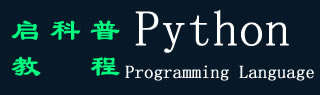
- Python 菜鸟教程
- Python 教程
- Python - 概述
- Python - 历史
- Python - 特性
- Python 与 C++
- Python - Hello World 程序
- Python - 应用领域
- Python 解释器及其模式
- Python - 环境设置
- Python - 虚拟环境
- Python - 基本语法
- Python - 变量
- Python - 数据类型
- Python - 类型转换
- Python - Unicode 系统
- Python - 文字
- Python - 运算符
- Python - 算术运算符
- Python - 比较运算符
- Python - 赋值运算符
- Python - 逻辑运算符
- Python - 按位运算符
- Python - 成员资格运算符
- Python - 身份运算符
- Python - 运算符优先级
- Python - 注释
- Python - 用户输入
- Python - 数字
- Python - 布尔值
- Python 控制语句
- Python - 控制流
- Python - 决策
- Python - if 语句
- Python - if-else 语句
- Python - 嵌套 if 语句
- Python - Match-Case 语句
- Python - 循环
- Python - For 循环
- Python for-else 循环
- Python - While 循环
- Python - break 语句
- Python - Continue 语句
- Python - pass 语句
- Python - 嵌套循环
- Python 函数和模块
- Python - 函数
- Python - 默认参数
- Python - 关键字参数
- Python - 仅关键字参数
- Python - 位置参数
- Python - 仅位置参数
- Python - 任意或可变长度参数
- Python - 变量范围
- Python - 函数注释
- Python - 模块
- Python - 内置函数
- Python 字符串
- Python - 字符串
- Python - 切片字符串
- Python - 修改字符串
- Python - 字符串连接
- Python - 字符串格式化
- Python - 转义字符
- Python - 字符串方法
- Python - 字符串练习
- Python 列表
- Python - 列表
- Python - 访问列表项
- Python - 更改列表项
- Python - 添加列表项
- Python - 删除列表项
- Python - 循环列表
- Python - 列表推导式
- Python - 排序列表
- Python - 复制列表
- Python - 联接列表
- Python - 列表方法
- Python - 列表练习
- Python 元组
- Python - 元组(Tuple )
- Python - 访问元组项
- Python - 更新元组
- Python - 解压缩元组项
- Python - 循环元组
- Python - 联接元组
- Python - 元组方法
- Python - 元组练习
- Python 集
- Python - 集(sets)
- Python - 访问 Set Items
- Python - 添加 Set Items
- Python - 删除 Set Items
- Python - 循环 Set Items
- Python - 联接 Sets
- Python - 复制 Set
- Python - Set 运算符
- Python - Set 方法
- Python - Set 的练习
- Python 字典
- Python - 字典
- Python - 访问字典项
- Python - 更改字典项
- Python - 添加字典项
- Python - 删除字典项
- Python - 字典视图对象
- Python - 循环字典
- Python - 复制字典
- Python - 嵌套字典
- Python - 字典方法
- Python - 字典练习
- Python 数组
- Python - 数组
- Python - 访问数组项
- Python - 添加数组项
- Python - 删除数组项
- Python - 循环数组
- Python - 复制数组
- Python - 反向数组
- Python - 对数组进行排序
- Python - 连接数组
- Python - 数组方法
- Python - 数组练习
- Python 文件处理
- Python - 文件处理
- Python - 写入文件
- Python - 读取文件
- Python - 重命名和删除文件
- Python - 目录
- Python - 文件方法
- Python OS 文件/目录方法
- Python - os.path 方法
- 面向对象编程
- Python - OOP 概念
- Python - 类和对象
- Python - 类属性
- Python - 类方法
- Python - 静态方法
- Python - 构造函数
- Python - 访问修饰符
- Python - 继承
- Python - 多态性
- Python - 方法覆盖
- Python - 方法重载
- Python - 动态绑定
- Python - 动态类型
- Python - 抽象
- Python - 封装
- Python - 接口
- Python - 软件包
- Python - 内部类
- Python - 匿名类和对象
- Python - 单例类
- Python - 包装类
- Python - 枚举
- Python - 反射
- Python 错误和异常
- Python - 语法错误
- Python - 异常处理
- Python - try-except 块
- Python - try-finally 块
- Python - 引发异常
- Python - 异常链接
- Python - 嵌套 try 块
- Python - 用户定义的异常
- Python - 日志记录
