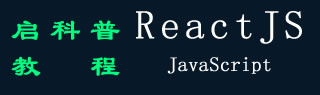
- ReactJS 教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - 使用创建 React 应用程序工具
让我们学习使用 Create React App 工具创建费用管理应用程序。
打开终端并转到您的工作区。
> cd /go/to/your/workspace
接下来,使用 Create React App 工具创建新的 React 应用程序。
> create-react-app expense-manager
它将使用启动模板代码创建一个新文件夹 expense-manager。
接下来,转到 expense-manager 文件夹并安装必要的库。
cd expense-manager
npm install
npm install
npm install 将在 node_modules 文件夹下安装必要的库。
接下来,启动应用程序。
npm start
Compiled successfully!
You can now view react-cra-web-app in the browser.
Local: http://localhost:3000
On Your Network: http://192.168.56.1:3000
Note that the development build is not optimized.
To create a production build, use npm run build.
Compiled successfully!
You can now view react-cra-web-app in the browser.
Local: http://localhost:3000
On Your Network: http://192.168.56.1:3000
Note that the development build is not optimized.
To create a production build, use npm run build.
接下来,打开浏览器并在地址栏中输入 http://localhost:3000,然后按 Enter。开发 Web 服务器将为我们的网页提供服务,如下所示。

让我们分析一下 React 应用程序的结构。
文件和文件夹
React 应用程序的内容如下 -
|-- README.md
|-- node_modules
|-- package-lock.json
|-- package.json
|-- public
| |-- favicon.ico
| |-- index.html
| |-- logo192.png
| |-- logo512.png
| |-- manifest.json
| `-- robots.txt
`-- src
|-- App.css
|-- App.js
|-- App.test.js
|-- index.css
|-- index.js
|-- logo.svg
|-- reportWebVitals.js
`-- setupTests.js
这里
package.json 是表示项目的核心文件。它配置整个项目,由项目名称、项目依赖项以及用于构建和运行应用程序的命令组成。
{
"name": "expense-manager",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.11.6",
"@testing-library/react": "^11.2.2",
"@testing-library/user-event": "^12.6.0",
"react": "^17.0.1",
"react-dom": "^17.0.1",
"react-scripts": "4.0.1",
"web-vitals": "^0.2.4"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
"eslintConfig": {
"extends": [
"react-app",
"react-app/jest"
]
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
}
}
package.json 在其依赖项部分引用了下面的 React 库。
- React 和 react-DOM 是用于开发 Web 应用程序的核心 React 库。
- web-vitals 是通用库,用于支持不同浏览器中的应用程序。
- react-scripts 是用于构建和运行应用程序的核心 React 脚本。
- @testing-library/jest-dom、@testing-library/react 和 @testing-library/user-event 是测试库,用于在开发后测试应用程序。
- public 文件夹 − 包含核心文件、index.html和其他 Web 资源,如图像、徽标、机器人等,index.html加载我们的 React 应用程序并在用户的浏览器中呈现它。
- src 文件夹 − 包含应用程序的实际代码。我们将在下一节检查它。
应用程序的源代码
让我们检查一下本章中应用程序的每一个源代码文档。
- index.js - 我们应用程序的入口点。它使用 ReactDOM.render 方法来启动和启动应用程序。代码如下 -
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
这里
React.StrictMode 是一个内置组件,用于通过分析组件的不安全生命周期、不安全的 API 使用、已弃用的 API 使用等来防止意外错误,并引发相关警告。
- App 是应用程序的第一个自定义和根组件。所有其他组件都将在 App 组件中呈现。
App.js − 应用程序的 Root 组件。让我们替换现有的 JSX 并显示简单的你好反应消息,如下所示 -
import './App.css';
function App() {
return (
<h1>Hello React!</h1>
);
}
export default App;
- App.css - 用于设置 App 组件的样式。让我们删除所有样式并从新代码开始。
- App.test.js − 用于为我们的组件编写单元测试函数。
- setupTests.js − 用于为我们的应用程序设置测试框架。
- reportWebVitals.js - 支持所有浏览器的通用 Web 应用程序启动代码。
- logo.svg − SVG 格式的徽标,可以使用 import 关键字加载到我们的应用程序中。让我们将其从项目中删除。