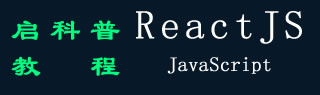
- ReactJS 菜鸟教程
- ReactJS 教程
- ReactJS - 简介
- ReactJS - 安装
- ReactJS - 特性
- ReactJS - 优点和缺点
- ReactJS - 架构
- ReactJS - 创建 React 应用程序
- ReactJS - JSX
- ReactJS - 组件
- ReactJS - 嵌套组件
- ReactJS - 使用组件
- ReactJS - 组件集合
- ReactJS - 样式
- ReactJS - 属性(props)
- ReactJS - 使用属性创建组件
- ReactJS - props 验证
- ReactJS - 构造函数
- ReactJS - 组件生命周期
- ReactJS - 事件管理
- ReactJS - 创建事件感知组件
- ReactJS - 在Expense Manager APP中引入事件
- ReactJS - 状态管理
- ReactJS - 状态管理 API
- ReactJS - 无状态组件
- ReactJS - 使用 React Hooks 进行状态管理
- ReactJS - 使用 React 钩子的组件生命周期
- ReactJS - 组件的布局
- ReactJS - 分页
- ReactJS - Material 用户界面
- ReactJS - Http 客户端编程
- ReactJS - 表单编程
- ReactJS - 受控组件
- ReactJS - 不受控制的组件
- ReactJS - Formik
- ReactJS - 条件渲染
- ReactJS - 列表
- ReactJS - 键
- ReactJS - 路由
- ReactJS - 冗余
- ReactJS - 动画
- ReactJS - 引导程序
- ReactJS - 地图
- ReactJS - 表格
- ReactJS - 使用 Flux 管理状态
- ReactJS - 测试
- ReactJS - CLI 命令
- ReactJS - 构建和部署
- ReactJS - 示例
- ReactJS - 钩子简介
- ReactJS - 使用 useState
- ReactJS - 使用 useEffect
- ReactJS - 使用 useContext
- ReactJS - 使用 useRef
- ReactJS - 使用 useReducer
- ReactJS - 使用 useCallback
- ReactJS - 使用 useMemo
- ReactJS - 自定义钩子
- ReactJS - 可访问性
- ReactJS - 代码拆分
- ReactJS - 上下文
- ReactJS - 错误边界
- ReactJS - 转发引用
- ReactJS - 片段
- ReactJS - 高阶组件
- ReactJS - 与其他库集成
- ReactJS - 优化性能
- ReactJS - 分析器 API
- ReactJS - 门户
- ReactJS - 没有 ES6 ECMAScript 的 React
- ReactJS - 没有 JSX 的 React
- ReactJS - 协调
- ReactJS - 引用和 DOM
- ReactJS - 渲染属性
- ReactJS - 静态类型检查
- ReactJS - 严格模式
- ReactJS - Web 组件
- ReactJS - 日期选择器
- ReactJS - Helmet
- ReactJS - 内联样式
- ReactJS - 属性类型
- ReactJS - 浏览器路由器
- ReactJS - DOM
- ReactJS - 旋转木马
- ReactJS - 图标
- ReactJS - 表单组件
- ReactJS - 参考 API
ReactJS - 静态 propTypes 属性
我们可以在 React 中使用“propTypes”来定义我们的组件应该接收的属性(props)类型。这确保了为我们的组件提供正确的信息,使我们的代码更安全。
PropTypes 可以看作是表达我们的组件期望接收到的信息类型的一种方式。这是对我们的组件说的一种形式,“嘿,我期待某种类型的数据,所以请确保我们得到它!
