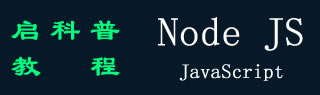
- Node.js教程
- Node.js - 教程
- Node.js - 简介
- Node.js - 环境设置
- Node.js - 首次申请
- Node.js - REPL 终端
- Node.js - 命令行选项
- Node.js - 包管理器 (NPM)
- Node.js - 回调概念
- Node.js - 上传文件
- Node.js - 发送电子邮件
- Node.js - 活动
- Node.js - 事件循环
- Node.js - 事件发射器
- Node.js - 调试器
- Node.js - 全局对象
- Node.js - 控制台
- Node.js - 流程
- Node.js - 扩展应用程序
- Node.js - 包装
- Node.js - Express 框架
- Node.js - RESTful API
- Node.js - 缓冲器
- Node.js - Streams
- Node.js - 文件系统
- Node.js MySQL
- Node.js - MySQL 快速入门
- Node.js - MySQL创建数据库
- Node.js - MySQL创建表
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where 子句
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB 快速入门
- Node.js - MongoDB 创建数据库
- Node.js - MongoDB 创建集合
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB 查询
- Node.js - MongoDB 排序
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js模块
- Node.js - 模块
- Node.js - 内置模块
- Node.js - utility 模块
- Node.js - Web 模块
NodeJS - urlSearchParams.get() 方法
URLSearchParams 类的 NodeJS urlSearchParams.get() 方法从查询字符串中的指定名称中检索值。
可以使用 URLSearchParams API 读取和写入 URL 的查询部分。此类在全局对象上也可用。
为了更好地理解这种方法,让我们看一个现实生活中的例子。请考虑此 URL ('https://www.youtube.com/watch?v=towpH780PT0'),其中 'v=towpH780PT0' 称为查询段。在此查询中,(v) 是名称,(towpH780PT0) 是值。它共同构成了一个名称-值对。
语法
以下是 NodeJS URLSearchParams.get() 方法的语法
URLSearchParams.get(name)
参数
name
:这指定了要返回的参数的名称。
返回值
此方法返回作为参数传递的给定名称的字符串值。
- 如果有多个具有给定名称的名称/值对,则此方法返回第一对中的值。
- 如果没有名称为 name 的对,则返回 null。
在以下示例中,我们尝试在查询字符串中获取名为“two”的键的值。
const url = require('node:url');
const MyUrl = new URL('https://www.qikepu.com?one=1&two=2&three=3');
console.log("URL: ", MyUrl.href);
const Params = new URLSearchParams('one=1&two=2&three=3');
console.log("Query string: " + Params);
console.log("Trying to get the value of key 'two'.....");
console.log("The value is: " + Params.get("two"));
输出
执行上述程序后,NodeJS get() 方法返回键“two”的值。
URL: https://www.qikepu.com/?one=1&two=2&three=3
Query string: one=1&two=2&three=3
Trying to get the value of key 'two'.....
The value is: 2
Query string: one=1&two=2&three=3
Trying to get the value of key 'two'.....
The value is: 2
例
如果要搜索的名称在查询字符串中多次出现,则 get() 方法将返回其第一次出现的值。
在下面的程序中,我们试图在查询字符串中获取名称“two”的值。
const url = require('node:url');
const MyUrl = new URL('https://www.qikepu.com?two=1&two=2&two=3');
console.log("URL: ", MyUrl.href);
const Params = new URLSearchParams('two=1&two=2&two=3');
console.log("Query string: " + Params);
console.log("Trying to get the value of the first key 'two'.....");
console.log("The value is: " + Params.get("two"));
输出
正如我们在输出中看到的,get() 方法返回第一次出现的值。
URL: https://www.qikepu.com/?two=1&two=2&two=3
Query string: two=1&two=2&two=3
Trying to get the value of the first key 'two'.....
The value is: 1
Query string: two=1&two=2&two=3
Trying to get the value of the first key 'two'.....
The value is: 1
例
如果查询字符串中不存在要搜索的名称,则 get() 方法返回 null。
在下面的示例中,我们尝试获取查询字符串中不存在的名称的值。
const url = require('node:url');
const MyUrl = new URL('https://www.qikepu.com?HTML=499&NODEJS=999&CSS=699');
console.log("URL: ", MyUrl.href);
const Params = new URLSearchParams('HTML=499&NODEJS=999&CSS=699');
console.log("Query string: " + Params);
console.log("Trying to get the value of the first key 'JavaScript'.....");
console.log("The value is: " + Params.get("JavaScript"));
输出
正如我们在输出中看到的,get() 方法返回 null。
URL: https://www.qikepu.com/?HTML=499&NODEJS=999&CSS=699
Query string: HTML=499&NODEJS=999&CSS=699
Trying to get the value of the first key 'JavaScript'.....
The value is: null
Query string: HTML=499&NODEJS=999&CSS=699
Trying to get the value of the first key 'JavaScript'.....
The value is: null