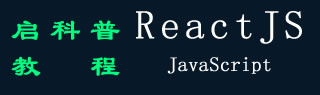
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - testInstance.findByProps() 方法
testInstance.findByProps(props) 是一个测试函数。testInstance 最有可能是指软件测试环境中的测试实例或对象。findByProps(props) 是一个组件的简单事实表明,我们正在测试实例中搜索特定的东西。术语“props”是指属性,即我们正在寻找的项的属性。
此代码查找具有特定属性的单个测试对象。这就像在许多项目中寻找特定项目一样。如果只有一个匹配项,则返回该实例。否则,将显示错误消息。
语法
testInstance.findByProps(props)
参数
- props - 术语“props”是指属性,即我们正在寻找的项的属性。
返回值
此代码查找具有特定功能的单个测试对象。这就像在寻找一个物体。如果只有一个匹配项,则返回该实例。如果不是这种情况,则会显示一条错误消息。
例子
示例 − 基本组件测试
使用 testInstance.findByProps(props) 方法创建 React 应用程序涉及编写测试用例以在我们的 React 组件中查找具有特定属性的特定元素。以下是此应用程序的简单代码 -
MyComponent.js
import React from 'react';
const MyComponent = ({ title }) => (
<div>
<h1>{title}</h1>
<p>Hello, this is a simple component.</p>
</div>
);
export default MyComponent;
MyComponent.test.js
import React from 'react';
import { render } from '@testing-library/react';
import MyComponent from './MyComponent';
test('finds the title using findByProps', () => {
const { findByProps } = render(<MyComponent title="My App" />);
const titleElement = findByProps({ title: 'My App' });
expect(titleElement).toBeInTheDocument();
});
输出
示例 - 列表组件测试
现在我们将创建一个名为 ListComponent 的 React 组件。它接受一个 prop 项,它是一个数组。它呈现一个无序列表 (<ul>),其中包含从 items 数组的元素生成的列表项 (<li>)。
然后,我们将为 ListComponent 组件创建ListComponent.test.js。它使用 @testing-library/react 库中的 render 函数来渲染带有 prop 项的 ListComponent。测试函数使用 findByProps 检查文本为“Item 2”的列表项是否存在于呈现的组件中。
ListComponent.js
import React from 'react';
const ListComponent = ({ items }) => (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
export default ListComponent;
ListComponent.test.js
import React from 'react';
import { render } from '@testing-library/react';
import ListComponent from './ListComponent';
test('finds the list item using findByProps', () => {
const items = ['Item 1', 'Item 2', 'Item 3'];
const { findByProps } = render(<ListComponent items={items} />);
const listItem = findByProps({ children: 'Item 2' });
expect(listItem).toBeInTheDocument();
});
输出
此代码使用项目列表测试 ListComponent 的呈现,并在 findByProps 方法的帮助下检查组件中是否存在特定列表项(“Item 2”)。
示例 − 表单组件测试
这次我们将创建一个名为 FormComponent 的 React 组件。它由一个带有输入字段的表单组成。输入字段使用 useState 钩子来管理 inputValue。标签具有 htmlFor 属性,输入字段使用相同的 id。
然后,我们将为 FormComponent 组件创建一个测试文件 (FormComponent.test.js)。该测试检查输入字段是否以空值 ('') 开头,并断言它最初存在于文档中。fireEvent.change 模拟用户在输入字段中键入“Hello, World!”。然后,它使用 findByProps 搜索具有更新值的输入字段,并断言它存在于文档中。
FormComponent.js
import React, { useState } from 'react';
const FormComponent = () => {
const [inputValue, setInputValue] = useState('');
return (
<form>
<label htmlFor="inputField">Type something:</label>
<input
type="text"
id="inputField"
value={inputValue}
onChange={(e) => setInputValue(e.target.value)}
/>
</form>
);
};
export default FormComponent;
FormComponent.test.js
import React from 'react';
import { render, fireEvent } from '@testing-library/react';
import FormComponent from './FormComponent';
test('finds the input value using findByProps', async () => {
const { findByProps } = render(<FormComponent />);
const inputElement = findByProps({ value: '' });
expect(inputElement).toBeInTheDocument();
fireEvent.change(inputElement, { target: { value: 'Hello, World!' } });
const updatedInputElement = findByProps({ value: 'Hello, World!' });
expect(updatedInputElement).toBeInTheDocument();
});
此代码通过检查输入字段在用户键入时是否正确更新来测试 FormComponent 的呈现和用户交互。
总结
因此,testInstance.findByProps(props) 帮助我们在测试过程中找到具有特定属性的对象。如果它恰好发现一个匹配项,则表示成功。否则,将发送错误。我们已经看到了不同的应用程序和案例,在这些应用程序中,我们可以使用此函数来测试 React 应用程序。