- Python - 断言
- Python - 内置异常
- Python 多线程
- Python - 多线程
- Python - 线程生命周期
- Python - 创建线程
- Python - 启动线程
- Python - 联接线程
- Python - 命名线程
- Python - 线程调度
- Python - 线程池
- Python - 主线程
- Python - 线程优先级
- Python - 守护程序线程
- Python - 同步线程
- Python 同步
- Python - 线程间通信
- Python - 线程死锁
- Python - 中断线程
- Python 网络
- Python - 网络编程
- Python - 套接字编程
- Python - URL 处理
- Python - 泛型
- Python 杂项
- Python - 日期和时间
- Python - math 模块
- Python - 迭代器
- Python - 生成器
- Python - 闭包(closures)
- Python - 装饰器( Decorators)
- Python - 递归
- Python - 正则表达式
- Python - PIP
- Python - 数据库访问
- Python - 弱引用
- Python - 序列化
- Python - 模板
- Python - 输出格式
- Python - 性能测量
- Python - 数据压缩
- Python - CGI 编程
- Python - XML 处理
- Python - GUI 编程
- Python - 命令行参数
- Python - 文档字符串
- Python - JSON
- Python - 发送电子邮件
- Python - 更多扩展
- Python - 工具/实用程序
- Python - 图形用户界面
- Python 高级概念
- Python - 抽象基类
- Python - 自定义异常
- Python - 高阶函数
- Python - 对象内部
- Python - 内存管理
- Python - 元类
- Python - 使用 Metaclasses 进行元编程
- Python - 模拟和存根
- Python - 猴子修补
- Python - 信号处理
- Python - 类型提示
- Python - 自动化教程
- Python - 人性化软件包
- Python - 上下文管理器
- Python - 协程
- Python - 描述符
- Python - 诊断和修复内存泄漏
- Python - 不可变数据结构
Python 字典 dict.get() 方法
Python dictionary get() 方法用于检索与指定 key 对应的值。
此方法接受键和值,其中 value 是可选的。如果在字典中找不到键,但指定了值,则此方法将检索指定的值。另一方面,如果在字典中找不到键并且也没有指定值,则此方法检索 None。
使用字典时,通常的做法是在字典中检索指定键的值。例如,如果您经营一家书店,您可能希望了解您收到了多少图书订单。
语法
以下是 Python dictionary get() 方法的语法 -
dict.get(key, value)
参数
此方法接受两个参数,如下所示:
- key − 这是要在字典中搜索的 Key。
- value (可选) − 这是在指定键不存在时要返回的 Value。它的默认值为 None。
返回值
此方法返回给定键的值。
如果 key 不可用且未给出值,则返回默认值 None。
如果未给出 key 且指定了值,则返回此值。
例如果作为参数传递给 get() 方法的键存在于字典中,则返回其相应的值。
以下示例显示了 Python dictionary get() 方法的用法。这里创建了一个字典 'dict',其中包含键 'Name' 和 'Age'。然后,键 'Age' 作为参数传递给该方法。因此,从字典中检索其相应的值 '7':
# creating the dictionary
dict = {'Name': 'Zebra', 'Age': 7}
# printing the value of the given key
print ("Value : %s" % dict.get('Age'))
当我们运行上述程序时,它会产生以下结果——
例
如果作为参数传递的键在字典中不存在,并且也未指定该值,则此方法返回默认值 None。
在这里,键 'Roll_No' 作为参数传递给 get() 方法。在字典 'dict' 中找不到此键,并且也没有指定该值。因此,使用 dict.get() 方法返回默认值 'None'。
# creating the dictionary
dict = {'Name': 'Rahul', 'Age': 7}
# printing the value of the given key
print ("The value is: ", dict.get('Roll_No'))
以下是上述代码的输出 -
例
如果我们传递一个键及其值作为字典中不存在的参数,那么这个方法会返回指定的值。
在下面的代码中,键 'Education' 和值 'Never' 作为参数传递给 dict.get() 方法。由于在字典 'dict' 中找不到给定的键,因此返回当前值。
# creating the dictionary
dict = {'Name': 'Zebra', 'Age': 7}
res = dict.get('Education', "Never")
print ("Value : %s" % res)
在执行上述代码时,我们得到以下输出 -
例
在下面给出的示例中,创建了一个嵌套字典 'dict_1' 。然后使用嵌套的 get() 方法返回给定键的值。
dict_1 = {'Universe' : {'Planet' : 'Earth'}}
print("The dictionary is: ", dict_1)
# using nested get() method
result = dict_1.get('Universe', {}).get('Planet')
print("The nested value obtained is: ", result)
上述代码的输出如下 -
The nested value obtained is: Earth