,MessageComponent 会用UNSAFE_componentWillReceiveProps更新其消息。App 组件有一个按钮,用于更改消息。
示例 - 计数器应用程序
让我们创建另一个简单的 React 应用程序,并将 CSS 应用于应用程序以进行样式设置。这个应用程序将是一个基本的待办事项列表,它显示了 UNSAFE_componentWillReceiveProps() 函数的用法。所以下面是这个应用程序提到的代码 -
TodoList.js
import React, { Component } from 'react';
import './TodoList.css';
class TodoList extends Component {
constructor(props) {
super(props);
this.state = {
todos: [],
newTodo: '',
};
}
UNSAFE_componentWillReceiveProps(nextProps) {
// Update todos when receiving new props
if (nextProps.todos !== this.props.todos) {
this.setState({ todos: nextProps.todos });
}
}
handleInputChange = (event) => {
this.setState({ newTodo: event.target.value });
};
handleAddTodo = () => {
if (this.state.newTodo) {
const updatedTodos = [...this.state.todos, this.state.newTodo];
this.setState({ todos: updatedTodos, newTodo: '' });
}
};
render() {
return (
<div className="todo-container App">
<h2>ToDo List</h2>
<ul>
{this.state.todos.map((todo, index) => (
<li key={index}>{todo}</li>
))}
</ul>
<div className="input-container">
<input
type="text"
value={this.state.newTodo}
onChange={this.handleInputChange}
placeholder="Add a new todo"
/>
<button onClick={this.handleAddTodo}>Add</button>
</div>
</div>
);
}
}
export default TodoList;
TodoList.css
.todo-container {
max-width: 400px;
margin: 20px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
ul {
list-style: none;
padding: 0;
}
li {
margin-bottom: 8px;
}
.input-container {
margin-top: 16px;
display: flex;
}
input {
flex-grow: 1;
padding: 8px;
margin-right: 8px;
}
button {
padding: 8px 16px;
background-color: #4caf50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
输出
示例 - 配置文件应用程序
下面是另一个使用 UNSAFE_componentWillReceiveProps() 和 CSS 进行样式设置的 React 应用的示例。这个应用程序是一个简单的“用户配置文件”组件,显示用户的姓名和年龄。
UserProfile.js
import React, { Component } from 'react';
import './UserProfile.css';
class UserProfile extends Component {
constructor(props) {
super(props);
this.state = {
name: 'John',
age: 20,
};
}
UNSAFE_componentWillReceiveProps(nextProps) {
// Update state when receiving new props
if (nextProps.user !== this.props.user) {
this.setState({
name: nextProps.user.name,
age: nextProps.user.age,
});
}
}
render() {
return (
<div className="user-profile-container">
<p className="user-name">Name: {this.state.name}</p>
<p className="user-age">Age: {this.state.age}</p>
</div>
);
}
}
export default UserProfile;
UserProfile.css
.user-profile-container {
max-width: 300px;
margin: 20px;
padding: 16px;
border: 1px solid #ddd;
border-radius: 4px;
box-shadow: 0 0 8px rgba(0, 0, 0, 0.1);
}
.user-name, .user-age {
font-size: 18px;
color: #333;
margin: 8px 0;
}
输出
请记住,在现代 React 中,我们通常使用其他生命周期方法或钩子来提供类似于 UNSAFE_componentWillReceiveProps 的功能。
总结
UNSAFE_componentWillReceiveProps 是一个旧的 React 生命周期函数,当组件要从其父级接收新属性时,会调用该函数。它用于通过将新道具与现有道具进行比较来响应道具更改。在处理新 React 代码中的 props 更改时,最好使用替代方案以获得更安全和一致的行为。