MongoDB是一个面向文档的数据库,可以通过名为mongodb的NPM模块与Node.js对接。文档是 MongoDB 数据库的核心。它是键值对的集合。我们也可以将其视为类似于基于SQL的关系数据库的表中的单行,就像MongoDB中的Collection类似于关系数据库中的表一样。NPM mongodb 包提供了 insertOne() 和 insertMany() 方法,使用这些方法可以在 Node.js 应用程序中将一个或多个文档添加到集合中。
MongoDB将数据记录存储为BSON文档。BSON 是 JSON 文档的二进制表示。MongoDB文档由字段和值对组成,并具有以下结构 -
{
field1: value1,
field2: value2,
field3: value3,
...
fieldN: valueN
}
字段的值可以是任何 BSON 数据类型,包括其他文档、数组和文档数组。
每个文档都有一个称为“_id”的特殊键,该键具有唯一值,类似于关系数据库实体表中的主键。键也称为字段。如果插入的文档省略了 _id字段,MongoDB 驱动程序会自动为_id字段生成 ObjectId。
insertOne() 方法
Collection 对象具有 insertOne() 方法,用于将单个文档插入到集合中。
Collection.insertOne(doc)
要插入的文档将作为参数传递。它将单个文档插入到 MongoDB 中。如果传入的文档不包含_id字段,则驱动程序将向缺少该字段的每个文档添加一个字段,从而改变文档。
例通过以下代码,我们将单个文档插入到数据库的产品集合中。
const {MongoClient} = require('mongodb');
async function main(){
const uri = "mongodb://localhost:27017";
const client = new MongoClient(uri);
try {
// Connect to the MongoDB cluster
await client.connect();
// Make the appropriate DB calls
// Create a single new document
await createdoc(client, "mydatabase", "products", {
"ProductID":1, "Name":"Laptop", "Price":25000
});
} finally {
// Close the connection to the MongoDB cluster
await client.close();
}
}
main().catch(console.error);
async function createdoc(client, dbname, colname, doc){
const dbobj = await client.db(dbname);
const col = dbobj.collection(colname);
const result = await col.insertOne(doc);
console.log(`New document created with the following id: ${result.insertedId}`);
}
输出
使用以下 ID 创建的新房源:65809214693bd4622484dce3
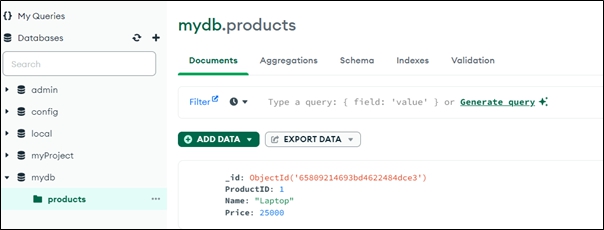
Mongo shell 也可用于查看插入的文档。
> use mydb;
< switched to db mydb
> products=db['products']
< mydb.products
> docs=products.find()
{
_id: ObjectId("65809214693bd4622484dce3"),
ProductID: 1,
Name: 'Laptop',
Price: 25000
}
insertMany() 方法
Collection 对象的 insertMany() 方法使插入多个文档成为可能。JSON 文档数组用作参数。我们还使用SRV连接字符串,以下示例 -
例
const {MongoClient} = require('mongodb');
async function main(){
//const uri = "mongodb://localhost:27017";
const uri = "mongodb+srv://user:mypwd@cluster0.zhmrg1h.mongodb.net/?retryWrites=true&w=majority";
const client = new MongoClient(uri);
try {
// Connect to the MongoDB cluster
await client.connect();
// Make the appropriate DB calls
// insert documents
await createdocs(client, [
{'ProductID':1, 'Name':'Laptop', 'price':25000},
{'ProductID':2, 'Name':'TV', 'price':40000},
{'ProductID':3, 'Name':'Router', 'price':2000},
{'ProductID':4, 'Name':'Scanner', 'price':5000},
{'ProductID':5, 'Name':'Printer', 'price':9000}
]);
} finally {
// Close the connection to the MongoDB cluster
await client.close();
}
}
main().catch(console.error);
async function createdocs(client, docs){
const result = await client.db("mydb").collection("products").insertMany(docs);
console.log(`${result.insertedCount} new document(s) created with the following id(s):`);
console.log(result.insertedIds);
}
输出
5 new listing(s) created with the following id(s):
{
'0': new ObjectId('6580964f20f979d2e9a72ae7'),
'1': new ObjectId('6580964f20f979d2e9a72ae8'),
'2': new ObjectId('6580964f20f979d2e9a72ae9'),
'3': new ObjectId('6580964f20f979d2e9a72aea'),
'4': new ObjectId('6580964f20f979d2e9a72aeb')
}
{
'0': new ObjectId('6580964f20f979d2e9a72ae7'),
'1': new ObjectId('6580964f20f979d2e9a72ae8'),
'2': new ObjectId('6580964f20f979d2e9a72ae9'),
'3': new ObjectId('6580964f20f979d2e9a72aea'),
'4': new ObjectId('6580964f20f979d2e9a72aeb')
}
您可以将文档集合导出为CSV格式。
_id,ProductID,Name,Price,price
65809214693bd4622484dce3,1,Laptop,25000,
6580964f20f979d2e9a72ae8,2,TV,40000
6580964f20f979d2e9a72ae9,3,Router,2000
6580964f20f979d2e9a72aea,4,Scanner,5000
6580964f20f979d2e9a72aeb,5,Printer,9000