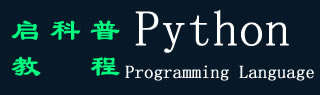
- Python 教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python dict() 函数
Python dict() 函数用于创建新字典。字典是一种存储键值对集合的数据结构。字典中的每个键都是唯一的,并映射到特定值。它是一个可变 (可变) 和无序的结构。
词典使用大括号 {} 定义,每个键值对用 “冒号” 分隔。例如,您可以使用字典来存储姓名和相应年龄等信息。
语法
以下是 Python dict() 函数的语法 -
dict(iterable)
参数
此函数接受元组列表作为参数,其中每个元组表示一个键值对。
返回值
此函数返回一个新的字典对象。
示例 1在下面的示例中,我们将使用带有关键字参数的 dict() 函数来创建一个字典 “person”,其中每个键都与特定值相关联 -
person = dict(name="Alice", age=30, city="New York")
print('The dictionary object obtained is:',person)
输出
以下是上述代码的输出 -
The dictionary object obtained is: {'name': 'Alice', 'age': 30, 'city': 'New York'}
示例 2
在这里,我们使用 dict() 函数将元组列表 “data_tuples” 转换为字典,方法是将每个元组的第一个元素关联为键,将第二个元素关联为值 -
data_tuples = [("a", 1), ("b", 2), ("c", 3)]
data_dict = dict(data_tuples)
print('The dictionary object obtained is:',data_dict)
输出
上述代码的输出如下 -
The dictionary object obtained is: {'a': 1, 'b': 2, 'c': 3}
示例 3
在这里,我们使用 dict() 函数和列表推导式将 “data_list” 中的字典合并为一个字典 -
data_list = [{'name': 'Alice'}, {'age': 25}, {'city': 'London'}]
data_dict = dict((key, value) for d in data_list for key, value in d.items())
print('The dictionary object obtained is:',data_dict)
输出
获得的结果如下所示 -
The dictionary object obtained is: {'name': 'Alice', 'age': 25, 'city': 'London'}
示例 4
在这种情况下,我们将 dict() 函数与 zip() 函数结合使用,将键和值列表中的元素配对以创建字典 -
keys = ["name", "age", "city"]
values = ["Bob", 28, "Paris"]
person_dict = dict(zip(keys, values))
print('The dictionary object obtained is:',person_dict)
输出
以下是上述代码的输出 -
The dictionary object obtained is: {'name': 'Bob', 'age': 28, 'city': 'Paris'}
示例 5
在此示例中,我们首先使用 dict() 函数初始化一个空字典 “empty_dict”。随后,我们通过添加键值对来填充字典 -
empty_dict = dict()
empty_dict["name"] = "John"
empty_dict["age"] = 30
empty_dict["city"] = "Berlin"
print('The dictionary object obtained is:',empty_dict)
输出
生成的结果如下 -
The dictionary object obtained is: {'name': 'John', 'age': 30, 'city': 'Berlin'}