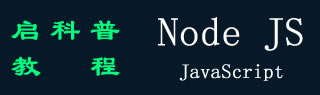
- Node.js 菜鸟教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
NodeJS - url.toJSON() 方法
URL 类的 NodeJS url.toJSON() 方法将从 URL 对象返回序列化的 URL。此方法的返回值等效于 URL.href 属性和 URL.toString() 方法。如果分配给 toJSON() 方法的值不是有效的 URL,则会抛出 TypeError。
Node.js 的 URL 模块提供了用于 URL 解析和解析的各种实用程序,toJSON() 方法就是其中之一。
语法
以下是 URL 类的 NodeJS toJSON() 方法的语法
URL.toJSON()
参数
此方法不接受任何参数。
返回值
此方法返回 URL 对象的序列化 URL。
例如果我们尝试为它分配一个有效的 URL,则 NodeJS url.toJSON() 方法将序列化的 URL 返回为字符串。
在下面的示例中,我们尝试从 URL 对象获取序列化的 URL。
const url = require('node:url');
const myURL = new URL("https://www.qikepu.com/index.htm");
console.log("The serialized URL: " + myURL.toJSON());
输出
正如我们在下面的输出中看到的,NodeJS url.toJSON() 方法返回一个序列化的 URL 字符串。
例
如果我们为 href 属性和 toJSON() 方法分配一个有效的 URL,则两者的返回值是等效的。
在以下示例中,我们将一个有效的 URL 分配给 href 属性和 toJSON 方法,并检查两者是否等效。
const url = require('node:url');
const myURL = new URL("https://www.qikepu.com/index.htm");
var toJSON = myURL.toJSON();
console.log("Result of toJSON property: " + toJSON);
var href = myURL.href;
console.log("Result of href property: " + href);
if(toJSON === href){
console.log("Both toJSON and href results are same...");
} else {
console.log("Both are not same...");
}
输出
正如我们在下面的输出中看到的,返回的值是相同的。
Result of toJSON property: https://www.qikepu.com/index.htm
Result of href property: https://www.qikepu.com/index.htm
Both toJSON and href results are same...
例
如果我们分配的值不是有效的 URL 类型,那么 toJSON() 方法将抛出 TypeError。
在以下示例中,我们将一个无效的 URL 分配给 toJSON() 方法。
const url = require('node:url');
const myURL = new URL("696wsfsps://www.qikepu4523spoint.cgfom/ind1234ex.htm");
var result = myURL.toJSON();
console.log("The serialized URL: " + result);
类型错误
如果我们编译并运行上述程序,toJSON() 方法会抛出 TypeError,因为分配的 URL 无效。
throw new ERR_INVALID_URL(input);
^
TypeError [ERR_INVALID_URL]: Invalid URL
at new NodeError (node:internal/errors:387:5)
at URL.onParseError (node:internal/url:564:9)
at new URL (node:internal/url:640:5)
at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:3:15)
at Module._compile (node:internal/modules/cjs/loader:1126:14)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10)
at Module.load (node:internal/modules/cjs/loader:1004:32)
at Function.Module._load (node:internal/modules/cjs/loader:839:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12)
at node:internal/main/run_main_module:17:47 {
input: '696wsfsps://www.qikepu4523spoint.cgfom/ind1234ex.htm',
code: 'ERR_INVALID_URL'
}