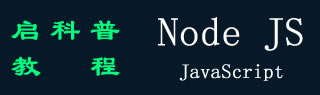
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - 回调概念
什么是回调?
Node.js 中的回调是函数的异步等效项。它是一种特殊类型的函数,作为参数传递给另一个函数。Node.js大量使用回调。回调帮助我们进行异步调用。Node 的所有 API 都以支持回调的方式编写。
默认情况下,编程指令是同步执行的。如果程序中的一条指令需要执行一个漫长的过程,那么执行的主线程就会被阻塞。只有在当前 I/O 完成后,才能执行后续指令。这就是回调的用武之地。
当包含回调作为参数的函数完成其执行时,将调用回调,并允许回调中的代码在此期间运行。这使得Node.js具有高度可扩展性,因为它可以处理大量请求,而无需等待任何函数返回结果。
在Node.js中实现回调的语法如下:
function function_name(argument, function (callback_argument){
// callback body
})
Node.js 中的 setTimeout() 函数是回调的典型示例。以下代码调用异步 setTimeout() 方法,该方法等待 1000 毫秒,但不会阻塞线程。取而代之的是随后的 Hello World 消息,然后是定时消息。
例
setTimeout(function () {
console.log('This prints after 1000 ms');
}, 1000);
console.log("Hello World");
输出
This prints after 1000 ms
阻塞代码示例
要了解回调功能,请将以下文本另存为input.txt文件。
Master any technology.
From programming languages and web development to data science and cybersecurity
以下代码借助 fs 模块中的 readFileSync() 函数同步读取文件。由于操作是同步的,因此它会阻止其余代码的执行。
var fs = require("fs");
var data = fs.readFileSync('input.txt');
console.log(data.toString());
let i = 1;
while (i <=5) {
console.log("The number is " + i);
i++;
}
输出显示Node.js读取文件并显示其内容。仅在此之后,才会执行打印数字 1 到 5 的下一个循环。
Master any technology.
From programming languages and web development to data science and cybersecurity
The number is 1
The number is 2
The number is 3
The number is 4
The number is 5
非阻塞代码示例
我们在下面的代码中使用相同的input.txt文件来演示回调的使用。
Master any technology.
From programming languages and web development to data science and cybersecurity
fs 模块中的 ReadFile() 函数提供了一个回调函数。传递给回调的两个参数是 error 和 ReadFile() 函数本身的返回值。当 ReadFile() 通过返回错误或文件内容完成时,将调用回调。当文件读取操作在进程内时,Node.js异步运行后续循环。
var fs = require("fs");
fs.readFile('input.txt', function (err, data) {
if (err) return console.error(err);
console.log(data.toString());
});
let i = 1;
while (i <=5) {
console.log("The number is " + i);
i++;
}
输出
The number is 2
The number is 3
The number is 4
The number is 5
Qikepu is the largest free online tutorials Library
Master any technology.
From programming languages and web development to data science and cybersecurity
作为箭头函数的回调
您还可以将箭头函数分配为回调参数。JavaScript 中的箭头函数是一个匿名函数。它也被称为 lambda 函数。使用箭头函数作为回调Node.js语法如下 -
function function_name(argument, (callback_argument) => {
// callback body
})
它是在 JavaScript 的 ES6 版本中引入的。让我们用箭头函数替换上面示例中的回调。
var fs = require("fs");
fs.readFile('input.txt', (err, data) => {
if (err) return console.error(err);
console.log(data.toString());
});
let i = 1;
while (i <=5) {
console.log("The number is " + i);
i++;
}
上述代码生成与上一个示例类似的输出。