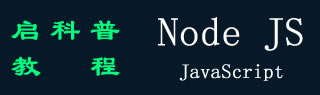
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - MongoDB 创建集合
MongoDB 数据库由一个或多个集合组成。Collection 是一组文档对象。在MongoDB服务器(独立服务器或MongoDB Atlas中的共享集群)上创建数据库后,您可以在其中创建集合。Node.js 的 mongodb 驱动程序有一个 cerateCollection() 方法,该方法返回一个 Collection 对象。
MongoDB中的集合类似于关系数据库中的表。但是,它没有预定义的架构。集合中的每个文档都可以由数量可变的 k-v 对组成,每个文档中不一定具有相同的键。
要创建集合,请从数据库连接获取数据库对象并调用 createCollection() 方法。
db.createCollection(name: string, options)
要创建的集合的名称将作为参数传递。该方法返回一个 Promise。集合命名空间验证在服务器端执行。
const dbobj = await client.db(dbname);
const collection = await dbobj.createCollection("MyCollection");
请注意,当您在其中插入文档时,即使未在插入之前创建该集合,也会隐式创建该集合。
const result = await client.db("mydatabase").collection("newcollection").insertOne({k1:v1, k2:v2});
例
以下Node.js代码在名为 mydatabase 的 MongoDB 数据库中创建名为 MyCollection 的 Collection。
const {MongoClient} = require('mongodb');
async function main(){
const uri = "mongodb://localhost:27017/";
const client = new MongoClient(uri);
try {
// Connect to the MongoDB cluster
await client.connect();
await newcollection(client, "mydatabase");
} finally {
// Close the connection to the MongoDB cluster
await client.close();
}
}
main().catch(console.error);
async function newcollection (client, dbname){
const dbobj = await client.db(dbname);
const collection = await dbobj.createCollection("MyCollection");
console.log("Collection created");
console.log(collection);
}
MongoDB Compass 显示 MyCollection 是在 mydatabase 中创建的。
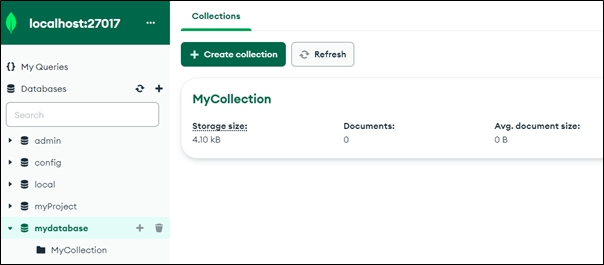
您也可以在 MongoDB shell 中验证相同的内容。
> use mydatabase
< switched to db mydatabase
> show collections
< MyCollection
< switched to db mydatabase
> show collections
< MyCollection