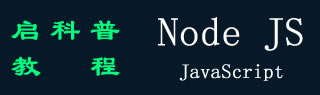
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
Node.js - MongoDB Update
Update 操作是指修改 MongoDB 集合中文档中一个或多个字段的值。Node.js 的 mongodb 驱动程序定义了 updateOne() 和 updateMany() 方法。updateOne() 方法用于修改单个文档,而 updateMany() 方法对多个文档执行更新。
updateOne() 方法的语法如下 -
collection.updateOne(query, values);
查询参数有助于查找要更新的所需文档。values 参数包含要修改文档的键值对。updateMany() 方法遵循相同的语法,不同之处在于查询从集合中返回多个文档。
updateOne()
以下示例更改 ProductID=3 的文档的价格。$set运算符为价格字段分配一个新值。
const {MongoClient} = require('mongodb');
async function main(){
const uri = "mongodb://localhost:27017/";
const client = new MongoClient(uri);
try {
await client.connect();
await sortdocs(client, "mydb", "products");
} finally {
// Close the connection to the MongoDB cluster
await client.close();
}
}
main().catch(console.error);
async function sortdocs(client, dbname, colname){
var qry = { ProductID: 3 };
var vals = { $set: { Name: "Router", price: 2750 } };
const result = await client.db(dbname).collection(colname).updateOne(qry, vals);
console.log("Document updated");
}
要验证文档是否已更新,请使用 Mongosh shell 并输入以下命令 -
> use mydb
< switched to db mydb
> db.products.find({"ProductID":3})
{
_id: ObjectId("6580964f20f979d2e9a72ae9"),
ProductID: 3,
Name: 'Router',
price: 2750
}
updateMany()
假设产品集合具有以下名称以“er”结尾的文档。
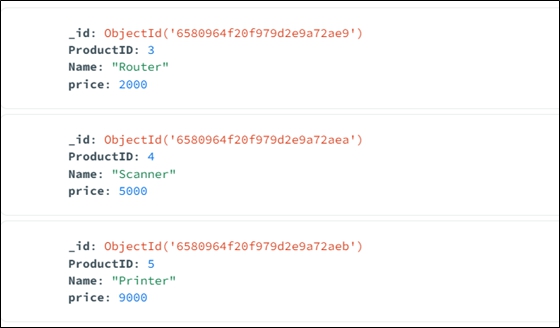
以下 sortdocs() 函数将上述所有产品的价格增加 125 卢比。我们使用了 $inc 运算符。
例
async function sortdocs(client, dbname, colname){
var qry = {Name: /er$/};
var vals = { $inc: { price: 125 } };
const result = await client.db(dbname).collection(colname).updateMany(qry, vals);
console.log("Documents updated");
}
输出