换句话说,我们可以说 PropTypes 是 React 中的一种方法,用于对 React 组件的 props 进行类型检查。它通过定义每个参数的预期类型来帮助开发人员检测常见问题。propTypes 属性作为静态属性添加到 React 组件类中,这意味着它链接到类而不是与类的实例链接。
导入 propTypes
import PropTypes from 'prop-types';
例子
示例 1
因此,我们正在创建一个带有静态 propTypes 的小型 React 应用程序,以定义我们的组件将需要的 props 类型。让我们创建一个接受我们的名字和问候的基本应用程序。
import React from 'react';
import PropTypes from 'prop-types';
class Greeting extends React.Component {
// Define propTypes
static propTypes = {
name: PropTypes.string.isRequired, // 'isRequired' means this prop is mandatory
};
render() {
return (
<div>
<h1>Hello, {this.props.name}!</h1>
</div>
);
}
}
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
userName: '',
};
}
handleNameChange = (event) => {
this.setState({ userName: event.target.value });
};
render() {
return (
<div>
<h1>Simple Greeting App</h1>
<label>
Enter your name:
<input
type="text"
value={this.state.userName}
onChange={this.handleNameChange}
/>
</label>
{/* Render the Greeting component and pass the name as a prop */}
<Greeting name={this.state.userName} />
</div>
);
}
}
export default App;
输出
在此示例中,我们有两个组件:Greeting 和 App。Greeting 组件需要一个名为 name 的字符串类型属性,该属性被标记为 isRequired,表示必须给出它。
App 组件是一个简单的应用程序,我们可以在其中输入我们的名字到输入框中。然后,给定的名称被发送到 Greeting 组件,该组件作为 prop 显示问候消息。
在启动此应用程序之前,请记住设置我们的 React 环境并安装必要的包,包括 prop-types。
示例 2
让我们创建另一个使用静态 propTypes 的小型 React 应用程序。这次我们来创建一个 Counter 应用。Counter 组件将显示一个数字作为属性。如果未指定数字,则将其设置为 0。
import React from 'react';
import PropTypes from 'prop-types';
class Counter extends React.Component {
// Define propTypes for the Counter component
static propTypes = {
number: PropTypes.number.isRequired,
};
render() {
return (
<div>
<h2>Counter: {this.props.number}</h2>
</div>
);
}
}
class CounterApp extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
handleIncrement = () => {
this.setState((prevState) => ({ count: prevState.count + 1 }));
};
handleDecrement = () => {
this.setState((prevState) => ({ count: prevState.count - 1 }));
};
render() {
return (
<div>
<h1>Simple Counter App</h1>
{/* Render the Counter component and pass the count as a prop */}
<Counter number={this.state.count} />
<button onClick={this.handleIncrement}>Increment</button>
<button onClick={this.handleDecrement}>Decrement</button>
</div>
);
}
}
export default CounterApp;
输出
我们在此应用程序中创建了一个 Counter 组件,其中包含用于数字属性的 propTypes。CounterApp 组件保留计数和按钮,用于在其状态下增加和减少计数。Counter 组件显示当前计数。
示例 3
让我们再创建一个使用静态 propTypes 的小型 React 应用程序。这一次,我们将创建一个 ColorBox 组件,该组件将颜色作为属性并显示一个彩色框。
import React from 'react';
import PropTypes from 'prop-types';
class ColorBox extends React.Component {
// Define propTypes for the ColorBox component
static propTypes = {
color: PropTypes.string.isRequired,
};
render() {
const boxStyle = {
width: '100px',
height: '100px',
backgroundColor: this.props.color,
};
return (
<div>
<h2>Color Box</h2>
<div style={boxStyle}></div>
</div>
);
}
}
class ColorBoxApp extends React.Component {
constructor(props) {
super(props);
this.state = {
selectedColor: 'blue',
};
}
handleColorChange = (event) => {
this.setState({ selectedColor: event.target.value });
};
render() {
return (
<div>
<h1>Color Box App</h1>
<label>
Select a color:
<select value={this.state.selectedColor} onChange={this.handleColorChange}>
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
</label>
<ColorBox color={this.state.selectedColor} />
</div>
);
}
}
export default ColorBoxApp;
输出
在此应用中,ColorBox 组件对颜色属性具有 propTypes 要求。ColorBoxApp 组件将选定的颜色保持其状态,并提供颜色选择下拉列表。然后,使用 ColorBox 组件根据已选定的颜色显示彩色框。
总结
PropTypes 是一个 React 特性,它允许我们定义我们的组件将接收的预期属性类型(props)。它允许开发人员在开发之前指定 props 的数据类型,从而帮助检测潜在问题。