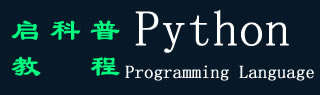
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python - f-strings
在 3.6 版中,Python 引入了一种新的字符串格式化方法,即 f-strings 或 Literal String Interpolation。使用这种格式化方法,您可以在字符串常量中使用嵌入式 Python 表达式。Python f-strings 是一种更快、更易读、更简洁且不易出错的方法。
使用 f-string 打印变量
要使用 f-strings 打印变量,你可以简单地将它们放在占位符 ({}) 内。字符串以 'f' 前缀开头,并在其中插入一个或多个占位符,其值是动态填充的。
例在下面的示例中,我们使用 f-strings 打印变量。
name = 'Qikepu'
age = 43
fstring = f'My name is {name} and I am {age} years old'
print (fstring)
它将产生以下输出 -
使用 f-string 计算表达式
f-string 还可用于计算 {} 占位符内的表达式。
例以下示例演示如何使用 f-string 计算表达式。
price = 10
quantity = 3
fstring = f'Price: {price} Quantity : {quantity} Total : {price*quantity}'
print (fstring)
它将产生以下输出 -
使用 f-string 打印词典键值
占位符可以由字典值填充。Dictionary 是 Python 中的内置数据类型之一,用于存储无序的键值对集合。
例在以下示例中,我们将使用 f-string 设置字典的格式。
user = {'name': 'Ramesh', 'age': 43}
fstring = f"My name is {user['name']} and I am {user['age']} years old"
print (fstring)
它将产生以下输出 -
f-string 中的自调试表达式
= 字符用于对 f-string 中的表达式进行自我调试,如以下示例所示 -
price = 10
quantity = 3
fstring = f"Total : {price*quantity=}"
print (fstring)
它将产生以下输出 -
使用 f-string 调用用户定义的函数
也可以在 f-string 表达式中调用用户定义的函数。为此,只需通过传递所需的参数来使用函数名称即可。
例下面的示例展示了如何在 f-string 中调用方法。
def total(price, quantity):
return price*quantity
price = 10
quantity = 3
fstring = f'Price: {price} Quantity : {quantity} Total : {total(price, quantity)}'
print (fstring)
它将产生以下输出 -
使用 f-string 进行精确处理
Python f-string 还支持使用精度规范格式化浮点数,如 format() 方法和字符串格式化运算符 %。
name="Qikepu"
age=43
percent=55.50
fstring = f"My name is {name} and I am {age} years old and I have scored {percent:6.3f} percent marks"
print (fstring)
它将产生以下输出 -
使用 f-string 进行字符串对齐
对于字符串变量,您可以指定对齐方式,就像我们使用 format() 方法和格式化运算符 % 一样。
name='qikepu'
fstring = f'Welcome To {name:>20} The largest qikepu Library'
print (fstring)
fstring = f'Welcome To {name:<20} The largest qikepu Library'
print (fstring)
fstring = f'Welcome To {name:^20} The largest qikepu Library'
print (fstring)
它将产生以下输出 -
Welcome To qikepu The largest qikepu Library
Welcome To qikepu The largest qikepu Library
使用 f-string 打印其他格式的数字
当分别与 “x”、“o” 和 “e” 一起使用时, f-strings 可以显示十六进制、八进制和科学记数法的数字。
例下面的示例演示如何使用 f-string 设置数字格式。
num= 20
fstring = f'Hexadecimal : {num:x}'
print (fstring)
fstring = f'Octal :{num:o}'
print (fstring)
fstring = f'Scientific notation : {num:e}'
print (fstring)
它将产生以下输出 -
Octal :24
Scientific notation : 2.000000e+01