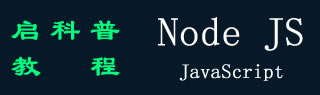
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - Web 模块
什么是 Web 服务器?
Node.js 中的 http 模块支持通过超文本传输协议 (HTTP) 在服务器和客户端之间传输数据。http 模块中的 createServer() 函数创建 http 服务器的实例Node.js。它通过指定的主机和端口侦听来自其他 http 客户端的传入请求。
Node.js服务器是一个软件应用程序,它处理HTTP客户端(如Web浏览器)发送的HTTP请求,并返回网页以响应客户端。Web 服务器通常提供 html 文档以及图像、样式表和脚本。
大多数 Web 服务器都支持服务器端脚本,使用脚本语言或将任务重定向到应用程序服务器,应用程序服务器从数据库中检索数据并执行复杂的逻辑,然后通过 Web 服务器将结果发送到 HTTP 客户端。
Web 应用程序架构
Web应用程序通常分为四层:
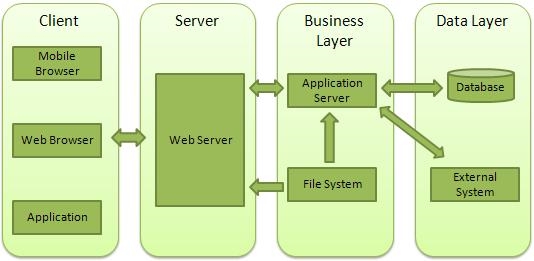
- 客户端 - 该层由Web浏览器,移动浏览器或应用程序组成,它们可以向Web服务器发出HTTP请求。
- 服务器 - 这一层有Web服务器,它可以拦截客户端发出的请求,并向它们传递响应。
- 业务 - 此层包含应用程序服务器,Web 服务器使用它来执行所需的处理。该层通过数据库或一些外部程序与数据层进行交互。
- 数据 - 此层包含数据库或任何其他数据源。
使用 Node 创建 Web 服务器
Node.js提供了一个 http 模块,可用于创建服务器的 HTTP 客户端。以下是在 5000 端口侦听的 HTTP 服务器的最低结构。
创建一个名为 server.js 的 js 文件 -
var http = require('http');
var fs = require('fs');
var url = require('url');
// Create a server
http.createServer( function (request, response) {
// Parse the request containing file name
var pathname = url.parse(request.url).pathname;
// Print the name of the file for which request is made.
console.log("Request for " + pathname + " received.");
// Read the requested file content from file system
fs.readFile(pathname.substr(1), function (err, data) {
if (err) {
console.log(err);
} else {
response.writeHead(200, {'Content-Type': 'text/html'});
// Write the content of the file to response body
console.log(data.toString());
response.write(data.toString());
}
// Send the response body
response.end();
});
}).listen(5000);
// Console will print the message
console.log('Server running at http://127.0.0.1:5000/');
createServer() 函数开始在 localhost 的 5000 端口上侦听客户端请求。Http Request 和 Server Response 对象由 Nde.js 服务器内部提供。它获取 HTTP 客户端请求的 HTML 文件的 URL。createServer() 函数的回调函数读取请求的文件,并将其内容写入服务器的响应。
您可以从服务器计算机本身的浏览器发送 HTTP 请求。运行 aboce server.js文件,并在浏览器窗口中输入 http://localhost:5000/index.html 作为 URL。将呈现index.html页面的内容。
为任何其他现有网页(如 http://localhost:5000/hello.html)发送请求,将呈现所请求的页面。
使用 Node 创建 Web 客户端
可以使用 http 模块创建 Web 客户端。让我们看一下以下示例。
创建一个名为 client.js 的 js 文件(将此文件保存在另一个文件夹中,而不是在包含server.js脚本的文件夹中) -
var http = require('http');
var fs = require('fs');
var path = require('path');
// Options to be used by request
var options = {
host: 'localhost',
port: '5000',
path: path.join('/',process.argv[2])
};
var body = '';
// Callback function is used to deal with response
var callback = function(response) {
// Continuously update stream with data
response.on('data', function(data) {
body += data;
});
response.on('end', function() {
// Data received completely.
console.log(body);
fs.writeFile(options.path.substr(1), body, function (err) {
if (err)
console.log(err);
else
console.log('Write operation complete.');
});
});
}
// Make a request to the server
var req = http.request(options, callback);
req.end();
此客户端脚本为作为命令行参数给出的网页发送 HTTP 请求。例如 -
文件的名称是参数列表中的 argv[2]。它用作 options 参数中的路径参数,以传递给 http.request() 方法。一旦服务器接受此请求,其内容就会写入响应流中。client.js接收此响应,并将收到的数据作为新文件写入客户端的文件夹中。
首先运行服务器代码。从另一个终端运行客户端代码。您将看到在客户端文件夹中创建的请求的 HTML 文件。